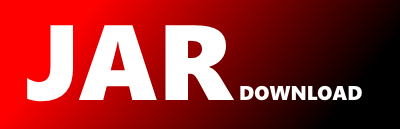
org.conqat.engine.index.shared.ProjectInfo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of teamscale-commons Show documentation
Show all versions of teamscale-commons Show documentation
Provides common DTOs for Teamscale
/*
* Copyright (c) CQSE GmbH
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.conqat.engine.index.shared;
import java.io.Serializable;
import java.util.Comparator;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import org.checkerframework.checker.nullness.qual.Nullable;
import org.conqat.lib.commons.collections.CollectionUtils;
import org.conqat.lib.commons.collections.UnmodifiableList;
import org.conqat.lib.commons.js_export.ExportAsType;
import org.conqat.lib.commons.js_export.ExportToTypeScript;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.google.common.base.Preconditions;
/**
* Descriptor for a single project.
*
* This class is used as DTO during communication with IDE clients via
* {@link com.teamscale.ide.commons.client.IIdeServiceClient}, special care has
* to be taken when changing its signature!
*/
@ExportToTypeScript
public class ProjectInfo implements Serializable, Comparable {
/** Serial version UID. */
private static final long serialVersionUID = 1;
/** The name of the JSON property name for {@link #internalId}. */
private static final String INTERNAL_ID_PROPERTY = "internalId";
/** The name of the JSON property name for {@link #name}. */
private static final String NAME_PROPERTY = "name";
/** The name of the JSON property name for {@link #publicIds}. */
private static final String PUBLIC_IDS_PROPERTY = "publicIds";
/** The name of the JSON property name for {@link #parentProjectId}. */
private static final String PARENT_PROJECT_ID_PROPERTY = "parentProjectId";
/** The name of the JSON property name for {@link #description}. */
private static final String DESCRIPTION_PROPERTY = "description";
/** The name of the JSON property name for {@link #creationTimestamp}. */
private static final String CREATION_TIMESTAMP_PROPERTY = "creationTimestamp";
/** Internal name of the maintenance project. */
public static final String MAINTENANCE_PROJECT_INTERNAL_NAME = "##maintenance##";
/** Internal ID of the project. */
@JsonProperty(INTERNAL_ID_PROPERTY)
// We add "undefined" here to allow the frontend to leave this unspecified
// during creation of a new project (will be auto-generated in that case).
@ExportAsType("string|undefined")
private final InternalProjectId internalId;
/**
* The public IDs of this project. Size of this list is guaranteed to be >= 1,
* and the primary public ID of the project is always the first element of the
* list.
*/
@JsonProperty(PUBLIC_IDS_PROPERTY)
private List publicIds;
/**
* An optional project id of a parent project, which provides mountable stores
* shared with this project.
*/
@JsonProperty(PARENT_PROJECT_ID_PROPERTY)
@Nullable
private InternalProjectId parentProjectId;
/** The project name. */
@JsonProperty(NAME_PROPERTY)
private String name;
/** The description, may be null. */
@JsonProperty(DESCRIPTION_PROPERTY)
@Nullable
private String description;
/** The creation time of the project. */
@JsonProperty(CREATION_TIMESTAMP_PROPERTY)
private long creationTimestamp;
/** Whether this project is in the process of being deleted. */
@JsonProperty("deleting")
private boolean deleting = false;
/** Whether this project is in the process of being re-analyzed. */
@JsonProperty("reanalyzing")
private boolean reanalyzing = false;
/** Whether data is being copied into this project. */
@JsonProperty("copyingData")
private boolean copyingData = false;
public ProjectInfo(String name, List publicIds, InternalProjectId parentProjectId,
String description, long creationTimestamp) {
this(InternalProjectId.create(), name, publicIds, parentProjectId, description, creationTimestamp);
}
@JsonCreator
public ProjectInfo(@JsonProperty(INTERNAL_ID_PROPERTY) @Nullable InternalProjectId internalId,
@JsonProperty(NAME_PROPERTY) String name,
@JsonProperty(PUBLIC_IDS_PROPERTY) List publicIds,
@JsonProperty(PARENT_PROJECT_ID_PROPERTY) @Nullable InternalProjectId parentProjectId,
@JsonProperty(DESCRIPTION_PROPERTY) String description,
@JsonProperty(CREATION_TIMESTAMP_PROPERTY) long creationTimestamp) {
if (internalId != null) {
this.internalId = internalId;
} else {
this.internalId = InternalProjectId.create();
}
setPublicIds(publicIds);
this.name = name;
this.parentProjectId = parentProjectId;
this.description = description;
this.creationTimestamp = creationTimestamp;
}
/** Returns the project id. */
public InternalProjectId getInternalId() {
return internalId;
}
/**
* Returns the primary alias if present. Otherwise the {@link #internalId} is
* returned. Can be used in case the REST API or UI code expects the alias to
* shadow the id.
*/
public PublicProjectId getPrimaryPublicId() {
return publicIds.get(0);
}
/** Returns {@link #publicIds}. */
public UnmodifiableList getPublicIds() {
return CollectionUtils.asUnmodifiable(publicIds);
}
/** Sets {@link #publicIds}. */
public void setPublicIds(List publicIds) {
Preconditions.checkState(!CollectionUtils.isNullOrEmpty(publicIds) || isDeleting(),
"At least one public ID needs to be provided.");
this.publicIds = publicIds;
}
/** @see #parentProjectId */
public Optional getParentProjectId() {
return Optional.ofNullable(parentProjectId);
}
/** Returns {@link #name}. */
public String getName() {
return name;
}
/** Sets {@link #name}. */
public void setName(String name) {
this.name = name;
}
/** Returns {@link #description}. */
public String getDescription() {
return description;
}
/** Sets {@link #description}. */
public void setDescription(String description) {
this.description = description;
}
/** Returns {@link #creationTimestamp}. */
public long getCreationTimestamp() {
return creationTimestamp;
}
/** Sets the creation timestamp. */
public void setCreationTimestamp(long creationTimestamp) {
this.creationTimestamp = creationTimestamp;
}
/** Returns {@link #deleting}. */
public boolean isDeleting() {
return deleting;
}
/** @see #isReanalyzing */
public boolean isReanalyzing() {
return reanalyzing;
}
/**
* Returns whether this project is currently in the process of either being
* reanalyzed or deleted.
*/
public boolean isDeletingOrReanalyzing() {
return isDeleting() || isReanalyzing();
}
/** @see #deleting */
public void setDeleting(boolean deleting) {
this.deleting = deleting;
}
/** @see #isReanalyzing */
public void setReanalyzing(boolean reanalyzing) {
this.reanalyzing = reanalyzing;
}
/** @see #copyingData */
public void setCopyingData(boolean copyingData) {
this.copyingData = copyingData;
}
/** @see #copyingData */
public boolean isCopyingData() {
return copyingData;
}
@Override
public String toString() {
return "ProjectInfo: " + "internalId='" + internalId + '\'' + ", publicIds='" + publicIds + '\''
+ ", parentProjectId='" + parentProjectId + '\'' + ", name='" + name + '\'' + ", deleting: " + deleting
+ ", reanalyzing: " + reanalyzing;
}
@Override
public boolean equals(Object other) {
if (this == other) {
return true;
}
if (other == null || getClass() != other.getClass()) {
return false;
}
ProjectInfo that = (ProjectInfo) other;
return Objects.equals(internalId, that.internalId) && Objects.equals(publicIds, that.publicIds)
&& Objects.equals(name, that.name);
}
@Override
public int hashCode() {
return Objects.hash(internalId, publicIds, name);
}
@Override
public int compareTo(ProjectInfo other) {
if (other == null) {
return -1;
}
if (this.getPrimaryPublicId().toString().equals(MAINTENANCE_PROJECT_INTERNAL_NAME)
&& !other.getPrimaryPublicId().toString().equals(MAINTENANCE_PROJECT_INTERNAL_NAME)) {
return -1;
}
if (other.getPrimaryPublicId().toString().equals(MAINTENANCE_PROJECT_INTERNAL_NAME)
&& !this.getPrimaryPublicId().toString().equals(MAINTENANCE_PROJECT_INTERNAL_NAME)) {
return 1;
}
return Comparator.comparing(ProjectInfo::getName, Comparator.nullsLast(Comparator.naturalOrder()))
.thenComparing(ProjectInfo::getPrimaryPublicId, Comparator.nullsLast(Comparator.naturalOrder()))
.compare(this, other);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy