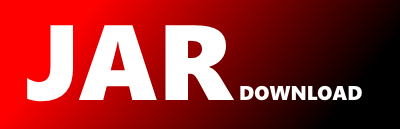
org.conqat.engine.sourcecode.coverage.TestUniformPathUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of teamscale-commons Show documentation
Show all versions of teamscale-commons Show documentation
Provides common DTOs for Teamscale
package org.conqat.engine.sourcecode.coverage;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import org.conqat.engine.resource.util.UniformPathUtils;
import org.conqat.lib.commons.collections.Pair;
import org.conqat.lib.commons.string.StringUtils;
import org.conqat.lib.commons.uniformpath.UniformPath;
import org.conqat.lib.commons.uniformpath.UniformPathCompatibilityUtil;
import com.google.common.base.Preconditions;
/**
* Utility methods for converting test IDs to uniform paths and the other way
* around.
*/
public class TestUniformPathUtils {
/**
* Converts the given test id string to a uniform path considering that the test
* name may contain parameters.
*/
public static UniformPath convertToUniformPath(String testUniformPath) {
Preconditions.checkArgument(!testUniformPath.startsWith(UniformPath.EType.TEST.getPrefix()));
Pair testPathAndName = getTestPathAndName(testUniformPath);
String testPath = testPathAndName.getFirst();
String testName = testPathAndName.getSecond();
return UniformPathCompatibilityUtil.convertRelative(testPath).addSuffix(UniformPath.escapeSegment(testName))
.resolveAgainstAbsolutePath(UniformPath.testRoot());
}
/**
* Converts the given test id string to a uniform path considering that the test
* name may contain parameters.
*/
public static String convertToTestUniformPathString(String uniformPath) {
Preconditions.checkArgument(!uniformPath.startsWith(UniformPath.EType.TEST.getPrefix()));
Pair testPathAndName = getTestPathAndName(uniformPath);
String testPath = testPathAndName.getFirst();
String testName = testPathAndName.getSecond();
String testUniformPath = UniformPath.escapeSegment(testName);
if (!testPath.isEmpty()) {
testUniformPath = testPath + "/" + testUniformPath;
}
return UniformPath.EType.TEST.getPrefix() + "/" + testUniformPath;
}
/**
* Returns a tuple of test path and test name. Splitting happens at the last
* slash that is not contained in a pair of brackets.
*/
private static Pair getTestPathAndName(String testUniformPath) {
List segments = Arrays.asList(testUniformPath.split("/"));
for (int i = 0; i < segments.size(); i++) {
if (segments.get(i).contains("[") || i == segments.size() - 1) {
String testPath = StringUtils.concat(segments.subList(0, i), "/");
String testName = StringUtils.concat(segments.subList(i, segments.size()), "/");
return new Pair<>(testPath, testName);
}
}
return new Pair<>("", testUniformPath);
}
/**
* Strips await the -test-/ prefix of a test uniform path and removes escaping
* so that the result matches the test id the test runner gave us.
*/
public static String convertToTestId(String uniformPath) {
String testIdWithoutPrefix = StringUtils.stripPrefix(uniformPath,
UniformPath.EType.TEST.getPrefix() + UniformPathUtils.SEPARATOR);
return StringUtils.unEscapeChars(testIdWithoutPrefix, Collections.singletonList('/'));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy