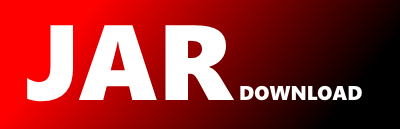
org.conqat.engine.sourcecode.coverage.dotnet.LookupBasedCoverageCreator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of teamscale-commons Show documentation
Show all versions of teamscale-commons Show documentation
Provides common DTOs for Teamscale
The newest version!
package org.conqat.engine.sourcecode.coverage.dotnet;
import java.util.HashMap;
import java.util.Map;
import java.util.Map.Entry;
import org.apache.logging.log4j.LogManager;
import org.apache.logging.log4j.Logger;
import org.conqat.engine.sourcecode.coverage.CoverageInfoRetriever;
import org.conqat.engine.sourcecode.coverage.ELineCoverage;
import org.conqat.engine.sourcecode.coverage.LineCoverageInfo;
/**
* Holds information about the mapping from file IDs to paths, and accepts coverage information for
* line ranges.
*/
public class LookupBasedCoverageCreator {
private static final Logger LOGGER = LogManager.getLogger();
/**
* Mapping from source file IDs as they occur in the coverage report to coverage information.
*/
private final Map sourceFileIdToCoverage = new HashMap<>();
/**
* Mapping from source file IDs as they occur in the coverage report to source file paths.
*/
private final Map sourceFileIdToSourceFilePath = new HashMap<>();
/** The line coverage info retriever. */
private final CoverageInfoRetriever retriever;
/** Constructor. */
public LookupBasedCoverageCreator(CoverageInfoRetriever retriever) {
this.retriever = retriever;
}
/** Stores a mapping from file ID to file path. */
public void storeFileMapping(int id, String path) {
sourceFileIdToSourceFilePath.put(id, path);
}
/**
* Adds line coverage information for the given file ID, line region and coverage type while merging
* already present coverage for the line(s). This e.g. means that a line marked as "fully covered"
* will never be reset, see {@link LineCoverageInfo#addLineCoverage}.
*
* To override coverage, use {@link #setLineCoverage(int, int, int, ELineCoverage)} instead.
*/
public void addLineCoverage(int sourceFileID, int lineStart, int lineEnd, ELineCoverage coverage) {
addLineCoverage(sourceFileID, lineStart, lineEnd, coverage, false);
}
/**
* Adds line coverage information for the given file ID, line region and coverage type while
* overriding existing coverage for the line(s).
*
* To merge coverage for a line, use {@link #addLineCoverage(int, int, int, ELineCoverage)} instead.
*/
public void setLineCoverage(int sourceFileID, int lineStart, int lineEnd, ELineCoverage coverage) {
addLineCoverage(sourceFileID, lineStart, lineEnd, coverage, true);
}
/**
* Adds the given coverage for the given file and line(s).
*
* @param removeExistingCoverageForLine
* if set to {@code true}, any existing coverage for will be removed before the current
* coverage is added. Otherwise, the coverage results will be merged.
*
*/
private void addLineCoverage(int sourceFileID, int lineStart, int lineEnd, ELineCoverage coverage,
boolean removeExistingCoverageForLine) {
if (!sourceFileIdToCoverage.containsKey(sourceFileID)) {
sourceFileIdToCoverage.put(sourceFileID, new LineCoverageInfo(false));
}
LineCoverageInfo lineCoverageInfo = sourceFileIdToCoverage.get(sourceFileID);
for (int line = lineStart; line <= lineEnd; line++) {
if (removeExistingCoverageForLine) {
lineCoverageInfo.removeLineCoverageInfo(line);
}
lineCoverageInfo.addLineCoverage(line, coverage);
}
}
/**
* Stores the coverage info in the {@link #retriever} and clears any internal coverage and mapping
* information.
*/
public void storeCoverageInfo() {
for (Entry fileIdAndCoverage : sourceFileIdToCoverage.entrySet()) {
String sourceFilePath = sourceFileIdToSourceFilePath.get(fileIdAndCoverage.getKey());
if (sourceFilePath == null) {
LOGGER.error("No source file with the ID '" + fileIdAndCoverage + "' found");
continue;
}
retriever.getOrCreateLineCoverageInfo(sourceFilePath).addAll(fileIdAndCoverage.getValue());
}
sourceFileIdToCoverage.clear();
sourceFileIdToSourceFilePath.clear();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy