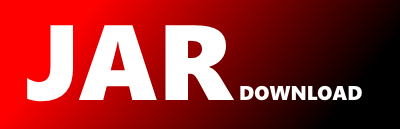
org.conqat.engine.index.shared.ProjectInfo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of teamscale-commons Show documentation
Show all versions of teamscale-commons Show documentation
Provides common DTOs for Teamscale
/*-------------------------------------------------------------------------+
| |
| Copyright 2005-2011 the ConQAT Project |
| |
| Licensed under the Apache License, Version 2.0 (the "License"); |
| you may not use this file except in compliance with the License. |
| You may obtain a copy of the License at |
| |
| http://www.apache.org/licenses/LICENSE-2.0 |
| |
| Unless required by applicable law or agreed to in writing, software |
| distributed under the License is distributed on an "AS IS" BASIS, |
| WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. |
| See the License for the specific language governing permissions and |
| limitations under the License. |
+-------------------------------------------------------------------------*/
package org.conqat.engine.index.shared;
import java.io.Serializable;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
import org.conqat.lib.commons.js_export.ExportToTypeScript;
import org.conqat.lib.commons.string.StringUtils;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.google.common.base.Preconditions;
/**
* Descriptor for a single project.
*/
@ExportToTypeScript
public class ProjectInfo implements Serializable {
/** Serial version UID. */
private static final long serialVersionUID = 1;
/** The name of the JSON property name for {@link #id}. */
private static final String ID_PROPERTY = "id";
/** The name of the JSON property name for {@link #name}. */
private static final String NAME_PROPERTY = "name";
@Override
public boolean equals(Object other) {
if (this == other) {
return true;
}
if (other == null || getClass() != other.getClass()) {
return false;
}
ProjectInfo that = (ProjectInfo) other;
return Objects.equals(id, that.id) && Objects.equals(alias, that.alias) && Objects.equals(name, that.name);
}
@Override
public int hashCode() {
return Objects.hash(id, alias, name);
}
/** The name of the JSON property name for {@link #alias}. */
private static final String ALIAS_PROPERTY = "alias";
/** The name of the JSON property name for {@link #parentProjectId}. */
private static final String PARENT_PROJECT_ID_PROPERTY = "parentProjectId";
/** The name of the JSON property name for {@link #description}. */
private static final String DESCRIPTION_PROPERTY = "description";
/** The name of the JSON property name for {@link #creationTimestamp}. */
private static final String CREATION_TIMESTAMP_PROPERTY = "creationTimestamp";
/** ID of the project */
@JsonProperty(ID_PROPERTY)
private final String id;
/**
* An alias ID that can be used to access this project. This may be null if the
* project has no alias.
*/
@JsonProperty(ALIAS_PROPERTY)
@Nullable
private String alias;
/**
* An optional project id of a parent project, which provides mountable stores
* shared with this project.
*/
@JsonProperty(PARENT_PROJECT_ID_PROPERTY)
@Nullable
private String parentProjectId;
/** The project name. */
@JsonProperty(NAME_PROPERTY)
private String name;
/** The description, may be null. */
@JsonProperty(DESCRIPTION_PROPERTY)
@Nullable
private String description;
/** The creation time of the project. */
@JsonProperty(CREATION_TIMESTAMP_PROPERTY)
private long creationTimestamp;
/** Whether this project is in the process of being deleted. */
@JsonProperty("deleting")
private boolean deleting = false;
/** Whether this project is in the process of being re-analyzed. */
@JsonProperty("reanalyzing")
private boolean reanalyzing = false;
@JsonCreator
public ProjectInfo(@JsonProperty(ID_PROPERTY) String id, @JsonProperty(NAME_PROPERTY) String name,
@JsonProperty(ALIAS_PROPERTY) String alias,
@JsonProperty(PARENT_PROJECT_ID_PROPERTY) String parentProjectId,
@JsonProperty(DESCRIPTION_PROPERTY) String description,
@JsonProperty(CREATION_TIMESTAMP_PROPERTY) long creationTimestamp) {
this.id = id;
this.name = name;
this.alias = alias;
this.parentProjectId = parentProjectId;
this.description = description;
this.creationTimestamp = creationTimestamp;
if (parentProjectId != null) {
Preconditions.checkState(!StringUtils.isEmpty(parentProjectId),
"Can't have an empty parent project id. Must be either null or a non-empty string.");
}
}
/** Returns the project id. */
public String getId() {
return id;
}
/** Returns {@link #alias}. */
public String getAlias() {
return alias;
}
/** Sets {@link #alias}. */
public void setAlias(String alias) {
this.alias = alias;
}
/** @see #parentProjectId */
public Optional getParentProjectId() {
return Optional.ofNullable(parentProjectId);
}
/**
* Returns the {@link #alias} if present. Otherwise the {@link #id} is returned.
* Can be used in case the REST API or UI code expects the alias to shadow the
* id.
*/
public String getProjectAliasOrId() {
if (alias != null) {
return alias;
}
return id;
}
/** Returns {@link #name}. */
public String getName() {
return name;
}
/** Sets {@link #name}. */
public void setName(String name) {
this.name = name;
}
/** Returns {@link #description}. */
public String getDescription() {
return description;
}
/** Sets {@link #description}. */
public void setDescription(String description) {
this.description = description;
}
/** Returns {@link #creationTimestamp}. */
public long getCreationTimestamp() {
return creationTimestamp;
}
/** Sets the creation timestamp. */
public void setCreationTimestamp(long creationTimestamp) {
this.creationTimestamp = creationTimestamp;
}
/** Returns {@link #deleting}. */
public boolean isDeleting() {
return deleting;
}
/** @see #isReanalyzing */
public boolean isReanalyzing() {
return reanalyzing;
}
/**
* Returns whether this project is currently in the process of either being
* reanalyzed or deleted.
*/
public boolean isDeletingOrReanalyzing() {
return isDeleting() || isReanalyzing();
}
/** @see #deleting */
public void setDeleting(boolean deleting) {
this.deleting = deleting;
}
/** @see #isReanalyzing */
public void setReanalyzing(boolean reanalyzing) {
this.reanalyzing = reanalyzing;
}
@Override
public String toString() {
return "ProjectInfo: " + "id='" + id + '\'' + ", alias='" + alias + '\'' + ", parentProjectId='"
+ parentProjectId + '\'' + ", name='" + name + '\'' + ", deleting: " + deleting + ", reanalyzing: "
+ reanalyzing;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy