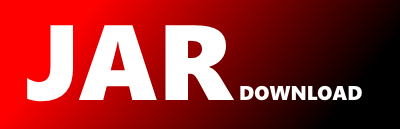
com.teamscale.index.metadata.abap.ReleaseContract Maven / Gradle / Ivy
/*
* Copyright (c) CQSE GmbH
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.teamscale.index.metadata.abap;
import java.io.Serializable;
import org.conqat.lib.commons.test.IndexValueClass;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonProperty;
/**
* A release contract is the classification of an ABAP repository object that is a prerequisite for
* its usability as a released API. It ensures a certain stability regarding consistency and
* compatibility of released ABAP APIs.
*/
@IndexValueClass
public class ReleaseContract implements Serializable {
private static final long serialVersionUID = 1L;
/**
* Sub-object name property. This id must not be changed, it is used in the .metadata files stored
* in the Abap git.
*/
public static final String SUB_OBJECT_NAME_PROPERTY = "subObjectName";
/**
* Sub-object type property. This id must not be changed, it is used in the .metadata files stored
* in the Abap git.
*/
public static final String SUB_OBJECT_TYPE_PROPERTY = "subObjectType";
/**
* Compatibility contract property. This id must not be changed, it is used in the .metadata files
* stored in the Abap git.
*/
public static final String COMPATIBILITY_CONTRACT_PROPERTY = "compatibilityContract";
/**
* Release state property. This id must not be changed, it is used in the .metadata files stored in
* the Abap git.
*/
public static final String RELEASE_STATE_PROPERTY = "releaseState";
/**
* "Use in key user apps" property. This id must not be changed, it is used in the .metadata files
* stored in the Abap git.
*/
public static final String USE_IN_KEY_USER_APPS_PROPERTY = "useInKeyUserApps";
/**
* "Use in SAP Cloud Platform" property. This id must not be changed, it is used in the .metadata
* files stored in the Abap git.
*/
public static final String USE_IN_SAP_CLOUD_PLATFORM_PROPERTY = "useInSapCloudPlatform";
/**
* Stores the release contract sub-object name of some ABAP object.
*/
@JsonProperty(SUB_OBJECT_NAME_PROPERTY)
private String subObjectName;
/**
* Stores the release contract sub-object type of some ABAP object.
*/
@JsonProperty(SUB_OBJECT_TYPE_PROPERTY)
private String subObjectType;
/**
* Stores the concrete value of the compatibility contract.
*/
@JsonProperty(COMPATIBILITY_CONTRACT_PROPERTY)
private ECompatibilityContract compatibilityContract;
/**
* Stores whether some abap object is release, deprecated, e.t.c.
*/
@JsonProperty(RELEASE_STATE_PROPERTY)
private EReleaseState releaseState;
/**
* Stores whether some ABAP object can be used in key user apps.
*/
@JsonProperty(USE_IN_KEY_USER_APPS_PROPERTY)
private boolean useInKeyUserApps;
/**
* Stores whether some ABAP object can be used in SAP cloud platform.
*/
@JsonProperty(USE_IN_SAP_CLOUD_PLATFORM_PROPERTY)
private boolean useInSapCloudPlatform;
@JsonCreator
public ReleaseContract(@JsonProperty(SUB_OBJECT_NAME_PROPERTY) String subObjectName,
@JsonProperty(SUB_OBJECT_TYPE_PROPERTY) String subObjectType,
@JsonProperty(COMPATIBILITY_CONTRACT_PROPERTY) ECompatibilityContract compatibilityContract,
@JsonProperty(RELEASE_STATE_PROPERTY) EReleaseState releaseState,
@JsonProperty(USE_IN_KEY_USER_APPS_PROPERTY) boolean useInKeyUserApps,
@JsonProperty(USE_IN_SAP_CLOUD_PLATFORM_PROPERTY) boolean useInSapCloudPlatform) {
this.subObjectName = subObjectName;
this.subObjectType = subObjectType;
this.compatibilityContract = compatibilityContract;
this.releaseState = releaseState;
this.useInKeyUserApps = useInKeyUserApps;
this.useInSapCloudPlatform = useInSapCloudPlatform;
}
/**
* @return Helper method to return empty instance of a release contract.
*/
public static ReleaseContract getEmptyContract() {
return new ReleaseContract(null, null, null, null, false, false);
}
/** @see #subObjectName */
public void setSubObjectName(String subObjectName) {
this.subObjectName = subObjectName;
}
/** @see #subObjectType */
public void setSubObjectType(String subObjectType) {
this.subObjectType = subObjectType;
}
/** @see #compatibilityContract */
public void setCompatibilityContract(ECompatibilityContract compatibilityContract) {
this.compatibilityContract = compatibilityContract;
}
/** @see #releaseState */
public void setReleaseState(EReleaseState releaseState) {
this.releaseState = releaseState;
}
/** @see #useInKeyUserApps */
public void setUseInKeyUserApps(boolean useInKeyUserApps) {
this.useInKeyUserApps = useInKeyUserApps;
}
/** @see #useInSapCloudPlatform */
public void setUseInSapCloudPlatform(boolean useInSapCloudPlatform) {
this.useInSapCloudPlatform = useInSapCloudPlatform;
}
/** @see #subObjectName */
public String getSubObjectName() {
return subObjectName;
}
/** @see #subObjectType */
public String getSubObjectType() {
return subObjectType;
}
/** @see #compatibilityContract */
public ECompatibilityContract getCompatibilityContract() {
return compatibilityContract;
}
/** @see #releaseState */
public EReleaseState getReleaseState() {
return releaseState;
}
/** @see #useInKeyUserApps */
public boolean isUseInKeyUserApps() {
return useInKeyUserApps;
}
/** @see #useInSapCloudPlatform */
public boolean isUseInSapCloudPlatform() {
return useInSapCloudPlatform;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy