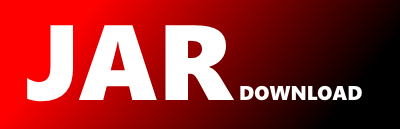
org.conqat.engine.sourcecode.coverage.TestUniformPathUtils Maven / Gradle / Ivy
/*
* Copyright (c) CQSE GmbH
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.conqat.engine.sourcecode.coverage;
import java.util.List;
import org.conqat.engine.resource.util.UniformPathUtils;
import org.conqat.lib.commons.collections.CollectionUtils;
import org.conqat.lib.commons.collections.Pair;
import org.conqat.lib.commons.string.StringUtils;
import org.conqat.lib.commons.uniformpath.UniformPath;
import org.conqat.lib.commons.uniformpath.UniformPathCompatibilityUtil;
import com.google.common.base.Preconditions;
import com.google.common.collect.Iterables;
/**
* Utility methods for converting test IDs to uniform paths and the other way
* around.
*/
public class TestUniformPathUtils {
/** Symbol that starts extending the test with parameters */
public static final String TEST_PARAMETERIZATION_START = "[";
/** Symbol that stops extending the test with parameters */
public static final String TEST_PARAMETERIZATION_END = "]";
/**
* Converts the given test id string to a uniform test path. All of the segments
* in the given path must already be escaped.
*/
public static UniformPath convertToTestUniformPath(String testUniformPath) {
Preconditions.checkArgument(!testUniformPath.startsWith(UniformPath.EType.TEST.getPrefix()));
return UniformPathCompatibilityUtil.convert(UniformPath.EType.TEST, testUniformPath);
}
/**
* Converts the given test name segments to a test path prepending the name of
* the token element. This is used to build test paths for tests that have been
* detected in source code. All of the segments will be escaped. If the
* testNameSegments may contain slashes that should be treated as a new
* directory level use {@link #convertToTestUniformPath(String, List)} instead.
*/
public static UniformPath escapeAndConvertToTestUniformPath(String tokenElementPath, String... testNameSegments) {
Preconditions.checkArgument(!tokenElementPath.startsWith(UniformPath.EType.TEST.getPrefix()));
List escapedSegments = CollectionUtils.map(testNameSegments, UniformPath::escapeSegment);
return convertToTestUniformPath(tokenElementPath, escapedSegments);
}
/**
* Converts the given test name segments to a test path prepending the name of
* the token element. This is used to build test paths for tests that have been
* detected in source code. All of the segments must already be escaped and
* therefore may not contain any unescaped slashes.
*/
public static UniformPath convertToTestUniformPath(String tokenElementPath, List escapedTestSegments) {
Preconditions.checkArgument(!tokenElementPath.startsWith(UniformPath.EType.TEST.getPrefix()));
return UniformPathCompatibilityUtil.convertRelative(tokenElementPath)
.addSuffix(Iterables.toArray(escapedTestSegments, String.class))
.resolveAgainstAbsolutePath(UniformPath.testRoot());
}
/**
* Converts the given path to a uniform test path. If the path contains a test
* parameter, its content must be unescaped, all the other segments must be
* escaped.
*/
public static UniformPath escapeAndConvertToTestUniformPath(String testUniformPath) {
int parameterIndex = testUniformPath.indexOf(TestUniformPathUtils.TEST_PARAMETERIZATION_START);
// If the path does not belong to a parameterized test, no additional escaping
// is required
if (parameterIndex <= 0) {
return UniformPathCompatibilityUtil.convertRelative(testUniformPath)
.resolveAgainstAbsolutePath(UniformPath.testRoot());
}
int lastSegmentIndex = testUniformPath.substring(0, parameterIndex).lastIndexOf("/");
String path = testUniformPath.substring(0, lastSegmentIndex + 1);
// Escape the last segment as it might contain unescaped slashes in the test
// parameter
String lastSegmentEscaped = UniformPath.escapeSegment(testUniformPath.substring(lastSegmentIndex + 1));
return UniformPathCompatibilityUtil.convertRelative(path).addSuffix(lastSegmentEscaped)
.resolveAgainstAbsolutePath(UniformPath.testRoot());
}
/**
* Strips away the -test-/ prefix of a test uniform path and removes escaping so
* that the result matches the test id the test runner gave us.
*/
public static String convertToTestId(String uniformPath) {
String testIdWithoutPrefix = StringUtils.stripPrefix(uniformPath,
UniformPath.EType.TEST.getPrefix() + UniformPathUtils.SEPARATOR);
return StringUtils.unescapeChars(testIdWithoutPrefix, CollectionUtils.asMap(Pair.createPair("/", "\\/")));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy