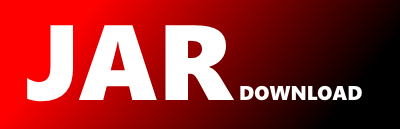
org.conqat.engine.index.shared.ArchitectureCreationModificationInfo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of teamscale-commons Show documentation
Show all versions of teamscale-commons Show documentation
Provides common DTOs for Teamscale
The newest version!
/*
* Copyright (c) CQSE GmbH
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.conqat.engine.index.shared;
import java.io.Serializable;
import java.util.Objects;
import org.checkerframework.checker.nullness.qual.Nullable;
import org.conqat.lib.commons.test.IndexValueClass;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonProperty;
/**
* Holds information about the creator and the creation date of an architecture as well as the name
* of the user who last modified the architecture and the modification date.
*/
@IndexValueClass
public class ArchitectureCreationModificationInfo implements Serializable {
private static final long serialVersionUID = 1L;
/** The name of the JSON property name for {@link #createdBy}. */
private static final String CREATED_BY_PROPERTY = "createdBy";
/** The name of the JSON property name for {@link #creationDate}. */
private static final String CREATION_DATE_PROPERTY = "creationDate";
/** The name of the JSON property name for {@link #lastModifiedBy}. */
private static final String LAST_MODIFIED_BY_PROPERTY = "lastModifiedBy";
/** The name of the JSON property name for {@link #modificationDate}. */
private static final String MODIFICATION_DATE_PROPERTY = "modificationDate";
/** The name of the creator of this architecture. */
@JsonProperty(CREATED_BY_PROPERTY)
@Nullable
private String createdBy;
/** The date the architecture was created as timestamp. */
@JsonProperty(CREATION_DATE_PROPERTY)
private Long creationDate;
/**
* The name of the user, who applied the last modification to the architecture.
*/
@JsonProperty(LAST_MODIFIED_BY_PROPERTY)
@Nullable
private String lastModifiedBy;
/** The date the last modification was made as timestamp. */
@JsonProperty(MODIFICATION_DATE_PROPERTY)
private Long modificationDate;
@JsonCreator
public ArchitectureCreationModificationInfo(@JsonProperty(CREATED_BY_PROPERTY) String createdBy,
@JsonProperty(CREATION_DATE_PROPERTY) Long creationDate,
@JsonProperty(LAST_MODIFIED_BY_PROPERTY) String lastModifiedBy,
@JsonProperty(MODIFICATION_DATE_PROPERTY) Long modificationDate) {
this.createdBy = createdBy;
this.creationDate = creationDate;
this.lastModifiedBy = lastModifiedBy;
this.modificationDate = modificationDate;
}
public ArchitectureCreationModificationInfo(Long modificationDate, String lastModifiedBy) {
this.lastModifiedBy = lastModifiedBy;
this.modificationDate = modificationDate;
}
public ArchitectureCreationModificationInfo(String createdBy, Long creationDate) {
this.createdBy = createdBy;
this.creationDate = creationDate;
}
/**
* Creates a new {@link ArchitectureCreationModificationInfo} and initializes the {@link #createdBy}
* and {@link #creationDate}.
*/
public static ArchitectureCreationModificationInfo createCreationInfo(String creator, Long creationDate) {
return new ArchitectureCreationModificationInfo(creator, creationDate);
}
/**
* Creates a new {@link ArchitectureCreationModificationInfo} and initializes the
* {@link #lastModifiedBy} and {@link #modificationDate}.
*/
public static ArchitectureCreationModificationInfo createModificationInfo(String lastModifiedBy,
Long modificationDate) {
return new ArchitectureCreationModificationInfo(modificationDate, lastModifiedBy);
}
/** @see #createdBy */
public String getCreatedBy() {
return createdBy;
}
/** @see #createdBy */
public void setCreatedBy(String creator) {
this.createdBy = creator;
}
/** @see #creationDate */
public Long getCreationDate() {
return creationDate;
}
/** @see #creationDate */
public void setCreationDate(Long creationDate) {
this.creationDate = creationDate;
}
/** @see #lastModifiedBy */
public String getLastModifiedBy() {
return lastModifiedBy;
}
/** @see #lastModifiedBy */
public void setLastModifiedBy(String lastModifiedBy) {
this.lastModifiedBy = lastModifiedBy;
}
/** @see #modificationDate */
public Long getModificationDate() {
return modificationDate;
}
/** @see #modificationDate */
public void setModificationDate(Long modificationDate) {
this.modificationDate = modificationDate;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
ArchitectureCreationModificationInfo that = (ArchitectureCreationModificationInfo) o;
return Objects.equals(createdBy, that.createdBy) && Objects.equals(creationDate, that.creationDate)
&& Objects.equals(lastModifiedBy, that.lastModifiedBy)
&& Objects.equals(modificationDate, that.modificationDate);
}
@Override
public int hashCode() {
return Objects.hash(createdBy, creationDate, lastModifiedBy, modificationDate);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy