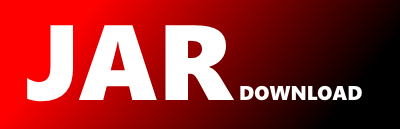
com.teamscale.commons.toml.TeamscaleIntegrationConfigurationFileV1 Maven / Gradle / Ivy
package com.teamscale.commons.toml;
import java.io.File;
import org.conqat.lib.commons.collections.Pair;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonProperty;
/**
* Representation of a configuration file or a hierarchy of configuration files.
* The represented file if formatted according to the format described in
* architecture-decisions/0016-ide-config-format.md (i.e., version 1.0 of that
* config-file format).
*
* The field names in this class are defined by the format.
*/
/* package */ class TeamscaleIntegrationConfigurationFileV1 {
/**
* The config-file format version.
*/
private final String version;
/**
* Whether this file is the "root" of the config hierarchy or the parser should
* continue searching upwards.
*/
private final boolean root;
/**
* {@link TeamscaleIntegrationConfiguration#server}
*/
private final Server server;
/**
* {@link TeamscaleIntegrationConfiguration#project}
*/
private final Project project;
/* package */ TeamscaleIntegrationConfigurationFileV1(@JsonProperty("version") String version,
@JsonProperty(value = "root", required = false, defaultValue = "false") boolean root,
@JsonProperty("server") Server server, @JsonProperty("project") Project project) {
if (version == null) {
// if no version is specified, "1.0" is the default (only in the 1.0 file
// version, in all others the version string must be non-null)
this.version = "1.0";
} else {
this.version = version;
}
this.root = root;
this.server = server;
this.project = project;
}
/**
* Converts this version 1.0 config file to the internal
* #TeamscaleIntegrationConfiguration format (where we can add format extensions
* and change field names).
*/
/* package */ TeamscaleIntegrationConfiguration convertToInternalFormat(File configFile) {
TeamscaleIntegrationConfiguration.Server newServer = null;
if (server != null) {
newServer = server.convertToInternalFormat();
}
TeamscaleIntegrationConfiguration.Project newProject = null;
if (project != null) {
newProject = project.convertToInternalFormat(configFile);
}
return new TeamscaleIntegrationConfiguration(version, root, newServer, newProject);
}
/**
* {@link TeamscaleIntegrationConfiguration.Server}
*/
/* package */ static class Server {
private final String url;
@JsonCreator
/* package */ Server(@JsonProperty("url") String url) {
this.url = url;
}
/**
* Converts this version 1.0 config file to the internal
* #TeamscaleIntegrationConfiguration format (where we can add format extensions
* and change field names).
*/
/* package */ TeamscaleIntegrationConfiguration.Server convertToInternalFormat() {
return new TeamscaleIntegrationConfiguration.Server(url);
}
}
/**
* {@link TeamscaleIntegrationConfiguration.Project}
*/
/* package */ static class Project {
private final String id;
private final String branch;
private final String path;
@JsonCreator
/* package */ Project(@JsonProperty("id") String id, @JsonProperty("branch") String branch,
@JsonProperty("path") String path) {
this.id = id;
this.branch = branch;
this.path = path;
}
/**
* Converts this version 1.0 config file to the internal
* #TeamscaleIntegrationConfiguration format (where we can to extensions and
* change field names).
*/
/* package */ TeamscaleIntegrationConfiguration.Project convertToInternalFormat(File configFile) {
// create a mapping from the parent dir of the current config file (i.e., path
// on the current machine) to the configured path in Teamscale
Pair mappedPath = null;
if (path != null) {
mappedPath = Pair.createPair(configFile.getParentFile(), path);
}
return new TeamscaleIntegrationConfiguration.Project(id, branch, mappedPath);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy