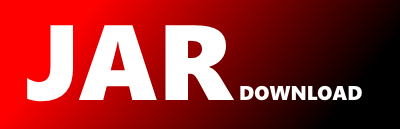
eu.cqse.check.framework.scanner.IToken Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of teamscale-commons Show documentation
Show all versions of teamscale-commons Show documentation
Provides common DTOs for Teamscale
The newest version!
/*
* Copyright (c) CQSE GmbH
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package eu.cqse.check.framework.scanner;
import java.io.Serializable;
import org.conqat.lib.commons.test.IndexValueClass;
/**
* Object of type {@link IToken} are returned by the scanners. {@link IToken}s are immutable.
*/
@IndexValueClass(containedInBackup = true)
public interface IToken extends Serializable {
/**
* Obtain the original input text for a token (copied verbatim from the source).
*/
String getText();
/**
* @param extractStringContent
* whether the content of a {@link ETokenType#STRING_LITERAL} should be returned (without
* opening/closing characters)
* @return A normalized text representation of this token
*/
default String getNormalizedText(boolean extractStringContent) {
String text = getText();
if (extractStringContent && getType() == ETokenType.STRING_LITERAL) {
return text.substring(1, text.length() - 1);
}
if (getLanguage().isCaseSensitive()) {
return text;
}
return text.toLowerCase();
}
/**
* Get the number of characters before this token in the text. The offset is 0-based and inclusive.
*/
int getOffset();
/**
* Get the number of characters before the end of this token in the text (i.e. the 0-based index of
* the last character, inclusive).
*/
int getEndOffset();
/**
* Obtain number of line this token was found at. Counting starts at 0. For tokens spanning multiple
* lines, this is the number of the line in which the first character of the token is.
*/
int getLineNumber();
/**
* Get string that identifies the origin of this token. This can, e.g., be a uniform path to the
* resource. Its actual content depends on how the token gets constructed.
*/
String getOriginId();
/**
* Obtain type of token.
*/
ETokenType getType();
/**
* Obtain language.
*/
ELanguage getLanguage();
/**
* Create new token. Can be used to clone or create modified copies of tokens.
*
* @param type
* Token type of new token
* @param offset
* Offset of new token
* @param lineNumber
* LineNumber of new token
* @param text
* Text of new token
* @param originId
* Origin id of new token
* @return New token with set values of the same java type as the token on which the method was
* called.
*/
IToken newToken(ETokenType type, int offset, int lineNumber, String text, String originId);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy