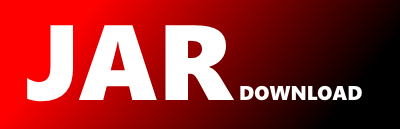
com.teamscale.service.testimpact.prioritization.PrioritizableTest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of teamscale-commons Show documentation
Show all versions of teamscale-commons Show documentation
Provides common DTOs for Teamscale
The newest version!
package com.teamscale.service.testimpact.prioritization;
import java.util.Collections;
import java.util.Objects;
import java.util.Set;
import org.conqat.engine.sourcecode.coverage.TestUniformPathUtils;
import org.conqat.lib.commons.uniformpath.UniformPath;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonGetter;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.teamscale.commons.lang.ToStringHelpers;
import com.teamscale.index.testimpact.MethodId;
/**
* Test with information about their partition as well as tracking data used during prioritization
* of tests. Two instances are considered equal if the test details are equals.
*/
public class PrioritizableTest extends PrioritizableTestBase {
private static final long serialVersionUID = 0;
/**
* The uniform path of the test or execution unit.
*/
@JsonProperty("uniformPath")
private UniformPath uniformPath;
/**
* The test name/path as it was provided in the report (without -test-execution- or -execution-unit-
* prefixes). This is needed for any TIA implementations in the test runners such as the teamscale
* Gradle plugin.
*/
@JsonProperty("testName")
private String testName;
/** The reason the test has been selected. */
@JsonProperty("selectionReason")
private ETestSelectionReason selectionReason;
/** Partition of the test. */
@JsonProperty("partition")
private String partition;
@JsonProperty("coveredMethodCount")
private int numberOfCoveredMethods = 0;
@JsonCreator
public PrioritizableTest() {
}
public PrioritizableTest(UniformPath uniformPath, ETestSelectionReason selectionReason, String partition) {
this(uniformPath, selectionReason, partition, Collections.emptySet());
}
public PrioritizableTest(UniformPath uniformPath, ETestSelectionReason selectionReason, String partition,
Set changedMethodLocations) {
super(changedMethodLocations);
this.uniformPath = uniformPath;
testName = TestUniformPathUtils.convertCoverageUnitPathToTestName(uniformPath.toString());
this.selectionReason = selectionReason;
this.partition = partition;
setCoveredMethods(changedMethodLocations);
}
@JsonGetter("type")
public UniformPath.EType getUniformPathType() {
return uniformPath.getType();
}
/** Create an instance for added or modified tests. */
public static PrioritizableTest createForAddedOrModifiedTest(String partition, UniformPath uniformPath) {
return new PrioritizableTest(uniformPath, ETestSelectionReason.ADDED_OR_MODIFIED_TEST, partition);
}
/** @see #durationInMs */
public void setDurationInMs(Long durationInMs) {
this.durationInMs = durationInMs;
}
/** Returns true if the duration is available. */
public boolean isDurationSet() {
return durationInMs != null;
}
/** @see #coveredMethods */
public void setCoveredMethods(Set coveredMethods) {
this.coveredMethods = coveredMethods;
this.numberOfCoveredMethods = coveredMethods.size();
}
/** @see #partition */
public String getPartition() {
return partition;
}
@Override
public Set getSelectionReasons() {
return Collections.singleton(selectionReason);
}
@Override
public long getDurationInMs(long durationValueIfNotSet) {
if (!isDurationSet()) {
return durationValueIfNotSet;
}
return durationInMs;
}
@Override
public Set getCoveredMethods() {
if (coveredMethods == null) {
return Collections.emptySet();
}
return Collections.unmodifiableSet(coveredMethods);
}
@Override
public long getNumberOfCoveredMethods() {
return this.numberOfCoveredMethods;
}
/** Get the test or execution unit path. */
public UniformPath getUniformPath() {
return uniformPath;
}
/** Get the test or execution unit path in string representation. */
public String getUniformPathString() {
return uniformPath.toString();
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
PrioritizableTest that = (PrioritizableTest) o;
return Objects.equals(uniformPath, that.uniformPath) && Objects.equals(partition, that.partition);
}
@Override
public int hashCode() {
return Objects.hash(uniformPath, partition);
}
/**
* Used for testing only.
*/
@Override
public String toString() {
return ToStringHelpers.toReflectiveStringHelper(this).toString();
}
@Override
public String getIdentifier() {
return this.uniformPath + ";" + this.partition;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy