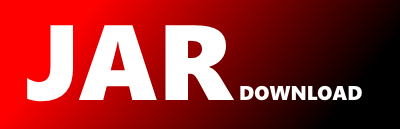
org.conqat.engine.sourcecode.coverage.CoverageInfoRetriever Maven / Gradle / Ivy
Show all versions of teamscale-commons Show documentation
package org.conqat.engine.sourcecode.coverage;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Map;
import java.util.Set;
import java.util.stream.Collectors;
import org.conqat.lib.commons.collections.CaseInsensitiveMap;
/**
* Allows retrieval of coverage infos based on qualified source names. Instances are passed as
* strategy to report handlers for line coverage tools, that are either used within a processor or
* other places of Teamscale (e.g. external analysis uploading). Depending on the usage of coverage
* info objects have to be retrieved in different ways.
*/
public class CoverageInfoRetriever {
/**
* The line coverage data as a mapping from qualified source file name to {@link LineCoverageInfo}.
* This mapping can be case sensitive or insensitive.
*/
private final Map lineCoverage;
/**
* The probe-based coverage data as a mapping from qualified source file name to
* {@link ProbeCoverageInfo}. This mapping can be case sensitive or insensitive.
*/
private final Map probeCoverage;
/**
* The testwise coverage data as a mapping from a test uniform path (with -test-execution-/ prefix,
* escaped) to {@link MultiFileRangeCoverageInfo}.
*/
private final Map testwiseCoverage;
private final Map executableUnits;
private final Map coverageUnits;
/** Constructor without report location normalization. */
public CoverageInfoRetriever() {
this(false);
}
public CoverageInfoRetriever(boolean caseSensitive) {
if (caseSensitive) {
lineCoverage = new HashMap<>();
probeCoverage = new HashMap<>();
} else {
lineCoverage = new CaseInsensitiveMap<>();
probeCoverage = new CaseInsensitiveMap<>();
}
testwiseCoverage = new HashMap<>();
executableUnits = new HashMap<>();
coverageUnits = new HashMap<>();
}
/**
* Retrieves either an already existing line coverage info for the given qualified source name or
* creates a new one and registers it in this retriever.
*/
public LineCoverageInfo getOrCreateLineCoverageInfo(String qualifiedSourceName) {
return lineCoverage.computeIfAbsent(qualifiedSourceName, k -> new LineCoverageInfo(false));
}
/**
* Retrieves either an already existing line coverage info for the given qualified source name or
* creates a new one and registers it in this retriever.
*/
public ProbeCoverageInfo getOrCreateProbeCoverageInfo(String qualifiedSourceName) {
return probeCoverage.computeIfAbsent(qualifiedSourceName, k -> new ProbeCoverageInfo(true));
}
/**
* Retrieves either an already existing line coverage info for the given qualified source name or
* creates a new one and registers it in this retriever.
*/
public TestInfoContainer createTestwiseCoverageInfo(String testExecutionPath) {
return testwiseCoverage.computeIfAbsent(testExecutionPath, TestInfoContainer::new);
}
/**
* Retrieves either an already existing execution unit for the given execution unit uniform path or
* creates a new one and registers it in this retriever.
*/
public ExecutionUnit createExecutionUnit(String executionUnitUniformPath) {
return executableUnits.computeIfAbsent(executionUnitUniformPath, ExecutionUnit::new);
}
/**
* Retrieves either an already existing coverage unit container for the given coverage unit uniform
* path or creates a new one and registers it in this retriever.
*
* Coverage units use -test-execution- paths, same as {@link TestInfoContainer}s.
*/
public CoverageUnitContainer createCoverageUnitContainer(String coverageUnitPath) {
return coverageUnits.computeIfAbsent(coverageUnitPath, k -> new CoverageUnitContainer());
}
/**
* Returns all paths that are affected by one of {@link #lineCoverage}, {@link #probeCoverage} or
* {@link #coverageUnits}.
*/
public Set getAllPaths() {
Set reportPaths = new HashSet<>();
reportPaths.addAll(lineCoverage.keySet());
reportPaths.addAll(probeCoverage.keySet());
reportPaths.addAll(coverageUnits.values().stream()
.flatMap(v -> v.getCoverageInfo().getCoverage().keySet().stream()).collect(Collectors.toSet()));
return reportPaths;
}
/**
* Returns all line coverage that has been created for each file. Important: If this
* retriever was created in case-insensitive mode, this returns an instance of
* {@link CaseInsensitiveMap} ignoring case, for which keySet() will return lower-cased keys.
*/
public Map getAllLineCoverage() {
return lineCoverage;
}
/**
* Returns all probe based coverage that has been created for each file. Important: If this
* retriever was created in case-insensitive mode, this returns an instance of
* {@link CaseInsensitiveMap} ignoring case, for which keySet() will return lower-cased keys.
*/
public Map getAllProbeCoverage() {
return probeCoverage;
}
/**
* Returns {@link TestInfoContainer} that has been created for each test.
*/
public Map getAllTestwiseCoverage() {
return testwiseCoverage;
}
public Map getAllExecutionUnits() {
return executableUnits;
}
public Map getAllCoverageUnits() {
return coverageUnits;
}
}