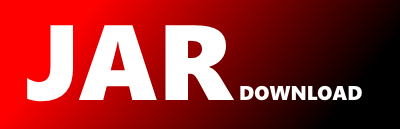
org.conqat.engine.sourcecode.coverage.TestDetails Maven / Gradle / Ivy
package org.conqat.engine.sourcecode.coverage;
import java.io.Serializable;
import java.util.Objects;
import javax.annotation.Nonnull;
import javax.annotation.Nullable;
import org.conqat.lib.commons.js_export.ExportToTypeScript;
import org.conqat.lib.commons.uniformpath.UniformPath;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonProperty;
/**
* Represents a test case data class, holding an uniformPath (Teamscale
* internal).
*/
@ExportToTypeScript
public class TestDetails implements Comparable, Serializable {
private static final long serialVersionUID = 1L;
/** The name of the JSON property name for {@link #uniformPath}. */
protected static final String UNIFORM_PATH_PROPERTY = "uniformPath";
/** The name of the JSON property name for {@link #sourcePath}. */
protected static final String SOURCE_PATH_PROPERTY = "sourcePath";
/** The name of the JSON property name for {@link #content}. */
protected static final String CONTENT_PROPERTY = "content";
/** The name of the JSON property name for {@link #lastChangedTimestamp}. */
protected static final String LAST_CHANGED_TIMESTAMP_PROPERTY = "lastChangedTimestamp";
/** The uniform path the test (unescaped and without -test- prefix). */
@JsonProperty(UNIFORM_PATH_PROPERTY)
public String uniformPath;
/**
* Path to the source of the method. Will be equal to {@link #uniformPath} in
* most cases, but e.g. @Test
methods in a base class will have the
* sourcePath pointing to the base class which contains the actual
* implementation, whereas {@link #uniformPath} will contain the class name of
* the most specific subclass from where it was actually executed.
*/
@JsonProperty(SOURCE_PATH_PROPERTY)
@Nullable
private String sourcePath;
/**
* Some kind of content to tell whether the test specification has changed. Can
* be revision number or hash over the specification or similar.
*/
@JsonProperty(CONTENT_PROPERTY)
@Nullable
private String content;
/** The last timestamp at which the content of the test did change. */
@JsonProperty(LAST_CHANGED_TIMESTAMP_PROPERTY)
private long lastChangedTimestamp;
public TestDetails(String uniformPath, String content) {
this(uniformPath, null, content, 0L);
}
public TestDetails(String uniformPath, String sourcePath, String content) {
this(uniformPath, sourcePath, content, 0L);
}
@JsonCreator
public TestDetails(@JsonProperty(UNIFORM_PATH_PROPERTY) String uniformPath,
@JsonProperty(SOURCE_PATH_PROPERTY) String sourcePath, @JsonProperty(CONTENT_PROPERTY) String content,
@JsonProperty(LAST_CHANGED_TIMESTAMP_PROPERTY) long lastChangedTimestamp) {
this.uniformPath = uniformPath;
this.content = content;
this.sourcePath = sourcePath;
this.lastChangedTimestamp = lastChangedTimestamp;
}
/** {@inheritDoc} */
@Override
public int compareTo(@Nonnull TestDetails other) {
return uniformPath.compareTo(other.uniformPath);
}
/** We explicitly don't check {@link #lastChangedTimestamp} here. */
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
TestDetails that = (TestDetails) o;
return Objects.equals(uniformPath, that.uniformPath) && Objects.equals(sourcePath, that.sourcePath)
&& Objects.equals(content, that.content);
}
/** We explicitly don't check {@link #lastChangedTimestamp} here. */
@Override
public int hashCode() {
return Objects.hash(uniformPath, sourcePath, content);
}
/** Compute the test execution path. */
public String getUniformPath() {
return TestUniformPathUtils.convertToTestUniformPathString(uniformPath);
}
/** Compute the test execution path. */
public UniformPath toUniformPath() {
return TestUniformPathUtils.convertToUniformPath(uniformPath);
}
/** @see #sourcePath */
public String getSourcePath() {
return sourcePath;
}
/** @see #sourcePath */
public void setSourcePath(String sourcePath) {
this.sourcePath = sourcePath;
}
/** @see #content */
public String getContent() {
return content;
}
/** @see #content */
public void setContent(String content) {
this.content = content;
}
/** @see #lastChangedTimestamp */
public long getLastChangedTimestamp() {
return lastChangedTimestamp;
}
/** @see #lastChangedTimestamp */
public void setLastChangedTimestamp(long lastChangedTimestamp) {
this.lastChangedTimestamp = lastChangedTimestamp;
}
@Override
public String toString() {
return uniformPath;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy