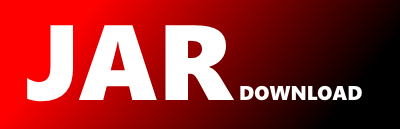
org.conqat.engine.service.shared.data.PreCommitServerLimits Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of teamscale-commons Show documentation
Show all versions of teamscale-commons Show documentation
Provides common DTOs for Teamscale
/*
* Copyright (c) CQSE GmbH
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.conqat.engine.service.shared.data;
import java.io.Serializable;
import java.util.Objects;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.teamscale.commons.lang.ToStringHelpers;
/**
* DTO containing all required information of the server concerning server
* limits
*
* This class is used as DTO during communication with IDE clients via
* {@link com.teamscale.ide.commons.client.IIdeServiceClient}, special care has
* to be taken when changing its signature!
*/
public final class PreCommitServerLimits implements Serializable {
private static final long serialVersionUID = 1;
/** No limits w.r.t. time or files. */
public static final PreCommitServerLimits NO_LIMITS = new PreCommitServerLimits(Integer.MAX_VALUE,
Integer.MAX_VALUE, Integer.MAX_VALUE);
/** The name of the JSON property name for {@link #timeLimitInSeconds}. */
private static final String TIME_LIMIT_IN_SECONDS_PROPERTY = "timeLimitInSeconds";
/** The name of the JSON property name for {@link #fileSizeLimitInBytes}. */
private static final String FILE_SIZE_LIMIT_IN_BYTES_PROPERTY = "fileSizeLimitInBytes";
/** The name of the JSON property name for {@link #fileCountLimit}. */
private static final String FILE_COUNT_LIMIT_PROPERTY = "fileCountLimit";
@JsonProperty(TIME_LIMIT_IN_SECONDS_PROPERTY)
private final int timeLimitInSeconds;
@JsonProperty(FILE_SIZE_LIMIT_IN_BYTES_PROPERTY)
private final long fileSizeLimitInBytes;
@JsonProperty(FILE_COUNT_LIMIT_PROPERTY)
private final int fileCountLimit;
@JsonCreator
public PreCommitServerLimits(@JsonProperty(FILE_COUNT_LIMIT_PROPERTY) int fileCountLimit,
@JsonProperty(FILE_SIZE_LIMIT_IN_BYTES_PROPERTY) long fileSizeLimitInBytes,
@JsonProperty(TIME_LIMIT_IN_SECONDS_PROPERTY) int timeLimitInSeconds) {
this.fileCountLimit = fileCountLimit;
this.fileSizeLimitInBytes = fileSizeLimitInBytes;
this.timeLimitInSeconds = timeLimitInSeconds;
}
public int getTimeLimitInSeconds() {
return timeLimitInSeconds;
}
public long getFileSizeLimitInBytes() {
return fileSizeLimitInBytes;
}
public int getFileCountLimit() {
return fileCountLimit;
}
@Override
public boolean equals(Object other) {
if (this == other) {
return true;
}
if (other == null) {
return false;
}
if (getClass() != other.getClass()) {
return false;
}
PreCommitServerLimits that = (PreCommitServerLimits) other;
return Objects.equals(timeLimitInSeconds, that.timeLimitInSeconds)
&& Objects.equals(fileSizeLimitInBytes, that.fileSizeLimitInBytes)
&& Objects.equals(fileCountLimit, that.fileCountLimit);
}
@Override
public int hashCode() {
return Objects.hash(timeLimitInSeconds, fileSizeLimitInBytes, fileCountLimit);
}
@Override
public String toString() {
return ToStringHelpers.toReflectiveStringHelper(this).toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy