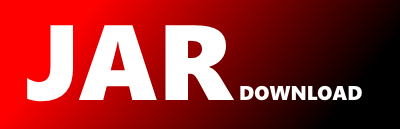
org.conqat.engine.index.shared.PublicProjectId Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of teamscale-commons Show documentation
Show all versions of teamscale-commons Show documentation
Provides common DTOs for Teamscale
The newest version!
package org.conqat.engine.index.shared;
import java.util.List;
import java.util.Set;
import java.util.regex.Pattern;
import org.conqat.lib.commons.collections.CollectionUtils;
import org.conqat.lib.commons.test.IndexValueClass;
import com.fasterxml.jackson.annotation.JsonCreator;
/**
* A public project ID (as opposed to an {@link InternalProjectId}) is a user-facing project ID. The
* assignment of a public project ID to a project is unambiguous but volatile, i.e. at any given
* point in time the ID will only refer to a single project, but it may be disassociated with the
* project and assigned to a different one whenever the user chooses.
*
* A project may have one or more public IDs. The first one of these is called "primary public ID",
* the other ones "alternative public IDs". The primary public ID is displayed by Teamscale as a
* canonical ID whenever the user interacts with the project, the alternative IDs are provided for
* backwards compatibility when a project ID changes but some old references remain (e.g., URLs
* still pointing to the former project ID).
*/
@IndexValueClass(containedInBackup = true)
public class PublicProjectId extends ProjectIdBase {
private static final long serialVersionUID = 1L;
/**
* List of characters allowed in a project id for a regular expression class.
*/
private static final String PROJECT_ID_ALLOWED_CHARACTERS = "-_.a-zA-Z0-9";
/**
* Regular expression matching all characters not allowed in a project id.
*/
public static final Pattern PROJECT_ID_FORBIDDEN_CHARACTERS_REGEX = Pattern
.compile("[^" + PROJECT_ID_ALLOWED_CHARACTERS + "]+|aliases|ids");
/**
* Expression defining valid project IDs. These are simple names that will never cause problems in a
* URL.
*/
public static final Pattern PROJECT_ID_REGEX = Pattern
.compile("^(?!aliases$)(?!ids$)[" + PROJECT_ID_ALLOWED_CHARACTERS + "]+$");
@JsonCreator
public PublicProjectId(String projectId) {
super(projectId);
if (isUuidFormat()) {
throw new IllegalArgumentException("Tried to create a public project ID from \"" + projectId
+ "\", which does not fit the expected format. Did you supply an internal project ID by mistake?");
}
}
/*** Returns whether the given ID is valid. */
public static boolean isValidId(PublicProjectId publicId) {
return PROJECT_ID_REGEX.matcher(publicId.toString()).matches();
}
@Override
public boolean isInternal() {
return false;
}
/** Creates and returns a {@link PublicProjectId} from the given string. */
public static PublicProjectId of(String projectId) {
return new PublicProjectId(projectId);
}
/**
* Creates and returns a {@link PublicProjectId} list from the given strings.
*/
public static List of(String... projectIds) {
return CollectionUtils.map(projectIds, PublicProjectId::new);
}
/**
* Creates and returns a {@link PublicProjectId} list from the given strings.
*/
public static List of(List projectIds) {
return CollectionUtils.map(projectIds, PublicProjectId::new);
}
/**
* Creates and returns a {@link PublicProjectId} set from the given strings.
*/
public static Set of(Set projectIds) {
return CollectionUtils.mapToSet(projectIds, PublicProjectId::new);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy