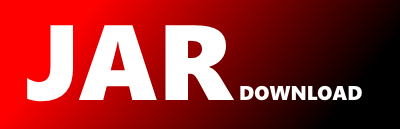
com.teamscale.index.testimpact.MethodId Maven / Gradle / Ivy
Show all versions of teamscale-commons Show documentation
package com.teamscale.index.testimpact;
import java.io.Serializable;
import java.util.Comparator;
import org.conqat.lib.commons.test.IndexValueClass;
import com.teamscale.commons.lang.ToStringHelpers;
/**
* Represents a single method at a given commit. A method ID is a tuple of uniform path and long
* value (id). The id is unique with each uniform path at a given point in time. Methods that are
* considered to have a parent<->child relationship normally keep the method ID of its predecessor
* with the exception of merges and methods being moved between uniform paths.
*
* The MethodId is an abstraction above {@link com.teamscale.index.testgap.MethodLocation} which
* tends to change quite frequently. As the TIA related indices rely on method information quite
* heavily for data persistence we use a MethodId as an additional level of indirection to keep them
* as stable as possible. The mapping between them is kept in {@link MethodIdIndex}.
*/
@IndexValueClass
public class MethodId implements Comparable, Serializable {
private static final long serialVersionUID = 1L;
/** The uniform path in which the method is contained in. */
private final String uniformPath;
/** The numeric ID of the method. */
private final int id;
public MethodId(String uniformPath, int id) {
this.uniformPath = uniformPath;
this.id = id;
}
/** @see #uniformPath */
public String getUniformPath() {
return uniformPath;
}
/** @see #id */
public int getId() {
return id;
}
@Override
public int compareTo(MethodId o) {
return Comparator.comparing(MethodId::getUniformPath).thenComparingInt(MethodId::getId).compare(this, o);
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
MethodId methodId = (MethodId) o;
return id == methodId.id && uniformPath.equals(methodId.uniformPath);
}
@Override
public int hashCode() {
// We don't use Objects.hash here as it creates an Object[] internally to
// compute the hash
return 31 * Integer.hashCode(id) + uniformPath.hashCode();
}
@Override
public String toString() {
return ToStringHelpers.toReflectiveStringHelper(this).toString();
}
}