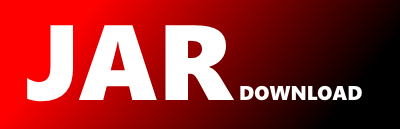
org.conqat.engine.index.shared.MergeRequestIdentifier Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of teamscale-commons Show documentation
Show all versions of teamscale-commons Show documentation
Provides common DTOs for Teamscale
/*-------------------------------------------------------------------------+
| |
| Copyright (c) 2009-2020 CQSE GmbH |
| |
+-------------------------------------------------------------------------*/
package org.conqat.engine.index.shared;
import java.io.Serializable;
import java.util.Objects;
import org.conqat.lib.commons.collections.Pair;
import org.conqat.lib.commons.js_export.ExportToTypeScript;
import org.conqat.lib.commons.string.StringUtils;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.google.common.base.Preconditions;
/**
* Identifier for merge requests of a repository.
*/
@ExportToTypeScript
public class MergeRequestIdentifier implements Serializable {
private static final long serialVersionUID = 1L;
/** Separator for String representation. */
private static final char SEPARATOR = '/';
/** The name of the repository the merge request belongs to. */
@JsonProperty("repositoryName")
public final String repositoryName;
/** The merge request ID. */
@JsonProperty("id")
public final long id;
public MergeRequestIdentifier(String repositoryName, long id) {
this.repositoryName = repositoryName;
this.id = id;
}
/**
* Converts the toString representation of this object from a string back into a
* {@link MergeRequestIdentifier}.
*/
public static MergeRequestIdentifier fromString(String value) {
Pair parts = StringUtils.splitAtLast(value, SEPARATOR);
Preconditions.checkNotNull(parts.getSecond(), "Merge request identifier has wrong format: %s", value);
return new MergeRequestIdentifier(parts.getFirst(), Long.parseLong(parts.getSecond()));
}
/** {@inheritDoc} */
@Override
@JsonProperty("idWithRepository")
public String toString() {
return repositoryName + SEPARATOR + id;
}
/** {@inheritDoc} */
@Override
public boolean equals(Object other) {
if (this == other) {
return true;
}
if (other == null || getClass() != other.getClass()) {
return false;
}
MergeRequestIdentifier identifier = (MergeRequestIdentifier) other;
return id == identifier.id && repositoryName.equals(identifier.repositoryName);
}
/** {@inheritDoc} */
@Override
public int hashCode() {
return Objects.hash(repositoryName, id);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy