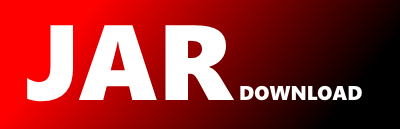
org.conqat.engine.commons.findings.location.TeamscaleIssueFieldLocation Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of teamscale-commons Show documentation
Show all versions of teamscale-commons Show documentation
Provides common DTOs for Teamscale
The newest version!
package org.conqat.engine.commons.findings.location;
import java.io.Serial;
import java.util.Objects;
import org.conqat.lib.commons.test.IndexValueClass;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonSubTypes;
import com.fasterxml.jackson.annotation.JsonTypeInfo;
/**
* This class describes a finding location in an issue or spec item or similar. That is a text
* region location in any of its fields, e.g. subject or description.
*/
@JsonTypeInfo(use = JsonTypeInfo.Id.NAME, property = "type", defaultImpl = TeamscaleIssueFieldLocation.class)
@JsonSubTypes(@JsonSubTypes.Type(value = ManualTestCaseTextRegionLocation.class, name = "ManualTestCaseTextRegionLocation"))
@IndexValueClass(containedInBackup = true)
public class TeamscaleIssueFieldLocation extends TextRegionLocation implements ITeamscaleIssueFindingLocation {
@Serial
private static final long serialVersionUID = 1L;
/** Id of the TeamscaleIssue the field belongs to. */
@JsonProperty("issueId")
private final String issueId;
/** The name of the issue field where this location belongs to. */
@JsonProperty("affectedField")
private final String affectedField;
/**
* The start offset within the {@link #affectedField}
*/
@JsonProperty("fieldStartOffset")
private final int fieldStartOffset;
/**
* The end offset within the {@link #affectedField}
*/
@JsonProperty("fieldEndOffset")
private final int fieldEndOffset;
/**
* The start line within the {@link #affectedField}
*/
@JsonProperty("fieldStartLine")
private final int fieldStartLine;
/**
* The end line within the {@link #affectedField}
*/
@JsonProperty("fieldEndLine")
private final int fieldEndLine;
public TeamscaleIssueFieldLocation(TeamscaleIssueFieldLocation location) {
super(location);
issueId = location.issueId;
affectedField = location.affectedField;
fieldStartOffset = location.fieldStartOffset;
fieldEndOffset = location.fieldEndOffset;
fieldStartLine = location.fieldStartLine;
fieldEndLine = location.fieldEndLine;
}
public TeamscaleIssueFieldLocation(String uniformPath, String issueId, RawAndFieldSpecific startOffset,
RawAndFieldSpecific endOffset, RawAndFieldSpecific startLine, RawAndFieldSpecific endLine,
String affectedField) {
super(uniformPath, startOffset.rawValue, endOffset.rawValue, startLine.rawValue, endLine.rawValue);
this.issueId = issueId;
fieldStartOffset = startOffset.fieldSpecificValue;
fieldEndOffset = endOffset.fieldSpecificValue;
fieldStartLine = startLine.fieldSpecificValue;
fieldEndLine = endLine.fieldSpecificValue;
this.affectedField = affectedField;
}
@Override
public String getIssueId() {
return issueId;
}
public String getAffectedField() {
return affectedField;
}
public int getFieldStartOffset() {
return fieldStartOffset;
}
/**
* @return Combined {@link #rawStartOffset} and {@link #fieldStartOffset}
*/
public RawAndFieldSpecific getStartOffset() {
return new RawAndFieldSpecific(getRawStartOffset(), getFieldStartOffset());
}
public int getFieldEndOffset() {
return fieldEndOffset;
}
/**
* @return Combined {@link #rawEndOffset} and {@link #fieldEndOffset}
*/
public RawAndFieldSpecific getEndOffset() {
return new RawAndFieldSpecific(getRawEndOffset(), getFieldEndOffset());
}
public int getFieldStartLine() {
return fieldStartLine;
}
/**
* @return Combined {@link #rawStartLine} and {@link #fieldStartLine}
*/
public RawAndFieldSpecific getStartLine() {
return new RawAndFieldSpecific(getRawStartLine(), getFieldStartLine());
}
public int getFieldEndLine() {
return fieldEndLine;
}
/**
* @return Combined {@link #rawEndLine} and {@link #fieldEndLine}
*/
public RawAndFieldSpecific getEndLine() {
return new RawAndFieldSpecific(getRawEndLine(), getFieldEndLine());
}
@Override
public String toLocationString() {
return String.join(ElementLocation.INTERNAL_PATH_SEPARATOR, getUniformPath(), getAffectedField(),
fieldStartLine + "-" + fieldEndLine);
}
@Override
protected boolean canEqual(Object other) {
return other instanceof TeamscaleIssueFieldLocation;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (!(o instanceof TeamscaleIssueFieldLocation that)) {
return false;
}
return that.canEqual(this) && Objects.equals(issueId, that.issueId)
&& Objects.equals(affectedField, that.affectedField) && fieldStartOffset == that.fieldStartOffset
&& fieldEndOffset == that.fieldEndOffset && fieldStartLine == that.fieldStartLine
&& fieldEndLine == that.fieldEndLine && super.equals(o);
}
@Override
public int hashCode() {
return Objects.hash(super.hashCode(), issueId, affectedField, fieldStartOffset, fieldEndOffset, fieldStartLine,
fieldEndLine);
}
/**
* Wrapper holding the raw value based the textual issue representation, and the field specific
* value.
*
* The raw value is required for the generic Teamscale features, such as findings tracking.
*/
public static class RawAndFieldSpecific {
private final int rawValue;
private final int fieldSpecificValue;
public RawAndFieldSpecific(int rawValue, int fieldSpecificValue) {
this.rawValue = rawValue;
this.fieldSpecificValue = fieldSpecificValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy