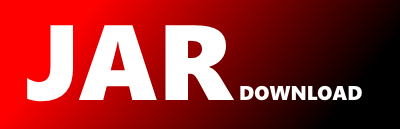
org.conqat.engine.commons.findings.location.ElementLocation Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of teamscale-commons Show documentation
Show all versions of teamscale-commons Show documentation
Provides common DTOs for Teamscale
The newest version!
/*
* Copyright (c) CQSE GmbH
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.conqat.engine.commons.findings.location;
import java.io.Serial;
import java.io.Serializable;
import java.util.Objects;
import org.checkerframework.checker.nullness.qual.Nullable;
import org.conqat.lib.commons.test.IndexValueClass;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonSubTypes;
import com.fasterxml.jackson.annotation.JsonTypeInfo;
/**
* Base class for locations. Locations are immutable and thus return this at deep cloning.
*/
@JsonTypeInfo(use = JsonTypeInfo.Id.NAME, property = "type", defaultImpl = ElementLocation.class)
@JsonSubTypes({ @JsonSubTypes.Type(value = TextRegionLocation.class, name = "TextRegionLocation"),
@JsonSubTypes.Type(value = QualifiedNameLocation.class, name = "QualifiedNameLocation"),
@JsonSubTypes.Type(value = TeamscaleIssueLocation.class, name = "TeamscaleIssueLocation"), })
@IndexValueClass(containedInBackup = true)
public class ElementLocation implements Serializable {
/** Version used for serialization. */
@Serial
private static final long serialVersionUID = 1;
/** The name of the JSON property name for {@link #location}. */
protected static final String LOCATION_PROPERTY = "location";
/** The name of the JSON property name for {@link #uniformPath}. */
protected static final String UNIFORM_PATH_PROPERTY = "uniformPath";
/**
* Separator between the uniform path (identifying a file) and the file-internal path to the
* location. For example, "foo.h:5-6" (TextRegionLocation) or "foo.slx:block1/block2"
* (QualifiedNameLocation on a Simulink model).
*/
public static final String INTERNAL_PATH_SEPARATOR = ":";
/**
* Only for backwards-compatibility. This is always equals to {@link #uniformPath}
*
* @deprecated Only intended for JsonCreator and for backwards compatibility. Use
* {{@link #uniformPath}} instead.
*/
@JsonProperty(LOCATION_PROPERTY)
@Nullable
@Deprecated
private final String location;
/** The uniform path (see {@link #getUniformPath()}). */
@JsonProperty(UNIFORM_PATH_PROPERTY)
private final String uniformPath;
/**
* @deprecated Only intended for JsonCreator. Use {@link #ElementLocation(String)} instead.
*/
@JsonCreator
@Deprecated
public ElementLocation(@JsonProperty(LOCATION_PROPERTY) String location,
@JsonProperty(UNIFORM_PATH_PROPERTY) String uniformPath) {
this.location = location;
this.uniformPath = uniformPath;
}
public ElementLocation(String uniformPath) {
this(uniformPath, uniformPath);
}
/**
* Returns the uniform path. This is an artificial path that uniquely defines a resource across
* machine boundaries. This should be used for persisted information.
*/
public String getUniformPath() {
return uniformPath;
}
/**
* Returns a single line description of the location that is meaningful to the user.
*/
public String toLocationString() {
return getUniformPath();
}
/**
* Returns a unique String representation for this location. As opposed to
* {@link #toLocationString()} this is not intended for showing to the user and may be more
* detailed.
*/
public String getLocationKey() {
return toLocationString();
}
/** {@inheritDoc} */
@Override
public String toString() {
return toLocationString();
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (!(o instanceof ElementLocation that)) {
return false;
}
return that.canEqual(this) && Objects.equals(location, that.location)
&& Objects.equals(uniformPath, that.uniformPath);
}
/**
* @return Whether {@code other} can be equal to this object.
* @apiNote This is called during {@link #equals(Object)} on the other object, to make sure
* that the equality is symmetric.
* @see How to Write an Equality
* Method in Java
*/
protected boolean canEqual(Object other) {
return other instanceof ElementLocation;
}
@Override
public int hashCode() {
return Objects.hash(location, uniformPath);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy