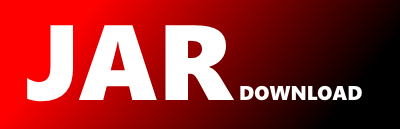
com.teamscale.service.testimpact.prioritization.IPrioritizableTests Maven / Gradle / Ivy
package com.teamscale.service.testimpact.prioritization;
import java.util.Collection;
import java.util.Comparator;
import java.util.Set;
import com.teamscale.index.testimpact.MethodId;
/**
* Interface for single tests or arbitrary sets of tests which can be prioritized.
*/
public interface IPrioritizableTests {
/** Comparator by score. */
Comparator COMPARATOR_BY_SCORE = Comparator
.comparingDouble(IPrioritizableTests::getCurrentScore);
/**
* Returns all {@link ETestSelectionReason}s for the test or test set.
*/
Set getSelectionReasons();
/**
* Returns the expected duration of executing the test or test set.
*/
long getDurationInMs(long durationValueIfNotSet);
/**
* Sets current score of the test or test set.
*/
void setCurrentScore(double currentScore);
/**
* Sets the rank of the test or test set, which is basically the 1-based index of the test in the
* prioritized list.
*/
void setRank(int rank);
/**
* Returns current score of the test or test set.
*/
double getCurrentScore();
/**
* Returns the {@link MethodId}s of the covered methods by the test or set of tests this includes
* all methods even those that have not been changed since the baseline as that is considered as
* well by the
* {@link com.teamscale.service.testimpact.prioritization.strategies.ETestPrioritizationStrategy#ADDITIONAL_COVERAGE_PER_TIME}
* strategy.
*/
Set getCoveredMethods();
/**
* Initializes the additionally covered methods by building the intersection of the covered and the
* methods that should be tested.
*/
void initAdditionallyCoveredMethods(Set methodsToTest);
/** Resets all additionally covered methods to not yet covered. */
void resetCurrentAdditionallyCoveredMethods();
/**
* Retains only the methods to keep in the additionally covered methods of the test or test set. For
* performance reasons we only count the methods so the caller needs to make sure that during one
* round a method is passed only ever once to this method when a test that covers.
*
* @see com.teamscale.service.testimpact.prioritization.strategies.CoverageRoundBasedTestPrioritizationStrategyBase#methodsCoveredInRound
*/
void removeFromCurrentAdditionallyCoveredMethods(Collection methodsToRemove);
/**
* Returns the number of unique methods covered by the test or set of tests.
*/
long getNumberOfCoveredMethods();
/**
* Returns the number of unique methods which are currently additionally covered by the test or set
* of tests.
*/
long getNumberOfCurrentAdditionallyCoveredMethods();
/**
* Returns the unique methods which are covered by the test or set of tests and have been changed
* since the baseline.
*/
Set getCoveredChangedMethods();
/**
* Returns the methods that have been changed and therefore caused the test to be impacted. This is
* only non-empty for tests impacted by code changes. Tests that are suggested because they failed,
* or ran through deleted code etc. return an empty set.
*/
Set getChangedMethodLocations();
/**
* Increases the number of additionally covered methods by 1.
*/
void incrementNumberOfAdditionallyCoveredMethods();
/**
* @return an identifier of the given prioritizable test unit. Used as sorting criterion in case
* metrics like duration and coverage are equal.
*/
String getIdentifier();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy