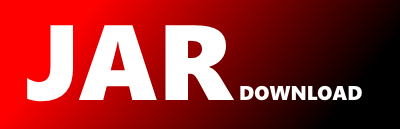
org.conqat.engine.commons.findings.location.QualifiedNameLocation Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of teamscale-commons Show documentation
Show all versions of teamscale-commons Show documentation
Provides common DTOs for Teamscale
The newest version!
/*
* Copyright (c) CQSE GmbH
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.conqat.engine.commons.findings.location;
import java.io.Serial;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import org.conqat.lib.commons.assertion.CCSMAssert;
import org.conqat.lib.commons.test.IndexValueClass;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonProperty;
/**
* Location identified by a qualified name, which usually is some kind of path expression.
*
* This is used only for finding locations in Simulink models. If you use it for another language,
* that will be a problem in findings tracking (we use this type of location to identify Simulink
* findings there).
*/
@IndexValueClass(containedInBackup = true)
public class QualifiedNameLocation extends ElementLocation {
/** Version used for serialization. */
@Serial
private static final long serialVersionUID = 1;
/** The name of the JSON property name for {@link #qualifiedName}. */
private static final String QUALIFIED_NAME_PROPERTY = "qualifiedName";
/** The name of the JSON property name for {@link #abbreviated}. */
private static final String ABBREVIATED_PROPERTY = "abbreviated";
/** The name of the JSON property name for {@link #signalNames}. */
private static final String SIGNAL_NAMES_PROPERTY = "signalNames";
/**
* The qualified name. For example, the path to a block/stateflow node in the simulink model (if
* {@link #uniformPath} points to a model) or the name of an entry in the data dictionary file (if
* {@link #uniformPath} points to a simulink data dictionary).
*/
@JsonProperty(QUALIFIED_NAME_PROPERTY)
private final String qualifiedName;
/**
* Indicates whether this is abbreviated, i.e. the qualified name is not expanded to full depth
* (e.g. because the reference can not be fully resolved).
*/
@JsonProperty(ABBREVIATED_PROPERTY)
private final boolean abbreviated;
/**
* List of signal full names that are going into the location. This is used in the Simulink
* architecture UI.
*/
@JsonProperty(SIGNAL_NAMES_PROPERTY)
private final List signalNames;
public QualifiedNameLocation(String qualifiedName, String uniformPath) {
this(qualifiedName, uniformPath, false);
}
public QualifiedNameLocation(String qualifiedName, String uniformPath, boolean abbreviated) {
this(qualifiedName, uniformPath, uniformPath, abbreviated, Collections.emptyList());
}
public QualifiedNameLocation(String qualifiedName, String uniformPath, boolean abbreviated,
List signalNames) {
this(qualifiedName, uniformPath, uniformPath, abbreviated, signalNames);
}
/**
* @deprecated Only intended for JsonCreator. Use {@link #QualifiedNameLocation(String, String)}
* instead.
*/
@JsonCreator
@Deprecated
public QualifiedNameLocation(@JsonProperty(QUALIFIED_NAME_PROPERTY) String qualifiedName,
@JsonProperty(LOCATION_PROPERTY) String location, @JsonProperty(UNIFORM_PATH_PROPERTY) String uniformPath,
@JsonProperty(ABBREVIATED_PROPERTY) boolean abbreviated,
@JsonProperty(SIGNAL_NAMES_PROPERTY) List signalNames) {
super(uniformPath);
CCSMAssert.isNotNull(qualifiedName);
this.qualifiedName = qualifiedName;
this.abbreviated = abbreviated;
this.signalNames = new ArrayList<>(signalNames);
}
/** Returns the qualified name. */
public String getQualifiedName() {
return qualifiedName;
}
@Override
public String toLocationString() {
return super.toLocationString() + INTERNAL_PATH_SEPARATOR + qualifiedName;
}
/** @see #abbreviated */
public boolean isAbbreviated() {
return abbreviated;
}
/** @see #signalNames */
public List getSignalNames() {
return signalNames;
}
@Override
protected boolean canEqual(Object other) {
return other instanceof QualifiedNameLocation;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (!(o instanceof QualifiedNameLocation that)) {
return false;
}
return that.canEqual(this) && Objects.equals(qualifiedName, that.qualifiedName)
&& abbreviated == that.abbreviated && Objects.equals(signalNames, that.signalNames) && super.equals(o);
}
@Override
public int hashCode() {
return Objects.hash(super.hashCode(), qualifiedName, abbreviated, signalNames);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy