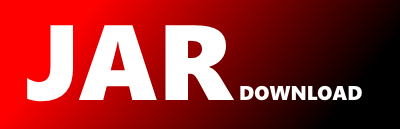
org.conqat.engine.service.shared.client.ServerDetails Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of teamscale-commons Show documentation
Show all versions of teamscale-commons Show documentation
Provides common DTOs for Teamscale
/*-------------------------------------------------------------------------+
| |
| Copyright 2005-2011 the ConQAT Project |
| |
| Licensed under the Apache License, Version 2.0 (the "License"); |
| you may not use this file except in compliance with the License. |
| You may obtain a copy of the License at |
| |
| http://www.apache.org/licenses/LICENSE-2.0 |
| |
| Unless required by applicable law or agreed to in writing, software |
| distributed under the License is distributed on an "AS IS" BASIS, |
| WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. |
| See the License for the specific language governing permissions and |
| limitations under the License. |
+-------------------------------------------------------------------------*/
package org.conqat.engine.service.shared.client;
/**
* Stores all information required to contact a server.
*
* This class is immutable.
*/
public class ServerDetails {
/** The default timeout in seconds. */
private static final int DEFAULT_TIMEOUT_SECONDS = 10;
/** The URL. */
private final String url;
/** The username. */
private final String username;
/** The password. */
private final String password;
/** The connection timeout in seconds. */
private final int timeoutSeconds;
/** Constructor using the default timeout of 10 seconds. */
public ServerDetails(String url, String username, String password) {
this(url, username, password, DEFAULT_TIMEOUT_SECONDS);
}
/** Constructor. */
public ServerDetails(String url, String username, String password, int timeoutSeconds) {
this.url = url;
this.username = username;
this.password = password;
this.timeoutSeconds = timeoutSeconds;
}
/** Returns the URL. */
public String getUrl() {
return url;
}
/** Returns the username. */
public String getUsername() {
return username;
}
/** Returns the password. */
public String getPassword() {
return password;
}
/** Hashing is based on {@link #url} only. */
@Override
public int hashCode() {
return url.hashCode();
}
/** Two ServerDetails are equal if the {@link #url} is the same. */
@Override
public boolean equals(Object obj) {
if (!(obj instanceof ServerDetails)) {
return false;
}
return url.equals(((ServerDetails) obj).url);
}
/** {@inheritDoc} */
@Override
public String toString() {
return username + "@" + url;
}
/** Returns the timeout for the connection to use (in seconds). */
public int getTimeoutSeconds() {
return timeoutSeconds;
}
/** Returns a copy of the server details with the new timeout value. */
public ServerDetails withTimeOut(int timeOutSeconds) {
return new ServerDetails(url, username, password, timeOutSeconds);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy