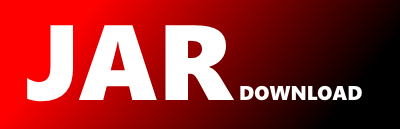
org.conqat.engine.service.shared.data.BranchesInfo Maven / Gradle / Ivy
/*
* Copyright (c) CQSE GmbH
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.conqat.engine.service.shared.data;
import java.util.ArrayList;
import java.util.Collection;
import java.util.List;
import org.conqat.lib.commons.js_export.ExportToTypeScript;
import org.conqat.lib.commons.string.NumbersAwareStringComparator;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.google.common.collect.ImmutableList;
/**
* Data class containing branches of one or more projects.
*
* This class is used as DTO during communication with IDE clients via
* {@link com.teamscale.ide.commons.client.IIdeServiceClient}, special care has
* to be taken when changing its signature!
*/
@ExportToTypeScript
public class BranchesInfo {
/**
* The names of all available active branches, including the default branch, but
* excluding deleted and anonymous branches.
*/
@JsonProperty("liveBranches")
private final List liveBranches = new ArrayList<>();
/** The names of deleted/inactive branches. */
@JsonProperty("deletedBranches")
private final List deletedBranches = new ArrayList<>();
/** A list of anonymous branches or an empty list if non exist. */
@JsonProperty("anonymousBranches")
private final List anonymousBranches = new ArrayList<>();
/**
* The sum of {@link #liveBranches}, {@link #deletedBranches} and
* {@link #anonymousBranches}. Note: This number does not necessarily represent
* the overall amount of branches returned by
* {@link org.conqat.engine.index.repository.ProjectRepositoryChangeIndex.ProjectRepositoryStatus#getBranchNames()}
* but only the number of branches added to this object.
*/
@JsonProperty("currentBranchesCount")
private int currentBranchesCount = 0;
/** Add the given branches to {@link #liveBranches}. */
public void addLiveBranches(Collection liveBranches) {
this.liveBranches.addAll(liveBranches);
currentBranchesCount += liveBranches.size();
}
/** Adds the given branches to {@link #deletedBranches}. */
public void addDeletedBranches(Collection deletedBranches) {
this.deletedBranches.addAll(deletedBranches);
currentBranchesCount += deletedBranches.size();
}
/**
* Adds the given branches to {@link #anonymousBranches}.
*/
public void addAnonymousBranches(Collection anonymousBranches) {
this.anonymousBranches.addAll(anonymousBranches);
currentBranchesCount += anonymousBranches.size();
}
/** @see #liveBranches */
public List getLiveBranches() {
return ImmutableList.copyOf(liveBranches);
}
/** @see #deletedBranches */
public List getDeletedBranches() {
return ImmutableList.copyOf(deletedBranches);
}
/** @see #anonymousBranches */
public List getAnonymousBranches() {
return ImmutableList.copyOf(anonymousBranches);
}
/** @see #currentBranchesCount */
public int getCurrentBranchesCount() {
return currentBranchesCount;
}
/** Sorts the branches. */
public void sortBranches() {
liveBranches.sort(NumbersAwareStringComparator.INSTANCE);
deletedBranches.sort(NumbersAwareStringComparator.INSTANCE);
anonymousBranches.sort(NumbersAwareStringComparator.INSTANCE);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy