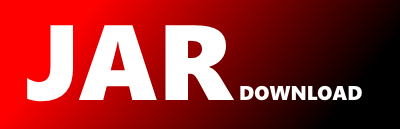
org.conqat.engine.index.GitBranchRefInfo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of teamscale-commons Show documentation
Show all versions of teamscale-commons Show documentation
Provides common DTOs for Teamscale
/*
* Copyright (c) CQSE GmbH
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.conqat.engine.index;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import org.conqat.engine.index.shared.GitUtils;
import org.conqat.lib.commons.assertion.CCSMAssert;
import org.conqat.lib.commons.collections.CollectionUtils;
import org.eclipse.jgit.lib.ObjectId;
import org.eclipse.jgit.lib.Ref;
/**
* Class for determining branch information from a Git repository based on a set
* of Git {@link Ref}s.
*/
public class GitBranchRefInfo {
/**
* The HEAD ref.
*
* @see GitUtils#isHeadRef(Ref)
*/
private final Ref headRef;
/**
* The branch head refs.
*
* @see GitUtils#isBranchHeadRef(Ref)
*/
private final List branchHeadRefs;
private GitBranchRefInfo(Ref headRef, List branchHeadRefs) {
this.branchHeadRefs = CollectionUtils.asUnmodifiable(branchHeadRefs);
this.headRef = headRef;
}
/**
* Returns true if there exists a branch head {@link Ref} for the branch name.
*/
public boolean containsRefForBranchName(String branchName) {
if (branchName == null) {
return false;
}
String branchHeadRefName = GitUtils.createBranchHeadRefName(branchName);
return branchHeadRefs.stream().map(Ref::getName).anyMatch(branchHeadRefName::equals);
}
/**
* Returns the branch names for the branch head {@link Ref}s pointing to the
* same Git revision as the {@link #headRef}; Note that the branch name for the
* {@link #headRef} may not be uniquely defined.
*
* @see GitUtils#HEAD_REF_NAME
*/
public List getBranchNamesMatchingHeadRef() {
if (headRef == null) {
return Collections.emptyList();
}
// Git revision hash for the head ref.
ObjectId headRevision = headRef.getObjectId();
if (headRevision == null) {
return Collections.emptyList();
}
List branchNames = new ArrayList<>(branchHeadRefs.size());
for (Ref branchHeadRef : branchHeadRefs) {
CCSMAssert.isFalse(headRef.equals(branchHeadRef), "Head ref should not be part of the branch head refs.");
if (headRevision.equals(branchHeadRef.getObjectId())) {
GitUtils.getBranchNameFromRef(branchHeadRef).ifPresent(branchNames::add);
}
}
return branchNames;
}
/** Creates a {@link GitBranchRefInfo} for the given {@link Ref}s. */
public static GitBranchRefInfo create(Collection refs) {
Ref headRef = null;
List branchHeadRefs = new ArrayList<>();
for (Ref ref : refs) {
if (GitUtils.isHeadRef(ref)) {
headRef = ref;
} else if (GitUtils.isBranchHeadRef(ref)) {
branchHeadRefs.add(ref);
}
}
return new GitBranchRefInfo(headRef, branchHeadRefs);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy