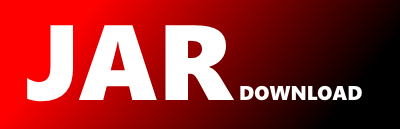
org.conqat.engine.index.shared.FindingDelta Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of teamscale-commons Show documentation
Show all versions of teamscale-commons Show documentation
Provides common DTOs for Teamscale
The newest version!
/*
* Copyright (c) CQSE GmbH
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.conqat.engine.index.shared;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.Collection;
import java.util.List;
import java.util.Set;
import org.conqat.lib.commons.collections.CollectionUtils;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonProperty;
/** Result object that wraps a list of added and removed findings */
public class FindingDelta implements Serializable {
/** Version used for serialization. */
private static final long serialVersionUID = 1;
/** The name of the JSON property name for {@link #addedFindings}. */
private static final String ADDED_FINDINGS_PROPERTY = "addedFindings";
/** The name of the JSON property name for {@link #findingsInChangedCode}. */
private static final String FINDINGS_IN_CHANGED_CODE_PROPERTY = "findingsInChangedCode";
/** The name of the JSON property name for {@link #removedFindings}. */
private static final String REMOVED_FINDINGS_PROPERTY = "removedFindings";
/** The added findings. */
@JsonProperty(ADDED_FINDINGS_PROPERTY)
private final List addedFindings;
/** The findings in changed code. */
@JsonProperty(FINDINGS_IN_CHANGED_CODE_PROPERTY)
private final List findingsInChangedCode;
/** The removed findings. */
@JsonProperty(REMOVED_FINDINGS_PROPERTY)
private final List removedFindings;
/** Creates a {@link FindingDelta}. */
public static FindingDelta create(Collection addedFindings,
Collection findingsInChangedCode, Collection removedFindings) {
return new FindingDelta(addedFindings, findingsInChangedCode, removedFindings);
}
@JsonCreator
public FindingDelta(@JsonProperty(ADDED_FINDINGS_PROPERTY) Collection addedFindings,
@JsonProperty(FINDINGS_IN_CHANGED_CODE_PROPERTY) Collection findingsInChangedCode,
@JsonProperty(REMOVED_FINDINGS_PROPERTY) Collection removedFindings) {
this.addedFindings = new ArrayList<>(addedFindings);
this.findingsInChangedCode = new ArrayList<>(findingsInChangedCode);
this.removedFindings = new ArrayList<>(removedFindings);
}
/** Returns addedFindings. */
public List getAddedFindings() {
return addedFindings;
}
/** Returns findingsInChangedCode. */
public List getFindingsInChangedCode() {
return findingsInChangedCode;
}
/** Returns removedFindings. */
public List getRemovedFindings() {
return removedFindings;
}
/** Returns numberOfAddedFindings. */
public int getNumberOfAddedFindings() {
return addedFindings.size();
}
/** Returns numberOfFindingsInChangedCode. */
public int getNumberOfFindingsInChangedCode() {
return findingsInChangedCode.size();
}
/** Returns numberOfRemovedFindings. */
public int getNumberOfRemovedFindings() {
return removedFindings.size();
}
/** Returns all findings that are part of this delta. */
public Set getAllFindings() {
return CollectionUtils.unionSet(addedFindings, findingsInChangedCode, removedFindings);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy