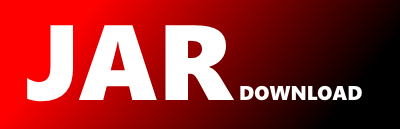
org.conqat.engine.index.shared.PreCommit3Result Maven / Gradle / Ivy
Show all versions of teamscale-commons Show documentation
/*
* Copyright (c) CQSE GmbH
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.conqat.engine.index.shared;
import java.util.List;
import java.util.Objects;
import org.checkerframework.checker.nullness.qual.Nullable;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.teamscale.commons.lang.ToStringHelpers;
/**
* Result from a pre-commit 3.0 analysis.
*/
public class PreCommit3Result {
/**
* The token to poll for final results. If this is provided, you should poll for finished results
* later on. If this is null, the returned results are final and not further analysis will happen.
*/
@JsonProperty("token")
@Nullable
private final String token;
/**
* Detailed descriptions/classifications of error that occurred (may be null if there were no
* errors).
*
* The list can contain multiple entries with the same type (e.g., multiple "file is excluded"
* errors for different paths).
*/
@JsonProperty("detailedErrors")
@Nullable
public final List detailedErrors;
/**
* The findings. This may contain data even in case of errors. Additionally, this may be null to
* indicate that the findings information is not valid (i.e. during intermediate polling).
*/
@JsonProperty("findings")
@Nullable
public final List findings;
@JsonCreator
public PreCommit3Result(@JsonProperty("token") @Nullable String token,
@JsonProperty("detailedErrors") @Nullable List detailedErrors,
@JsonProperty("findings") @Nullable List findings) {
this.token = token;
this.detailedErrors = detailedErrors;
this.findings = findings;
}
public @Nullable String getToken() {
return token;
}
/**
* Types of errors that can occur in the pre-commit analysis. Some error types are for the entire
* analysis (e.g., "pre-commit is disabled"), some are file-specific (e.g., "file is excluded").
*
* Even if there are errors in a {@link PreCommit3Result}, it may still contain meaningful results
* (for other files).
*/
public enum EPrecommit3ErrorType {
/**
* This server is configured to discard any pre-commit3 requests. The configuration may change of
* course.
*
* This is a global error (file independent).
*/
PRECOMMIT_COMMIT_ANALYSIS_DISABLED_ON_SERVER,
/**
* The precommit3 request was aborted because it contained too many files.
*
* This is a global error (file independent).
*/
FILE_COUNT_LIMIT,
/**
* The precommit3 request was aborted because it contained too large files.
*
* This is a file-specific error. However, at the moment, the entire pre-commit3 request will be
* rejected if one file is above the limit.
*/
FILE_SIZE_LIMIT,
/**
* The pre-commit3 request was aborted because the user sent this request too soon after the
* previous precommit3 request (load limiting).
*
* This is a global error (file independent).
*/
USER_TIME_LIMIT,
/**
* The pre-commit3 request was aborted because the Teamscale license on the server is not valid.
*
* This is a global error (file independent).
*/
TEAMSCALE_LICENSE_ERROR,
/**
* The pre-commit request was aborted because the server was polled for a pre-commit result but the
* respective pre-commit branch did not exist anymore.
*/
PRECOMMIT_BRANCH_NOT_AVAILABLE_DURING_POLL,
/**
* The reported file was not analyzed because it was excluded by the project configuration.
*
* This is a file-specific error.However, at the moment, the entire pre-commit3 request will be
* rejected if one file is excluded.
*/
FILE_EXCLUDED_BY_PROJECT_CONFIGURATION,
/**
* The pre-commit3 request was aborted because the project has no connector which accepts
* pre-commits.
*/
NO_VALID_CONNECTOR,
/**
* The branch analysis is not on head or close enough.
*
* This is a file independent error.
*/
ANALYSIS_NOT_LIVE,
/**
* The branch used in the local repository is not known in Teamscale.
*
* This is a file independent error.
*/
BRANCH_UNKNOWN
}
/**
* Detailed information on one error that occurred during pre-commit analysis. Depending on the
* information in this object clients (IDE plugins) may decide to display the error more or less
* visible or hide it completely.
*/
public static final class PreCommit3ErrorDetail {
/**
* The type of this error.
*
* Should be {@link EPrecommit3ErrorType#toString()} of one of {@link EPrecommit3ErrorType}, but if
* this object was loaded in a plugin and comes from a server that is newer than the plugin, the
* {@link EPrecommit3ErrorType} item might not be known to the code in the plugin yet. To ensure
* that we can still deserialize the object, we store the type as string.
*/
@JsonProperty("type")
public final String type;
/**
* The message of this error. Intended for display to the user.
*/
@JsonProperty("message")
public final String message;
/**
* Uniform path that is affected by this error (or null if it is an error that affects the entire
* pre-commit analysis).
*/
@JsonProperty("affectedUniformPath")
public final @Nullable String affectedUniformPath;
/**
* If true this error causes results in this pre-commit-result object to be invalid, for example
* because the analysis was aborted.
*
* If {@link #affectedUniformPath} is non-null, this could abort only the analysis of that file.
* Other files might still be analyzed.
*
* If {@link #affectedUniformPath} is null (i.e., this is a file-independent problem such as
* "pre-commit analysis is disabled on server"), then the entire analysis request is aborted.
*/
@JsonProperty("resultsInvalid")
public final boolean resultsInvalid;
@JsonCreator
private PreCommit3ErrorDetail(@JsonProperty("type") String type, @JsonProperty("message") String message,
@JsonProperty("affectedUniformPath") @Nullable String affectedUniformPath,
@JsonProperty("resultsInvalid") boolean resultsInvalid) {
this.type = Objects.requireNonNull(type);
this.message = Objects.requireNonNull(message);
this.affectedUniformPath = affectedUniformPath;
this.resultsInvalid = resultsInvalid;
}
/**
* Returns whether this error invalidates all results.
*/
public boolean isFileIndependentAbortionError() {
return resultsInvalid && affectedUniformPath == null;
}
/**
* Creates an error detail that affects the entire pre-commit request (instead of only a single
* file).
*/
public static PreCommit3ErrorDetail createFileIndependentError(EPrecommit3ErrorType type, String message,
boolean analysisAborted) {
return new PreCommit3ErrorDetail(type.toString(), message, null, analysisAborted);
}
/**
* Creates an error detail that affects only one file of the pre-commit request.
*/
public static PreCommit3ErrorDetail createErrorForFile(EPrecommit3ErrorType type, String message,
String affectedUniformPath, boolean analysisAborted) {
return new PreCommit3ErrorDetail(type.toString(), message, affectedUniformPath, analysisAborted);
}
@Override
public boolean equals(Object other) {
if (this == other) {
return true;
}
if (other == null || getClass() != other.getClass()) {
return false;
}
PreCommit3ErrorDetail that = (PreCommit3ErrorDetail) other;
return resultsInvalid == that.resultsInvalid && Objects.equals(type, that.type)
&& Objects.equals(message, that.message)
&& Objects.equals(affectedUniformPath, that.affectedUniformPath);
}
@Override
public int hashCode() {
return Objects.hash(type, message, affectedUniformPath, resultsInvalid);
}
@Override
public String toString() {
return ToStringHelpers.toReflectiveStringHelper(this).toString();
}
}
}