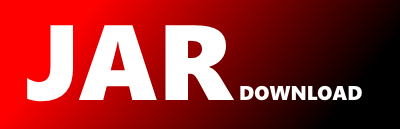
org.conqat.lib.commons.uniformpath.UniformPathCompatibilityUtil Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of teamscale-commons Show documentation
Show all versions of teamscale-commons Show documentation
Provides common DTOs for Teamscale
The newest version!
/*
* Copyright (c) CQSE GmbH
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.conqat.lib.commons.uniformpath;
import java.util.Arrays;
import java.util.Collection;
import java.util.List;
import java.util.Set;
import org.conqat.engine.resource.util.UniformPathUtils;
import org.conqat.lib.commons.collections.CollectionUtils;
import org.conqat.lib.commons.collections.PairList;
import com.google.common.base.Preconditions;
/**
* Utility methods to aid the migration from string-typed uniform paths to type-safe
* {@link UniformPath}s. Can be removed when/if string-typed uniform paths are no longer relevant.
*/
public class UniformPathCompatibilityUtil {
/** Converts the given string to a {@link UniformPath}. */
public static UniformPath convert(String uniformPath) {
return UniformPath.of(getAbsoluteSegments(uniformPath));
}
/** Converts the given string and type to a {@link UniformPath}. */
public static UniformPath convert(UniformPath.EType type, String uniformPath) {
return UniformPath.of(type, getAbsoluteSegments(uniformPath));
}
/** Converts the given string to a {@link RelativeUniformPath}. */
public static RelativeUniformPath convertRelative(String uniformPath) {
Preconditions.checkNotNull(uniformPath, "Uniform path must not be null");
return RelativeUniformPath.of(UniformPathUtils.splitPath(uniformPath.trim()));
}
/** Splits the path into segments and resolves relative parts. */
/* package */static List getAbsoluteSegments(String uniformPath) {
Preconditions.checkNotNull(uniformPath, "Uniform path must not be null");
return RelativeUniformPath
.resolveRelativeSegments(Arrays.asList(UniformPathUtils.splitPath(uniformPath.trim())));
}
/** Converts the given strings to {@link UniformPath}s. */
public static List convertCollection(Collection uniformPaths) {
return CollectionUtils.map(uniformPaths, UniformPathCompatibilityUtil::convert);
}
/** Converts the given strings to {@link UniformPath}s. */
public static Set convertSet(Collection uniformPaths) {
return CollectionUtils.mapToSet(uniformPaths, UniformPathCompatibilityUtil::convert);
}
/**
* Returns the {@link String} representations of the given {@link UniformPath}s.
*/
public static List asUniformPathStrings(Collection uniformPaths) {
return CollectionUtils.map(uniformPaths, UniformPath::toString);
}
/**
* Returns the {@link String} representations of the given {@link UniformPath}s.
*/
public static Set asUniformPathStringSet(Collection uniformPaths) {
return CollectionUtils.mapToSet(uniformPaths, UniformPath::toString);
}
/**
* Converts a pair list containing {@link UniformPath}s back to a string-typed uniform path pair
* list.
*/
public static PairList convertPairList(PairList pairList) {
return pairList.mapFirst(UniformPath::toString);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy