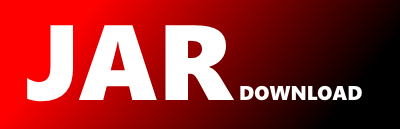
org.conqat.engine.index.shared.ProjectIdBase Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of teamscale-commons Show documentation
Show all versions of teamscale-commons Show documentation
Provides common DTOs for Teamscale
The newest version!
package org.conqat.engine.index.shared;
import java.io.Serializable;
import java.util.Objects;
import java.util.regex.Pattern;
import org.conqat.lib.commons.string.StringUtils;
import org.conqat.lib.commons.test.IndexValueClass;
import com.fasterxml.jackson.annotation.JsonValue;
import com.google.common.base.Preconditions;
/** Abstract base class for project IDs. */
@IndexValueClass(containedInBackup = true)
public abstract class ProjectIdBase implements IProjectId, Serializable {
private static final long serialVersionUID = 1L;
/**
* Pattern for detecting UUIDs (which are used exclusively for internal IDs).
*/
private static final Pattern UUID_PATTERN = Pattern
.compile("^[0-9A-Fa-f]{8}-[0-9A-Fa-f]{4}-[0-9A-Fa-f]{4}-[0-9A-Fa-f]{4}-[0-9A-Fa-f]{12}$");
/** The project ID. */
@JsonValue
public final String projectId;
protected ProjectIdBase(String projectId) {
Preconditions.checkArgument(!StringUtils.isEmpty(projectId), "Project ID must not be empty");
this.projectId = projectId;
}
/**
* Returns whether this ID conforms to the format expected for an internal ID.
*/
protected boolean isUuidFormat() {
return isUuidIdFormat(projectId);
}
@Override
public String toString() {
return projectId;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
ProjectIdBase that = (ProjectIdBase) o;
return Objects.equals(projectId, that.projectId);
}
@Override
public int hashCode() {
return Objects.hash(projectId);
}
@Override
public int compareTo(IProjectId o) {
return toString().compareTo(o.toString());
}
/**
* Returns whether the given ID conforms to the format expected for an internal ID.
*/
private static boolean isUuidIdFormat(String id) {
return UUID_PATTERN.matcher(id).matches();
}
/**
* Converts a given string into a typed representation, based on its format. UUIDs are converted
* into internal IDs, everything else is assumed to be a public ID. If the given argument is
* null
or null
, then this returns null
.
*/
public static IProjectId convert(String projectId) {
if (StringUtils.isEmpty(projectId)) {
return null;
}
if (isUuidIdFormat(projectId)) {
return new InternalProjectId(projectId);
}
return new PublicProjectId(projectId);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy