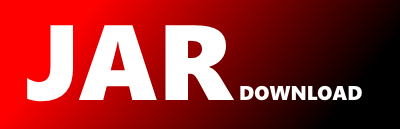
org.conqat.engine.index.shared.BasicTokenElementInfo Maven / Gradle / Ivy
Show all versions of teamscale-commons Show documentation
/*
* Copyright (c) CQSE GmbH
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.conqat.engine.index.shared;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Optional;
import org.conqat.engine.index.shared.element_details.TokenElementDetailBase;
import org.conqat.engine.resource.text.filter.base.Deletion;
import org.conqat.engine.resource.text.filter.util.StringOffsetTransformer;
import org.conqat.lib.commons.collections.CollectionUtils;
import org.conqat.lib.commons.collections.UnmodifiableList;
import org.conqat.lib.commons.js_export.ExportToTypeScript;
import org.conqat.lib.commons.js_export.NotExported;
import org.conqat.lib.commons.test.IndexValueClass;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonProperty;
import eu.cqse.check.framework.scanner.ELanguage;
/**
* Transport object with the information needed for a token element.
*
* This class is immutable.
*/
@ExportToTypeScript
@IndexValueClass
public class BasicTokenElementInfo implements Serializable {
/** The name of the JSON property name for uniformPath. */
private static final String UNIFORM_PATH_PROPERTY = "uniformPath";
/** The name of the JSON property name for language. */
private static final String LANGUAGE_PROPERTY = "language";
/** The name of the JSON property name for isLanguageSetByUser. */
private static final String IS_LANGUAGE_SET_BY_USER_PROPERTY = "isLanguageSetByUser";
/** The name of the JSON property name for text. */
private static final String TEXT_PROPERTY = "text";
/** The name of the JSON property name for filterDeletions. */
private static final String FILTER_DELETIONS_PROPERTY = "filterDeletions";
/** The name of the JSON property name for details. */
private static final String DETAILS_PROPERTY = "details";
/** Serial version UID. */
private static final long serialVersionUID = 1;
/** The uniform path. */
@JsonProperty(UNIFORM_PATH_PROPERTY)
protected final String uniformPath;
/** The language. */
@JsonProperty(LANGUAGE_PROPERTY)
protected final ELanguage language;
/**
* Whether the language was chosen based on a user defined language mapping specified in the project
* settings.
*/
@JsonProperty(IS_LANGUAGE_SET_BY_USER_PROPERTY)
@NotExported
private final boolean isFileLanguageSetByUser;
/** The text content. */
@JsonProperty(TEXT_PROPERTY)
protected final String text;
/** The list of filtered regions */
@JsonProperty(FILTER_DELETIONS_PROPERTY)
protected final ArrayList filterDeletions;
/** Additional details of this info object. */
@JsonProperty(DETAILS_PROPERTY)
protected final ArrayList details;
@JsonCreator
public BasicTokenElementInfo(@JsonProperty(UNIFORM_PATH_PROPERTY) String uniformPath,
@JsonProperty(LANGUAGE_PROPERTY) ELanguage language,
@JsonProperty(IS_LANGUAGE_SET_BY_USER_PROPERTY) boolean isFileLanguageSetByUser,
@JsonProperty(TEXT_PROPERTY) String text,
@JsonProperty(FILTER_DELETIONS_PROPERTY) Collection filterDeletions,
@JsonProperty(DETAILS_PROPERTY) Collection details) {
this.uniformPath = uniformPath;
this.language = language;
this.isFileLanguageSetByUser = isFileLanguageSetByUser;
this.text = text;
this.filterDeletions = new ArrayList<>();
if (filterDeletions != null) {
this.filterDeletions.addAll(filterDeletions);
}
Collections.sort(this.filterDeletions);
this.details = new ArrayList<>();
if (details != null) {
this.details.addAll(details);
}
}
/** Copy constructor. */
protected BasicTokenElementInfo(BasicTokenElementInfo other) {
this(other.uniformPath, other.language, other.isFileLanguageSetByUser, other.text, other.filterDeletions,
other.details);
}
public BasicTokenElementInfo(BasicTokenElementInfo other, ELanguage language) {
this(other.uniformPath, language, other.isFileLanguageSetByUser, other.text, other.filterDeletions,
other.details);
}
/** Returns the uniform path. */
public String getUniformPath() {
return uniformPath;
}
/** Returns the language. */
public ELanguage getLanguage() {
return language;
}
/** @see #isFileLanguageSetByUser */
public boolean getIsFileLanguageSetByUser() {
return isFileLanguageSetByUser;
}
/**
* Returns the text content. Note that this is the raw content, not taking into
* account content filters stored in {@link #filterDeletions}. To obtain the filtered content,
* transform this into an ITokenElement.
*/
public String getText() {
return text;
}
/**
* Returns the filtered text of this token element. Please note that the filtered content is
* computed on the fly.
*/
public String getFilteredText() {
return new StringOffsetTransformer(filterDeletions).filterString(text);
}
/** Returns the filter deletions. */
public UnmodifiableList getFilterDeletions() {
return CollectionUtils.asUnmodifiable(filterDeletions);
}
/** @see #details */
public UnmodifiableList getDetails() {
return CollectionUtils.asUnmodifiable(details);
}
/** Returns the first detail of given type (or null). */
public Optional getFirstDetailOfType(Class type) {
return getFirstDetailOfType(type, details);
}
/** Returns the first detail of given type (or null) in the given list. */
@SuppressWarnings("unchecked")
public static Optional getFirstDetailOfType(Class type,
List details) {
for (TokenElementDetailBase detail : details) {
if (type.isInstance(detail)) {
return Optional.of((T) detail);
}
}
return Optional.empty();
}
/** {@inheritDoc} */
@Override
public String toString() {
return uniformPath;
}
/** Adds the given token element detail */
public void addDetail(TokenElementDetailBase detail) {
details.add(detail);
}
}