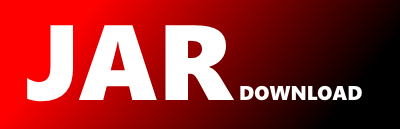
org.conqat.engine.index.shared.ExternalStorageProjectMappingId Maven / Gradle / Ivy
Show all versions of teamscale-commons Show documentation
package org.conqat.engine.index.shared;
import org.conqat.lib.commons.test.IndexValueClass;
import java.io.Serializable;
import java.util.Objects;
import java.util.UUID;
import org.conqat.lib.commons.string.StringUtils;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonValue;
import com.google.common.base.Preconditions;
/**
* This ID is currently used by the external analysis REST service to redirect uploaded data to a
* known location in an external backend which is not managed by Teamscale (Artifactory, S3, file
* system, ...). This allows us to fetch only data specifically uploaded for this project.
*
* This does not necessarily uniquely identify a project on a Teamscale instance, but is supposed to
* identify all projects that the external data is intended for (includes copies which keep all
* project data, backup imports, etc.).
*/
@IndexValueClass
public class ExternalStorageProjectMappingId implements Serializable {
private static final long serialVersionUID = 1L;
@JsonValue
private final String externalStorageProjectMappingId;
@JsonCreator
public ExternalStorageProjectMappingId(String externalStorageProjectMappingId) {
Preconditions.checkArgument(!StringUtils.isEmpty(externalStorageProjectMappingId),
"External storage project mapping ID must not be empty");
this.externalStorageProjectMappingId = externalStorageProjectMappingId;
}
/**
* Creates a new {@link ExternalStorageProjectMappingId} initialized with a random UUID.
*/
public static ExternalStorageProjectMappingId create() {
return new ExternalStorageProjectMappingId(UUID.randomUUID().toString());
}
/**
* Creates and returns a {@link ExternalStorageProjectMappingId} from the given string.
*/
public static ExternalStorageProjectMappingId of(String externalStorageProjectMappingId) {
return new ExternalStorageProjectMappingId(externalStorageProjectMappingId);
}
@Override
public String toString() {
return externalStorageProjectMappingId;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
ExternalStorageProjectMappingId that = (ExternalStorageProjectMappingId) o;
return Objects.equals(externalStorageProjectMappingId, that.externalStorageProjectMappingId);
}
@Override
public int hashCode() {
return Objects.hash(externalStorageProjectMappingId);
}
}