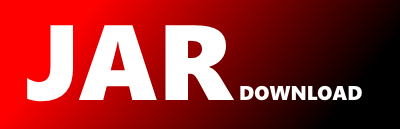
org.conqat.engine.commons.util.CollectionMapSerializationModule Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of teamscale-commons Show documentation
Show all versions of teamscale-commons Show documentation
Provides common DTOs for Teamscale
The newest version!
/*
* Copyright (c) CQSE GmbH
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.conqat.engine.commons.util;
import java.io.IOException;
import java.util.Collection;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Set;
import org.conqat.lib.commons.collections.CollectionMap;
import org.conqat.lib.commons.collections.ListMap;
import org.conqat.lib.commons.collections.SetMap;
import com.fasterxml.jackson.core.JsonGenerator;
import com.fasterxml.jackson.core.JsonParser;
import com.fasterxml.jackson.databind.BeanProperty;
import com.fasterxml.jackson.databind.DeserializationContext;
import com.fasterxml.jackson.databind.JavaType;
import com.fasterxml.jackson.databind.JsonDeserializer;
import com.fasterxml.jackson.databind.JsonSerializer;
import com.fasterxml.jackson.databind.SerializerProvider;
import com.fasterxml.jackson.databind.deser.ContextualDeserializer;
import com.fasterxml.jackson.databind.module.SimpleModule;
import com.fasterxml.jackson.databind.type.TypeFactory;
/** Module for deserialization and serialization of {@link CollectionMap}s. */
public class CollectionMapSerializationModule extends SimpleModule {
private static final long serialVersionUID = 1L;
/** Adds the serializers and deserializers to the module. */
// Since we want to allow serialization and deserialization for all types we use
// raw and unchecked types of the collections here.
@SuppressWarnings({ "unchecked", "rawtypes" })
CollectionMapSerializationModule() {
addSerializer(CollectionMap.class, new CollectionMapSerializer());
addDeserializer(ListMap.class, ListMapDeserializer.create());
addDeserializer(SetMap.class, SetMapDeserializer.create());
}
/** Generic serializer for {@link CollectionMap}s. */
private static class CollectionMapSerializer>
extends JsonSerializer> {
@Override
public void serialize(CollectionMap value, JsonGenerator generator, SerializerProvider serializers)
throws IOException {
HashMap map = new HashMap<>();
for (Map.Entry entry : value.entrySet()) {
map.put(entry.getKey(), entry.getValue());
}
generator.writeObject(map);
}
}
/** Generic deserializer base for {@link CollectionMap}s. */
private abstract static class CollectionMapDeserializerBase>
extends JsonDeserializer> {
@Override
public abstract CollectionMap deserialize(JsonParser p, DeserializationContext ctxt)
throws IOException;
/** Type of the {@link CollectionMap}'s key. */
protected JavaType keyType;
/** Type of the value that the {@link CollectionMap} stores. */
protected JavaType valueType;
private CollectionMapDeserializerBase(JavaType keyType, JavaType valueType) {
this.keyType = keyType;
this.valueType = valueType;
}
/**
* @return The {@link JavaType} of the {@link CollectionMap} that should be parsed.
*/
protected static JavaType getPropertyType(DeserializationContext ctxt, BeanProperty property) {
if (property == null) {
return ctxt.getContextualType();
}
return property.getType();
}
}
/** Deserializer for {@link ListMap} objects. */
private static class ListMapDeserializer extends CollectionMapDeserializerBase>
implements ContextualDeserializer {
private ListMapDeserializer(JavaType keyType, JavaType valueType) {
super(keyType, valueType);
}
/** Factory method for creating {@link ListMapDeserializer}s without types. */
// Since we determine the types of key and value in createContextual we use raw
// types.
@SuppressWarnings("rawtypes")
public static ListMapDeserializer create() {
return new ListMapDeserializer<>(null, null);
}
@Override
public ListMap deserialize(JsonParser parser, DeserializationContext ctx) throws IOException {
TypeFactory typeFactory = ctx.getTypeFactory();
JavaType listType = typeFactory.constructCollectionLikeType(List.class, valueType);
JavaType mapType = typeFactory.constructMapLikeType(Map.class, keyType, listType);
HashMap> root = ctx.readValue(parser, mapType);
return new ListMap<>(root);
}
@Override
public JsonDeserializer> createContextual(DeserializationContext ctxt, BeanProperty property) {
JavaType listMapType = CollectionMapDeserializerBase.getPropertyType(ctxt, property);
return new ListMapDeserializer<>(listMapType.containedType(0), listMapType.containedType(1));
}
}
/** Deserializer for {@link SetMap} objects. */
private static class SetMapDeserializer extends CollectionMapDeserializerBase>
implements ContextualDeserializer {
private SetMapDeserializer(JavaType keyType, JavaType valueType) {
super(keyType, valueType);
}
/** Factory method for creating {@link SetMapDeserializer}s without types. */
// Since we determine the types of key and value in createContextual we use raw
// types.
@SuppressWarnings("rawtypes")
public static SetMapDeserializer create() {
return new SetMapDeserializer<>(null, null);
}
@Override
public SetMap deserialize(JsonParser parser, DeserializationContext ctx) throws IOException {
TypeFactory typeFactory = ctx.getTypeFactory();
JavaType listType = typeFactory.constructCollectionLikeType(Set.class, valueType);
JavaType mapType = typeFactory.constructMapLikeType(Map.class, keyType, listType);
HashMap> root = ctx.readValue(parser, mapType);
return new SetMap<>(root);
}
@Override
public JsonDeserializer> createContextual(DeserializationContext ctxt, BeanProperty property) {
JavaType setMapType = ListMapDeserializer.getPropertyType(ctxt, property);
return new SetMapDeserializer<>(setMapType.containedType(0), setMapType.containedType(1));
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy