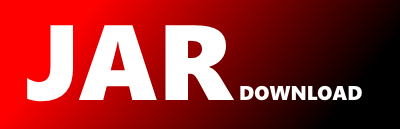
org.conqat.engine.service.shared.ServiceUtils Maven / Gradle / Ivy
/*-------------------------------------------------------------------------+
| |
| Copyright 2005-2011 the ConQAT Project |
| |
| Licensed under the Apache License, Version 2.0 (the "License"); |
| you may not use this file except in compliance with the License. |
| You may obtain a copy of the License at |
| |
| http://www.apache.org/licenses/LICENSE-2.0 |
| |
| Unless required by applicable law or agreed to in writing, software |
| distributed under the License is distributed on an "AS IS" BASIS, |
| WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. |
| See the License for the specific language governing permissions and |
| limitations under the License. |
+-------------------------------------------------------------------------*/
package org.conqat.engine.service.shared;
import java.net.MalformedURLException;
import java.net.URI;
import java.net.URISyntaxException;
import java.net.URL;
import java.util.Optional;
import java.util.function.Function;
import org.conqat.engine.resource.util.UniformPathUtils;
import org.conqat.lib.commons.string.StringUtils;
import com.google.common.net.UrlEscapers;
/**
* Utility methods for services.
*/
public class ServiceUtils {
/**
* Encodes the given url path segment. Slashes will be escaped. If you want to
* keep them you need to split the input before and supply them one by one.
*/
public static String encodePathSegment(String pathSegment) {
return UrlEscapers.urlPathSegmentEscaper().escape(pathSegment);
}
/**
* Encodes the given value as query parameter (includes slashes and + symbols).
*/
public static String encodeQueryParameter(String queryParameter) {
return UrlEscapers.urlFormParameterEscaper().escape(queryParameter);
}
/**
* Encodes the given uniform path by URL-encoding each segment while preserving
* the slashes.
*/
public static String encodeUniformPath(String uniformPath) {
boolean leadingSlash = uniformPath.startsWith("/");
String[] parts = UniformPathUtils.splitPath(uniformPath);
for (int i = 0; i < parts.length; i++) {
parts[i] = encodePathSegment(parts[i]);
}
if (leadingSlash) {
return "/" + UniformPathUtils.concatenate(parts);
}
return UniformPathUtils.concatenate(parts);
}
/** Uses Java API to check if the server address is valid */
public static boolean isValidServerAddress(String address) {
try {
// Check whether the given address is both a valid URL and a valid
// URI.
new URL(address);
new URI(address);
} catch (URISyntaxException e) {
return false;
} catch (MalformedURLException e) {
return false;
}
return true;
}
/**
* Produces a specific error message if the identifier is invalid (e.g.,
* contains linebreaks, invisible unicode chars, or is empty). The error message
* contains the (truncated) text if it is not empty.
*
* @param identifierText
* the text of the identifier (e.g., "x" or "foo")
* @param identifierDescription
* a description of what this identifier should be (e.g., "Group
* name" or "Id"). This is used at the beginning of the error message
* and therefore should start with an uppercase letter.
*/
public static Optional getErrorMessageForInvalidIdentifiers(String identifierText,
String identifierDescription) {
Function truncate = identifier -> "\""
+ StringUtils.removeAll(StringUtils.truncate(identifier, 9, "..."), "\n") + "\"";
if (identifierText.contains("\n")) {
return Optional.of(identifierDescription + " " + truncate.apply(identifierText) + " contains linebreaks");
}
if (identifierText.matches(".*\\p{C}.*")) {
return Optional.of(identifierDescription + " " + truncate.apply(identifierText)
+ " contains invisible Unicode control characters");
}
if (identifierText.equals(StringUtils.EMPTY_STRING)) {
return Optional.ofNullable(identifierDescription + " is empty");
}
return Optional.empty();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy