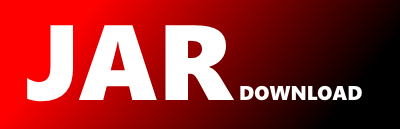
org.conqat.engine.index.baseline.BaselineInfo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of teamscale-commons Show documentation
Show all versions of teamscale-commons Show documentation
Provides common DTOs for Teamscale
The newest version!
package org.conqat.engine.index.baseline;
import java.io.Serializable;
import org.checkerframework.checker.nullness.qual.Nullable;
import org.conqat.lib.commons.test.IndexValueClass;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonProperty;
/**
* A baseline is basically a named timestamp (e.g. a release date). Objects of this class are
* immutable.
*
* This class is used as DTO during communication with IDE clients via
* {@link com.teamscale.ide.commons.client.IIdeServiceClient}, special care has to be taken when
* changing its signature!
*/
@IndexValueClass(containedInBackup = true)
public class BaselineInfo implements Serializable, Comparable {
/** Serial version UID. */
private static final long serialVersionUID = 1;
/** The name of the JSON property name for {@link #name}. */
private static final String NAME_PROPERTY = "name";
/** The name of the JSON property name for {@link #description}. */
private static final String DESCRIPTION_PROPERTY = "description";
/** The name of the JSON property name for {@link #timestamp}. */
private static final String TIMESTAMP_PROPERTY = "timestamp";
/** The name of the JSON property name for {@link #baselineRevision}. */
private static final String BASELINEREVISION_PROPERTY = "baselineRevision";
/** The name of this baseline */
@JsonProperty(NAME_PROPERTY)
private final String name;
/** The description of this baseline */
@JsonProperty(DESCRIPTION_PROPERTY)
private final String description;
/** The timestamp of this baseline */
@JsonProperty(TIMESTAMP_PROPERTY)
private final long timestamp;
/** The revision of this baseline */
@Nullable
@JsonProperty(BASELINEREVISION_PROPERTY)
private final String baselineRevision;
@JsonCreator
public BaselineInfo(@JsonProperty(NAME_PROPERTY) String name,
@JsonProperty(DESCRIPTION_PROPERTY) String description, @JsonProperty(TIMESTAMP_PROPERTY) long timestamp,
@JsonProperty(BASELINEREVISION_PROPERTY) String baselineRevision) {
this.name = name;
this.description = description;
this.timestamp = timestamp;
this.baselineRevision = baselineRevision;
}
/** Returns name. */
public String getName() {
return name;
}
/** Returns description. */
public String getDescription() {
return description;
}
/** Returns timestamp. */
public long getTimestamp() {
return timestamp;
}
/** Returns baselineRevision. */
public String getBaselineRevision() {
return baselineRevision;
}
/** {@inheritDoc} */
@Override
public int compareTo(BaselineInfo other) {
return Long.compare(timestamp, other.timestamp);
}
/** {@inheritDoc} */
@Override
public String toString() {
return name + "@" + timestamp;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy