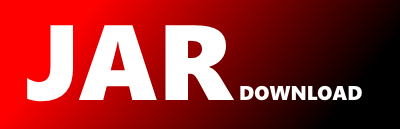
org.conqat.engine.service.shared.data.Legacy64BranchesInfo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of teamscale-commons Show documentation
Show all versions of teamscale-commons Show documentation
Provides common DTOs for Teamscale
/*
* Copyright (c) CQSE GmbH
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.conqat.engine.service.shared.data;
import java.util.ArrayList;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.conqat.engine.index.shared.GitRefUtils;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.teamscale.commons.lang.ToStringHelpers;
/**
* Transport class for the branches of a project.
*
* This class is used as DTO during communication with IDE clients via
* {@link com.teamscale.ide.commons.client.IIdeServiceClient}, special care has
* to be taken when changing its signature!
*
* @deprecated Since Teamscale 6.4.0
*/
@Deprecated
public class Legacy64BranchesInfo {
/**
* Name of the default branch. This is called "main" branch for history reasons
* and we decided to keep as is, as we otherwise need migrations and new
* versions for the IDE plugins.
*/
@JsonProperty("mainBranchName")
private final String mainBranchName;
/**
* The names of all available active branches, including the default branch, but
* excluding deleted and anonymous branches.
*/
@JsonProperty("branchNames")
private final List branchNames = new ArrayList<>();
/**
* The names of deleted/inactive branches.
*
* @since 5.0
*/
@JsonProperty("deletedBranches")
private final List deletedBranches = new ArrayList<>();
/**
* A list of anonymous branches or an empty list if non exist.
*
* @since 5.0
*/
@JsonProperty("anonymousBranches")
private final List anonymousBranches = new ArrayList<>();
/**
* This maps aliases to branch names. The aliases are displayed in the UI,
* instead of the branch name.
*/
@JsonProperty("aliases")
private final Map aliases = new HashMap<>();
/**
* The list of branches last viewed by the current user.
*
* @since 6.3
*/
@JsonProperty("recentBranches")
private final List recentBranches = new ArrayList<>();
/** Empty constructor for serialization purposes */
public Legacy64BranchesInfo() {
this("", Collections.emptyList(), Collections.emptyList(), Collections.emptyList());
}
public Legacy64BranchesInfo(String defaultBranchName, List branchNames, List deletedBranches,
List recentBranches) {
this.mainBranchName = defaultBranchName;
copyNonAnonymousBranches(branchNames, this.branchNames);
copyNonAnonymousBranches(deletedBranches, this.deletedBranches);
this.recentBranches.addAll(recentBranches);
}
/**
* Copies all non-anonymous branches from source to target. Anonymous branches
* are stored in {@link #anonymousBranches}.
*/
private void copyNonAnonymousBranches(List sourceBranches, List targetBranches) {
for (String branchName : sourceBranches) {
if (GitRefUtils.isAnonymousBranchName(branchName)) {
anonymousBranches.add(branchName);
} else {
targetBranches.add(branchName);
}
}
}
/** @see #aliases */
public void addBranchAlias(String branch, String alias) {
aliases.put(branch, alias);
}
/** @see #aliases */
public Map getAliases() {
return Collections.unmodifiableMap(aliases);
}
/** @see #mainBranchName */
public String getDefaultBranchName() {
return mainBranchName;
}
/**
* The names of all active branches, including the default branch but excluding
* deleted or anonymous branches.
*/
public List getActiveBranchNames() {
return branchNames;
}
/** Returns the union of all branch names. */
public List getAllBranchNames() {
List allBranchNames = new ArrayList<>(branchNames);
// prevent NPE for deserialized objects (https://jira.cqse.eu/browse/TS-18489)
if (deletedBranches != null) {
allBranchNames.addAll(deletedBranches);
}
if (anonymousBranches != null) {
allBranchNames.addAll(anonymousBranches);
}
return allBranchNames;
}
@Override
public String toString() {
return ToStringHelpers.toReflectiveStringHelper(this).toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy