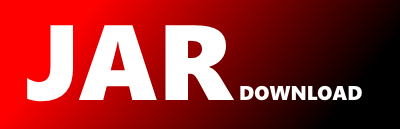
org.conqat.engine.sourcecode.coverage.testwise_coverage.TestInfo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of teamscale-commons Show documentation
Show all versions of teamscale-commons Show documentation
Provides common DTOs for Teamscale
The newest version!
package org.conqat.engine.sourcecode.coverage.testwise_coverage;
import java.util.List;
import java.util.Map;
import org.checkerframework.checker.nullness.qual.Nullable;
import org.conqat.engine.commons.util.LineRangeDeserializer;
import org.conqat.engine.commons.util.LineRangeSerializer;
import org.conqat.engine.index.shared.tests.ETestExecutionResult;
import org.conqat.lib.commons.collections.CollectionUtils;
import org.conqat.lib.commons.collections.CompactLines;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import com.fasterxml.jackson.databind.annotation.JsonSerialize;
/**
* Generic container of all information about a specific test as written to the report.
*
* This class is a DTO used to deserialize testwise coverage reports. Field names and structure may
* not be changed.
*/
public class TestInfo {
/** The name of the JSON property name for {@link #uniformPath}. */
private static final String UNIFORM_PATH_PROPERTY = "uniformPath";
/** The name of the JSON property name for {@link #testLocations}. */
private static final String TEST_LOCATION_PROPERTY = "testLocations";
/** The name of the JSON property name for {@link #content}. */
private static final String CONTENT_PROPERTY = "content";
/** The name of the JSON property name for {@link #duration}. */
private static final String DURATION_PROPERTY = "duration";
/** The name of the JSON property name for {@link #result}. */
private static final String RESULT_PROPERTY = "result";
/** The name of the JSON property name for {@link #message}. */
private static final String MESSAGE_PROPERTY = "message";
/** The name of the JSON property for {@link #readableName}. */
private static final String READABLE_NAME_PROPERTY = "readableName";
/** The name of the JSON property for {@link #parameters}. */
private static final String PARAMETERS_PROPERTY = "parameters";
/** The name of the JSON property for {@link #hash}. */
private static final String HASH_PROPERTY = "hash";
/** The name of the JSON property for {@link #coverage}. */
private static final String COVERAGE_PROPERTY = "coverage";
/** The name of the JSON property for {@link #properties}. */
private static final String PROPERTIES_PROPERTY = "properties";
/** The name of the JSON property for {@link #externalLink}. */
private static final String EXTERNAL_LINK_PROPERTY = "externalLink";
/** The name of the JSON property for {@link #associatedSpecItems}. */
private static final String ASSOCIATED_SPEC_ITEMS_PROPERTY = "associatedSpecItems";
/**
* Unique name of the test case by using a path like hierarchical description, which can be shown in
* the UI. Present in reports of all versions.
*/
@JsonProperty(UNIFORM_PATH_PROPERTY)
public final String uniformPath;
/**
* A list of ANT-patterns limiting the search space for a corresponding test implementation.
*/
@JsonProperty(TEST_LOCATION_PROPERTY)
public final List testLocations;
/**
* A list of parameters with which the test has been executed. The order of the parameters in the
* list is significant.
*
* Present in reports of version 2+.
*/
@JsonProperty(PARAMETERS_PROPERTY)
@Nullable
public final List parameters;
/**
* Some kind of content to tell whether the test specification has changed. Can be revision number
* or hash over the specification or similar. Present in reports of version 1.
*/
@JsonProperty(CONTENT_PROPERTY)
@Nullable
public final String content;
/** Duration of the execution in seconds. Present in reports of all versions. */
@JsonProperty(DURATION_PROPERTY)
public final Double duration;
/** The actual execution result state. Present in reports of all versions. */
@JsonProperty(RESULT_PROPERTY)
public final ETestExecutionResult result;
/**
* Optional message given for test failures (normally contains a stack trace). May be {@code null}.
* Present in reports of all versions.
*/
@JsonProperty(MESSAGE_PROPERTY)
@Nullable
public final String message;
/** Present in reports of version 1. */
@JsonProperty("paths")
@Nullable
public List paths;
/**
* A readable name for the test that must not be unique which can be shown in the UI. Present in
* reports of version 2+.
*/
@JsonProperty(READABLE_NAME_PROPERTY)
@Nullable
public final String readableName;
/**
* A hash determining the version of the test implementation. Present in reports of version 2+.
*/
@JsonProperty(HASH_PROPERTY)
@Nullable
public final String hash;
/**
* A map of strings to line range strings. The keys are the integer indexes in string form of the
* {@link FileInfo} objects in the top-level files array. The values are the line range strings
* representing the covered lines. If omitted, only the values of the other fields are updated in
* Teamscale, but the coverage isn't modified. Present in reports of version 2+.
*/
@JsonProperty(COVERAGE_PROPERTY)
@Nullable
@JsonSerialize(contentUsing = LineRangeSerializer.class)
@JsonDeserialize(contentUsing = LineRangeDeserializer.class)
public final Map coverage;
/**
* A map of strings that can carry additional properties that are displayed on the test details
* page. E.g., ID that identifies the test case or the test run in an external system or the name of
* a tester if the test is executed manually. Present in reports of version 2+.
*/
@JsonProperty(PROPERTIES_PROPERTY)
@Nullable
public final Map properties;
/**
* Link to an external build or test management tool that offers information about the run of the
* execution unit. Present in reports of version 2+.
*/
@JsonProperty(EXTERNAL_LINK_PROPERTY)
@Nullable
public final String externalLink;
/**
* List of unparsed spec items that are verified by this test.
*/
@JsonProperty(ASSOCIATED_SPEC_ITEMS_PROPERTY)
@Nullable
public final List associatedSpecItems;
public TestInfo(String uniformPath) {
this.uniformPath = uniformPath;
testLocations = null;
parameters = null;
content = null;
duration = null;
result = null;
message = null;
readableName = null;
hash = null;
coverage = null;
properties = null;
externalLink = null;
associatedSpecItems = null;
}
public TestInfo(String uniformPath, @Nullable Double duration, @Nullable ETestExecutionResult result,
@Nullable Map coverage) {
this.uniformPath = uniformPath;
this.duration = duration;
this.result = result;
this.coverage = coverage;
testLocations = null;
parameters = null;
content = null;
message = null;
readableName = null;
hash = null;
paths = null;
properties = null;
externalLink = null;
associatedSpecItems = null;
}
/**
* A constructor that copies all the fields of an existing TestInfo object, except of a few fields
* calculated by teamscale-build. This is a means to reduce the maintenance work in teamscale-build
* if the TestInfo object is updated with additional fields (as they can then be set by the
* metadata.json, see teamscale-build).
*/
public TestInfo(TestInfo other, @Nullable Double duration, @Nullable ETestExecutionResult result,
@Nullable Map coverage, @Nullable String content) {
this.uniformPath = other.uniformPath;
this.testLocations = other.testLocations;
this.parameters = other.parameters;
this.content = content;
this.duration = duration;
this.result = result;
this.message = other.message;
this.readableName = other.readableName;
this.hash = other.hash;
this.coverage = coverage;
this.properties = other.properties;
this.externalLink = other.externalLink;
this.associatedSpecItems = other.associatedSpecItems;
}
@JsonCreator
public TestInfo(@JsonProperty(UNIFORM_PATH_PROPERTY) String uniformPath,
@JsonProperty(TEST_LOCATION_PROPERTY) List testLocations,
@JsonProperty(PARAMETERS_PROPERTY) @Nullable List parameters,
@JsonProperty(CONTENT_PROPERTY) @Nullable String content, @JsonProperty(DURATION_PROPERTY) Double duration,
@JsonProperty(RESULT_PROPERTY) ETestExecutionResult result,
@JsonProperty(MESSAGE_PROPERTY) @Nullable String message,
@JsonProperty(READABLE_NAME_PROPERTY) @Nullable String readableName,
@JsonProperty(HASH_PROPERTY) @Nullable String hash,
@JsonProperty(COVERAGE_PROPERTY) @Nullable Map coverage,
@JsonProperty(PROPERTIES_PROPERTY) @Nullable Map properties,
@JsonProperty(EXTERNAL_LINK_PROPERTY) @Nullable String externalLink,
@JsonProperty(ASSOCIATED_SPEC_ITEMS_PROPERTY) @Nullable List associatedSpecItems) {
this.uniformPath = uniformPath;
this.testLocations = testLocations;
this.parameters = parameters;
this.content = content;
this.duration = duration;
this.result = result;
this.message = message;
this.readableName = readableName;
this.hash = hash;
this.coverage = coverage;
this.properties = properties;
this.externalLink = externalLink;
this.associatedSpecItems = associatedSpecItems;
}
/**
* Returns whether all required fields in testwise coverage V1 are empty. This meant that the test
* was not executed, but still exists and should not be deleted as part of the upload.
*/
@JsonIgnore
public boolean isEmpty() {
return content == null && duration == null && result == null && CollectionUtils.isNullOrEmpty(paths);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy