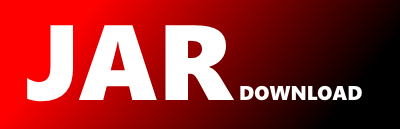
org.conqat.engine.sourcecode.coverage.testwise_coverage.ExecutionUnitInfo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of teamscale-commons Show documentation
Show all versions of teamscale-commons Show documentation
Provides common DTOs for Teamscale
The newest version!
package org.conqat.engine.sourcecode.coverage.testwise_coverage;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
import org.checkerframework.checker.nullness.qual.Nullable;
import org.conqat.engine.commons.util.LineRangeDeserializer;
import org.conqat.engine.commons.util.LineRangeSerializer;
import org.conqat.engine.index.shared.tests.ETestExecutionResult;
import org.conqat.lib.commons.collections.CompactLines;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import com.fasterxml.jackson.databind.annotation.JsonSerialize;
/**
* Represents a set of tests that are executed together. May support executing a subset of the tests
* it represents. May also always execute all tests. Examples include test binaries built for C/C++
* code or test Jar files for Java code. In the build one would execute all impacted tests for these
* execution units at once to avoid overhead.
*
* This class is a DTO used to deserialize testwise coverage reports of version 2+. Field names and
* structure may not be changed.
*/
public class ExecutionUnitInfo {
/**
* Unique name of the test case by using a path like hierarchical description, which can be shown in
* the UI.
*/
@JsonProperty("uniformPath")
private String uniformPath;
/**
* The overall result of the execution of the execution unit. May differ from the combined result of
* tests contained in the execution unit.
*/
@JsonProperty("result")
@Nullable
private ETestExecutionResult result;
/** A hash determining the version of the execution unit. */
@JsonProperty("hash")
@Nullable
private String hash;
/** The duration in seconds it took to execute the execution unit. */
@JsonProperty("duration")
@Nullable
private Double durationSeconds;
/**
* The coverage produced by executing all the tests in the execution unit. A map of strings to line
* range strings. The keys are the integer indexes in string form of the {@link FileInfo} objects in
* the top-level files array. The values are the line range strings representing the covered lines.
*/
@JsonProperty("coverage")
@JsonSerialize(contentUsing = LineRangeSerializer.class)
@JsonDeserialize(contentUsing = LineRangeDeserializer.class)
private Map coverage;
/** The tests contained in the unit. */
@JsonProperty("tests")
private final List tests = new ArrayList<>();
/**
* Link to an external build or test management tool that offers information about the run of the
* execution unit.
*/
@JsonProperty("externalLink")
@Nullable
private String externalLink;
public String getUniformPath() {
return uniformPath;
}
public void setUniformPath(String uniformPath) {
this.uniformPath = uniformPath;
}
public @Nullable ETestExecutionResult getResult() {
return result;
}
public void setResult(@Nullable ETestExecutionResult result) {
this.result = result;
}
public @Nullable String getHash() {
return hash;
}
public void setHash(@Nullable String hash) {
this.hash = hash;
}
public @Nullable Double getDurationSeconds() {
return durationSeconds;
}
public void setDurationSeconds(@Nullable Double durationSeconds) {
this.durationSeconds = durationSeconds;
}
public Map getCoverage() {
return coverage;
}
public void setCoverage(@Nullable Map coverage) {
this.coverage = coverage;
}
public List getTests() {
return tests;
}
public @Nullable String getExternalLink() {
return externalLink;
}
public void setExternalLink(@Nullable String externalLink) {
this.externalLink = externalLink;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy