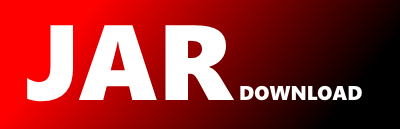
org.conqat.engine.index.shared.BasicTokenElementInfo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of teamscale-commons Show documentation
Show all versions of teamscale-commons Show documentation
Provides common DTOs for Teamscale
/*-------------------------------------------------------------------------+
| |
| Copyright 2005-2011 the ConQAT Project |
| |
| Licensed under the Apache License, Version 2.0 (the "License"); |
| you may not use this file except in compliance with the License. |
| You may obtain a copy of the License at |
| |
| http://www.apache.org/licenses/LICENSE-2.0 |
| |
| Unless required by applicable law or agreed to in writing, software |
| distributed under the License is distributed on an "AS IS" BASIS, |
| WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. |
| See the License for the specific language governing permissions and |
| limitations under the License. |
+-------------------------------------------------------------------------*/
package org.conqat.engine.index.shared;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Optional;
import org.conqat.engine.index.shared.element_details.TokenElementDetailBase;
import org.conqat.engine.resource.text.filter.base.Deletion;
import org.conqat.engine.resource.text.filter.util.StringOffsetTransformer;
import org.conqat.lib.commons.collections.CollectionUtils;
import org.conqat.lib.commons.collections.UnmodifiableList;
import org.conqat.lib.commons.js_export.ExportToTypeScript;
import com.fasterxml.jackson.annotation.JsonProperty;
import eu.cqse.check.framework.scanner.ELanguage;
/**
* Transport object with the information needed for a token element.
*
* This class is immutable.
*/
@ExportToTypeScript
public class BasicTokenElementInfo implements Serializable {
/** Serial version UID. */
private static final long serialVersionUID = 1;
/** The uniform path. */
@JsonProperty("uniformPath")
protected final String uniformPath;
/** The language. */
@JsonProperty("language")
protected final ELanguage language;
/** The text content. */
@JsonProperty("text")
protected final String text;
/** The list of filtered regions */
@JsonProperty("filterDeletions")
protected final ArrayList filterDeletions;
/** Additional details of this info object. */
@JsonProperty("details")
protected final ArrayList details;
/** Constructor. */
public BasicTokenElementInfo(String uniformPath, ELanguage language, String text,
Collection filterDeletions, Collection details) {
this.uniformPath = uniformPath;
this.language = language;
this.text = text;
this.filterDeletions = new ArrayList<>(filterDeletions);
Collections.sort(this.filterDeletions);
this.details = new ArrayList<>(details);
}
/** Copy constructor. */
protected BasicTokenElementInfo(BasicTokenElementInfo other) {
this(other.uniformPath, other.language, other.text, other.filterDeletions, other.details);
}
/** Returns the uniform path. */
public String getUniformPath() {
return uniformPath;
}
/** Returns the language. */
public ELanguage getLanguage() {
return language;
}
/**
* Returns the text content. Note that this is the raw content,
* not taking into account content filters stored in
* {@link #filterDeletions}. To obtain the filtered content, transform this into
* an ITokenElement.
*/
public String getText() {
return text;
}
/**
* Returns the filtered text of this token element. Please note that the
* filtered content is computed on the fly.
*/
public String getFilteredText() {
return new StringOffsetTransformer(filterDeletions).filterString(text);
}
/** Returns the filter deletions. */
public UnmodifiableList getFilterDeletions() {
return CollectionUtils.asUnmodifiable(filterDeletions);
}
/** @see #details */
public UnmodifiableList getDetails() {
return CollectionUtils.asUnmodifiable(details);
}
/** Returns the first detail of given type (or null). */
public Optional getFirstDetailOfType(Class type) {
return getFirstDetailOfType(type, details);
}
/** Returns the first detail of given type (or null) in the given list. */
@SuppressWarnings("unchecked")
public static Optional getFirstDetailOfType(Class type,
List details) {
for (TokenElementDetailBase detail : details) {
if (type.isInstance(detail)) {
return Optional.of((T) detail);
}
}
return Optional.empty();
}
/** {@inheritDoc} */
@Override
public String toString() {
return uniformPath;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy