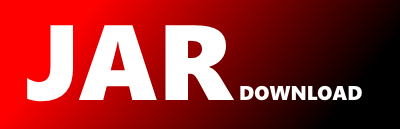
org.conqat.engine.commons.util.JavaPathModule Maven / Gradle / Ivy
Show all versions of teamscale-commons Show documentation
/*
* Copyright (c) CQSE GmbH
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.conqat.engine.commons.util;
import java.io.IOException;
import java.io.Serial;
import java.nio.file.Path;
import org.checkerframework.checker.nullness.qual.Nullable;
import com.fasterxml.jackson.core.JsonGenerator;
import com.fasterxml.jackson.core.JsonParser;
import com.fasterxml.jackson.databind.DeserializationContext;
import com.fasterxml.jackson.databind.KeyDeserializer;
import com.fasterxml.jackson.databind.SerializerProvider;
import com.fasterxml.jackson.databind.deser.std.StdDeserializer;
import com.fasterxml.jackson.databind.module.SimpleModule;
import com.fasterxml.jackson.databind.ser.std.StdSerializer;
/**
* Jackson module to support (de)serializing {@link Path}.
*/
public class JavaPathModule extends SimpleModule {
@Serial
private static final long serialVersionUID = 1L;
public JavaPathModule() {
super("JavaPathModule");
addSerializer(Path.class, new PathSerializer());
addDeserializer(Path.class, new PathDeserializer());
addKeyDeserializer(Path.class, new KeyDeserializer() {
@Override
public Object deserializeKey(String key, DeserializationContext ctxt) {
return PathDeserializer.deserialize(key);
}
});
}
/**
* Jackson serializer for {@link Path}.
*
* Works by using its {@link Path#toString()} representation. The path is used as-is, i.e., no
* {@link Path#normalize() normalization} or transforming to {@link Path#toAbsolutePath() absolute}.
*/
private static class PathSerializer extends StdSerializer {
@Serial
private static final long serialVersionUID = 1L;
public PathSerializer() {
super(Path.class);
}
@Override
public void serialize(Path value, JsonGenerator gen, SerializerProvider provider) throws IOException {
if (value == null) {
gen.writeNull();
} else {
gen.writeString(value.toString());
}
}
}
/**
* Jackson deserializer for {@link Path}.
*
* Works by using the existing factory {@link Path#of(String, String...)}.
*/
private static class PathDeserializer extends StdDeserializer {
@Serial
private static final long serialVersionUID = 1L;
public PathDeserializer() {
super(Path.class);
}
@Override
public Path deserialize(JsonParser p, DeserializationContext ctxt) throws IOException {
return deserialize(p.readValueAs(String.class));
}
/**
* @return The deserialized {@link Path}. {@code null} if {@code value} was {@code null}.
*/
public static @Nullable Path deserialize(@Nullable String value) {
if (value == null) {
return null;
}
return Path.of(value);
}
}
}