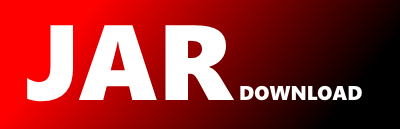
org.conqat.engine.index.shared.EGitProtocol Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of teamscale-commons Show documentation
Show all versions of teamscale-commons Show documentation
Provides common DTOs for Teamscale
The newest version!
/*
* Copyright (c) CQSE GmbH
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.conqat.engine.index.shared;
import java.net.URI;
import java.net.URISyntaxException;
import java.util.Optional;
import org.conqat.lib.commons.enums.EnumUtils;
import org.conqat.lib.commons.net.UrlUtils;
import org.conqat.lib.commons.string.StringUtils;
/**
* Enumeration of supported git transport protocols.
*/
public enum EGitProtocol {
/** File protocol. */
FILE("file", "//"),
/** HTTP protocol. */
HTTP("http", "//"),
/** HTTPS protocol. */
HTTPS("https", "//"),
/** SSH protocol */
SSH("ssh", "//git@"),
/** GIT protocol */
GIT("git", "//");
/** The scheme part of the URLs. */
private final String protocol;
/**
* The usual prefix of corresponding URLs. This is more than just the scheme in some cases (e.g.
* SSH). Used for protocol rewriting.
*/
private final String prefix;
EGitProtocol(String protocol, String protocolSuffix) {
this.protocol = protocol;
this.prefix = protocol + ":" + protocolSuffix;
}
/**
* @return the protocol from the given {@code uri}. May be empty if the uri is invalid or the
* protocol is unknown.
*/
public static Optional fromUri(String uri) {
try {
return fromUri(UrlUtils.parseUri(uri));
} catch (URISyntaxException e) {
return Optional.empty();
}
}
/**
* @return the protocol from the given {@code uri}. May be empty if the protocol is unknown.
*/
public static Optional fromUri(URI uri) {
return Optional.ofNullable(EnumUtils.valueOfIgnoreCase(EGitProtocol.class, uri.getScheme()));
}
/**
* Returns the URL protocol represented by this {@link EGitProtocol}.
*
* @return the protocol, e.g., 'git' or 'file'
*/
public String getProtocol() {
return protocol;
}
/**
* Returns the URL prefix corresponding to this protocol, e.g. file:// or http://.
*/
public String getUrlPrefix() {
return prefix;
}
/** Strips the prefix of this protocol from URL. */
public String stripUrlPrefix(String url) {
return StringUtils.stripPrefix(url, getUrlPrefix());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy