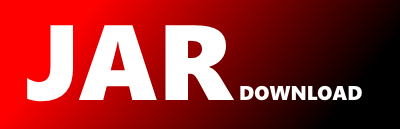
eu.cqse.check.framework.scanner.ITokenMatcher Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of teamscale-commons Show documentation
Show all versions of teamscale-commons Show documentation
Provides common DTOs for Teamscale
The newest version!
/*
* Copyright (c) CQSE GmbH
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package eu.cqse.check.framework.scanner;
import java.util.Arrays;
import java.util.Collection;
import java.util.stream.Stream;
import org.conqat.lib.commons.test.NoIndexValueClass;
/**
* Matcher for {@link IToken}.
*/
@NoIndexValueClass(rationale = "ETokenType and ETokenClass are in backup, but not any other ITokenMatcher implementation")
public interface ITokenMatcher {
/**
* @return Whether the {@code token} is matched by this matcher.
*/
boolean matches(IToken token);
/** @return A human-readable representation of this matcher. */
String humanReadable();
/**
* @return A {@link ITokenMatcher} matching on {@code this} or any {@code further}.
*/
default ITokenMatcher or(ITokenMatcher... further) {
return or(Stream.concat(Stream.of(this), Arrays.stream(further)).toList());
}
/**
* @return A {@link ITokenMatcher} matching on {@code this} and all {@code further}.
*/
default ITokenMatcher and(ITokenMatcher... further) {
return and(Stream.concat(Stream.of(this), Arrays.stream(further)).toList());
}
/**
* @return A {@link ITokenMatcher} matching not {@code this}.
*/
default ITokenMatcher negated() {
return new NegatedTokenMatcher(this);
}
/**
* @return A {@link ITokenMatcher} matching any of the {@code matchers}.
* @throws IllegalArgumentException
* Thrown if {@code matchers} is empty.
*/
static ITokenMatcher or(Collection extends ITokenMatcher> matchers) {
if (matchers.size() == 1) {
return matchers.iterator().next();
}
return new OrTokenMatcher(matchers);
}
/**
* @return A {@link ITokenMatcher} matching all of the {@code matchers}.
* @throws IllegalArgumentException
* Thrown if {@code matchers} is empty.
*/
static ITokenMatcher and(Collection extends ITokenMatcher> matchers) {
if (matchers.size() == 1) {
return matchers.iterator().next();
}
return new AndTokenMatcher(matchers);
}
/**
* @return A {@link ITokenMatcher} matching not {@code matcher}.
*/
static ITokenMatcher not(ITokenMatcher matcher) {
return matcher.negated();
}
/**
* @return A {@link ITokenMatcher} matching on any of the provided {@code tokenTypes}.
*/
static ITokenMatcher anyOfType(ETokenType... tokenTypes) {
return anyOfType(Arrays.asList(tokenTypes));
}
/**
* @return A {@link ITokenMatcher} matching on any of the provided {@code tokenTypes}.
*/
static ITokenMatcher anyOfType(Collection tokenTypes) {
return new TokenTypeTokenMatcher(tokenTypes);
}
/**
* @return A {@link ITokenMatcher} matching on none of the provided {@code tokenTypes}.
*/
static ITokenMatcher noneOfType(ETokenType... tokenTypes) {
return noneOfType(Arrays.asList(tokenTypes));
}
/**
* @return A {@link ITokenMatcher} matching on none of the provided {@code tokenTypes}.
*/
static ITokenMatcher noneOfType(Collection tokenTypes) {
return anyOfType(tokenTypes).negated();
}
/**
* @return A {@link ITokenMatcher} matching on any of the provided {@code tokenClasses}.
*/
static ITokenMatcher anyOfClass(ETokenType.ETokenClass... tokenClasses) {
return anyOfClass(Arrays.asList(tokenClasses));
}
/**
* @return A {@link ITokenMatcher} matching on any of the provided {@code tokenClasses}.
*/
static ITokenMatcher anyOfClass(Collection tokenClasses) {
return new TokenClassTokenMatcher(tokenClasses);
}
/**
* @return A {@link ITokenMatcher} matching on none of the provided {@code tokenClasses}.
*/
static ITokenMatcher noneOfClass(ETokenType.ETokenClass... tokenClasses) {
return noneOfClass(Arrays.asList(tokenClasses));
}
/**
* @return A {@link ITokenMatcher} matching on none of the provided {@code tokenClasses}.
*/
static ITokenMatcher noneOfClass(Collection tokenClasses) {
return anyOfClass(tokenClasses).negated();
}
/**
* @return A {@link ITokenMatcher} matching tokens, which have any of the provided {@code texts} as
* {@link IToken#getText() text}.
*/
static ITokenMatcher hasText(String... texts) {
return hasText(Arrays.asList(texts));
}
/**
* @return A {@link ITokenMatcher} matching tokens, which have any of the provided {@code texts} as
* {@link IToken#getText() text}.
*/
static ITokenMatcher hasText(Collection texts) {
return new TokenTextTokenMatcher(texts);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy