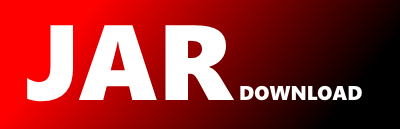
org.conqat.engine.index.shared.ParentedCommitDescriptor Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of teamscale-commons Show documentation
Show all versions of teamscale-commons Show documentation
Provides common DTOs for Teamscale
The newest version!
/*
* Copyright (c) CQSE GmbH
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.conqat.engine.index.shared;
import org.conqat.lib.commons.test.IndexValueClass;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import org.checkerframework.checker.nullness.qual.NonNull;
import org.conqat.lib.commons.assertion.CCSMAssert;
import org.conqat.lib.commons.collections.CollectionUtils;
import org.conqat.lib.commons.collections.UnmodifiableList;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonProperty;
/**
* A commit descriptor that additionally contains parent information.
*/
@IndexValueClass(containedInBackup = true)
public class ParentedCommitDescriptor extends CommitDescriptor {
/** Version for serialization. */
private static final long serialVersionUID = 1L;
/** The name of the JSON property name for {@link #parentCommits}. */
private static final String PARENT_COMMITS_PROPERTY = "parentCommits";
/**
* List of commits for which are parents of the described commit. The first commit is the direct
* parent, other commits are merge parents. This may also be empty for start commits.
*/
@JsonProperty(PARENT_COMMITS_PROPERTY)
private final List<@NonNull CommitDescriptor> parentCommits = new ArrayList<>();
public ParentedCommitDescriptor(CommitDescriptor commitDescriptor, List parentCommits) {
super(commitDescriptor.getBranchName(), commitDescriptor.getTimestamp());
insertParentCommits(parentCommits);
}
public ParentedCommitDescriptor(CommitDescriptor commitDescriptor, CommitDescriptor... parentCommits) {
this(commitDescriptor, Arrays.asList(parentCommits));
}
/** Inserts the parent commits. */
private void insertParentCommits(List parentCommits) {
for (CommitDescriptor parent : parentCommits) {
CCSMAssert.isTrue(parent.getTimestamp() < getTimestamp(),
() -> "Can't set newer commit (" + parent + ") as parent of older commit (" + this + ")");
this.parentCommits.add(new CommitDescriptor(parent.getBranchName(), parent.getTimestamp()));
}
}
/** Constructor. */
@JsonCreator
public ParentedCommitDescriptor(@JsonProperty(BRANCH_NAME_PROPERTY) String branchName,
@JsonProperty(TIMESTAMP_PROPERTY) long timestamp,
@JsonProperty(PARENT_COMMITS_PROPERTY) CommitDescriptor... parentCommits) {
super(branchName, timestamp);
insertParentCommits(Arrays.asList(parentCommits));
}
/**
* Creates a clean copy of the given {@link ParentedCommitDescriptor}. It will remove any additional
* information, if the given commit is a specialization of {@link ParentedCommitDescriptor}, such as
* RichCommitDescriptor
*/
public static ParentedCommitDescriptor cleanCopyOf(ParentedCommitDescriptor parentedCommitDescriptor) {
return new ParentedCommitDescriptor(
new CommitDescriptor(parentedCommitDescriptor.getBranchName(), parentedCommitDescriptor.getTimestamp()),
parentedCommitDescriptor.getParentCommits());
}
/** @see #parentCommits */
public UnmodifiableList getParentCommits() {
return CollectionUtils.asUnmodifiable(parentCommits);
}
/** Returns the first parent or null. */
public CommitDescriptor getFirstParentCommit() {
if (isStartCommit()) {
return null;
}
return parentCommits.get(0);
}
/** Returns the parent commits without the first parent commit. */
public UnmodifiableList getOtherParentCommits() {
if (isStartCommit()) {
return CollectionUtils.asUnmodifiable(Collections.emptyList());
}
return CollectionUtils.asUnmodifiable(parentCommits.subList(1, parentCommits.size()));
}
/** Returns true if this is a merge commit (i.e., has more than one parents). */
public boolean isMergeCommit() {
return parentCommits.size() > 1;
}
/** Returns true if this is a start commit (i.e., has no parents). */
public boolean isStartCommit() {
return parentCommits.isEmpty();
}
/** Returns a string representation including parents. */
public String toStringWithParents() {
StringBuilder builder = new StringBuilder();
builder.append(this);
parentCommits.forEach(parent -> builder.append(" parent:").append(parent));
return builder.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy