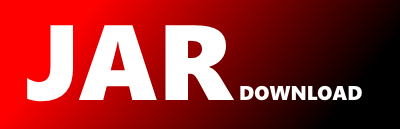
org.conqat.engine.sourcecode.coverage.TestInfoContainer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of teamscale-commons Show documentation
Show all versions of teamscale-commons Show documentation
Provides common DTOs for Teamscale
The newest version!
package org.conqat.engine.sourcecode.coverage;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import org.checkerframework.checker.nullness.qual.Nullable;
import org.conqat.engine.index.shared.tests.ETestExecutionResult;
import org.conqat.engine.index.shared.tests.TestExecution;
/**
* A container that holds {@link TestExecution} and coverage of a single test case
* ({@link MultiFileRangeCoverageInfo}).
*/
public class TestInfoContainer {
/**
* The uniform path of the test execution (-test-execution-).
*/
private final String uniformPath;
/**
* A list of ANT-patterns limiting the search space for a corresponding test implementation.
*/
@Nullable
private List testLocations;
@Nullable
private String executionUnit;
/** The content of the test (See {@link TestExecution#getHash()}). */
private @Nullable String hash;
/**
* The execution duration of the test (See {@link TestExecution#getDurationSeconds()}).
*/
private Double durationSeconds;
/** The result of the test (See {@link TestExecution#getResult()}). */
private ETestExecutionResult result;
/**
* The error message or ignore reason (See {@link TestExecution#getMessage()}).
*/
@Nullable
private String message;
/**
* A readable name for the test that must not be unique which can be shown in the UI.
*/
@Nullable
private String readableName;
/**
* A map of strings that can carry additional properties that are displayed on the Test Details
* page.
*/
@Nullable
private Map properties;
/**
* Link to an external build or test management tool that offers information about the run of the
* execution unit.
*/
@Nullable
private String externalLink;
/** A list of unparsed spec item ids that reference this test. */
@Nullable
private List associatedSpecItems;
private boolean partOfPartialReport = false;
/** Constructor. */
public TestInfoContainer(String uniformPath) {
TestUniformPathUtils.assertIsTestExecutionPath(uniformPath);
this.uniformPath = uniformPath;
}
public String getUniformPath() {
return uniformPath;
}
public void setTestLocations(@Nullable List testLocations) {
this.testLocations = testLocations;
}
public boolean isPartOfPartialReport() {
return partOfPartialReport;
}
public void setPartOfPartialReport(boolean partOfPartialReport) {
this.partOfPartialReport = partOfPartialReport;
}
/**
* Returns true if the container contains test execution information ({@link #durationSeconds} and
* {@link #result} is set).
*/
public boolean hasTestExecution() {
return result != null || durationSeconds != null;
}
/**
* Constructs a {@link TestExecution} object with the information in this container.
*/
public TestExecution getTestExecution() {
ETestExecutionResult result = Optional.ofNullable(this.result).orElse(ETestExecutionResult.PASSED);
double durationSeconds = Optional.ofNullable(this.durationSeconds).orElse(0.0);
return TestExecution.Builder.fromUniformPath(uniformPath).setDurationInSeconds(durationSeconds)
.setResult(result).setFailureMessage(message).setHash(hash).setExternalLink(externalLink)
.setExecutionUnit(executionUnit).setAssociatedSpecItems(associatedSpecItems)
.setTestLocations(testLocations).build();
}
/** @see #hash */
public void setHash(@Nullable String hash) {
this.hash = hash;
}
/** @see #durationSeconds */
public void setDurationSeconds(Double durationSeconds) {
this.durationSeconds = durationSeconds;
}
/** @see #result */
public void setResult(ETestExecutionResult result) {
this.result = result;
}
/** @see #executionUnit */
public void setExecutionUnit(@Nullable String executionUnit) {
this.executionUnit = executionUnit;
}
/** @see #message */
public void setMessage(@Nullable String message) {
this.message = message;
}
/** @see #readableName */
public void setReadableName(@Nullable String readableName) {
this.readableName = readableName;
}
/** @see #properties */
public void setProperties(@Nullable Map properties) {
this.properties = properties;
}
/** @see #externalLink */
public void setExternalLink(@Nullable String externalLink) {
this.externalLink = externalLink;
}
/** @see #associatedSpecItems */
public void setAssociatedSpecItems(@Nullable List associatedSpecItems) {
this.associatedSpecItems = associatedSpecItems;
}
/** @see #durationSeconds */
public Double getDurationSeconds() {
return durationSeconds;
}
/** @see #result */
public ETestExecutionResult getResult() {
return result;
}
/** @see #properties */
@Nullable
public Map getProperties() {
return properties;
}
/** @see #readableName */
@Nullable
public String getReadableName() {
return readableName;
}
/** @see #externalLink */
@Nullable
public String getExternalLink() {
return externalLink;
}
/** @see #associatedSpecItems */
@Nullable
public List getAssociatedSpecItems() {
return associatedSpecItems;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy