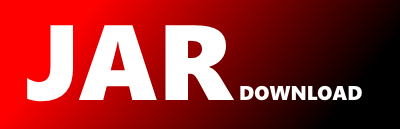
org.conqat.engine.commons.util.NullableFieldValidator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of teamscale-commons Show documentation
Show all versions of teamscale-commons Show documentation
Provides common DTOs for Teamscale
/*
* Copyright (c) CQSE GmbH
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.conqat.engine.commons.util;
import java.lang.reflect.Field;
import java.util.ArrayDeque;
import java.util.ArrayList;
import java.util.Deque;
import java.util.List;
import javax.annotation.Nullable;
import org.conqat.lib.commons.collections.IdentityHashSet;
import org.conqat.lib.commons.reflect.ReflectionUtils;
import org.conqat.lib.commons.string.StringUtils;
import com.teamscale.commons.lang.ToStringHelpers;
/**
* Validator that checks whether all values of an object are non-null unless
* explicitly specified as {@link Nullable}.
*/
public class NullableFieldValidator {
/**
* Ensures that the given object is not null and that all its fields are set
* (throws {@link JsonSerializationException} otherwise). Arrays and other
* iterables are checked recursively, as well as all fields of the given object
* (but only if their type is defined in ConQAT/Teamscale packages).
*
* If you get a false positive for a field from this method, annotate the field
* as {@link Nullable}.
*/
public static T ensureAllFieldsNonNull(T parsedObject, String queryContent) throws JsonSerializationException {
if (StringUtils.isEmpty(queryContent)) {
// Returns null, but that's okay for empty queries
return parsedObject;
}
try {
return ensureAllFieldsNonNull(parsedObject);
} catch (JsonSerializationException e) {
throw new JsonSerializationException(e.getMessage() + "\nQuery: " + queryContent, e);
}
}
/**
* Ensures that the given object is not null and that all its fields are set
* (throws {@link JsonSerializationException} otherwise). Arrays and other
* iterables are checked recursively, as well as all fields of the given object
* (but only if their type is defined in ConQAT/Teamscale packages).
*
* If you get a false positive for a field from this method, annotate the field
* as {@link Nullable}.
*/
public static T ensureAllFieldsNonNull(T parsedObject) throws JsonSerializationException {
if (parsedObject == null) {
throw createNullObjectException("", "whole object");
}
Deque
© 2015 - 2025 Weber Informatics LLC | Privacy Policy