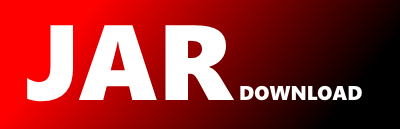
org.conqat.engine.index.shared.ApacheMinaSshSessionFactory Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of teamscale-commons Show documentation
Show all versions of teamscale-commons Show documentation
Provides common DTOs for Teamscale
/*-------------------------------------------------------------------------+
| |
| Copyright (c) 2009-2019 CQSE GmbH |
| |
+-------------------------------------------------------------------------*/
package org.conqat.engine.index.shared;
import java.io.ByteArrayInputStream;
import java.io.File;
import java.io.IOException;
import java.net.InetSocketAddress;
import java.security.GeneralSecurityException;
import java.security.KeyPair;
import java.security.PublicKey;
import java.util.Collections;
import java.util.Iterator;
import java.util.List;
import org.apache.sshd.common.NamedResource;
import org.apache.sshd.common.config.keys.FilePasswordProvider;
import org.apache.sshd.common.keyprovider.KeyIdentityProvider;
import org.apache.sshd.common.session.SessionContext;
import org.apache.sshd.common.util.security.SecurityUtils;
import org.conqat.engine.index.shared.GitUtils.TeamscaleGitCredentialsProvider;
import org.eclipse.jgit.transport.CredentialItem.Password;
import org.eclipse.jgit.transport.CredentialsProvider;
import org.eclipse.jgit.transport.SshSessionFactory;
import org.eclipse.jgit.transport.URIish;
import org.eclipse.jgit.transport.sshd.ServerKeyDatabase;
import org.eclipse.jgit.transport.sshd.SshdSessionFactory;
/**
* An {@link SshSessionFactory} based on Apache Mina.
*
* @see JGit
* 5.2 New and Noteworthy
*/
/* package */ class ApacheMinaSshSessionFactory extends SshdSessionFactory {
private final URIish uri;
private final TeamscaleGitCredentialsProvider credentials;
/* package */ ApacheMinaSshSessionFactory(URIish location, TeamscaleGitCredentialsProvider credentials) {
uri = location;
this.credentials = credentials;
}
@Override
protected File getSshConfig(File sshDir) {
return null;
}
@Override
protected String getDefaultPreferredAuthentications() {
return "publickey";
}
@Override
protected ServerKeyDatabase getServerKeyDatabase(File homeDir, File sshDir) {
return new ServerKeyDatabase() {
@Override
public List lookup(String connectAddress, InetSocketAddress remoteAddress,
Configuration config) {
// There are no pre-configured public keys for remote servers.
return Collections.emptyList();
}
@Override
public boolean accept(String connectAddress, InetSocketAddress remoteAddress, PublicKey serverKey,
Configuration config, CredentialsProvider provider) {
// Accept any remote server, as we have no way to configure its public key.
return true;
}
};
}
@Override
protected Iterable getDefaultKeys(File sshDir) {
return new KeyAuthenticator();
}
private class KeyAuthenticator implements KeyIdentityProvider, Iterable {
@Override
public Iterator iterator() {
throw new UnsupportedOperationException(
"The need to implement Iterable is an left-over of JGit 2.0's API; iterator() has been replacyed by loadKeys(..)");
}
@Override
public Iterable loadKeys(SessionContext session) throws IOException, GeneralSecurityException {
return SecurityUtils.loadKeyPairIdentities(session, null,
new ByteArrayInputStream(credentials.getSshPrivateKey().getBytes()), new FilePasswordProvider() {
@Override
public String getPassword(SessionContext session, NamedResource resourceKey, int retryIndex) {
Password password = new Password();
credentials.get(uri, password);
return new String(password.getValue());
}
});
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy