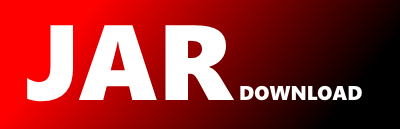
org.conqat.engine.sourcecode.coverage.ProbeCoverageInfo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of teamscale-commons Show documentation
Show all versions of teamscale-commons Show documentation
Provides common DTOs for Teamscale
/*
* Copyright (c) CQSE GmbH
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.conqat.engine.sourcecode.coverage;
import org.conqat.lib.commons.test.IndexValueClass;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.Collection;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import java.util.stream.Collectors;
import org.conqat.lib.commons.collections.CollectionUtils;
import org.conqat.lib.commons.collections.UnmodifiableList;
import org.conqat.lib.commons.js_export.ExportAsType;
import org.conqat.lib.commons.js_export.ExportToTypeScript;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonProperty;
/**
* Holds coverage information for a file represented as "probes". See
* {@link CoverageProbeBase} for a description of probes.
*
* We use this object both to represent covered and coverable information. The
* flag {@link #uploaded} differs between the two cases.
*/
@ExportToTypeScript
@IndexValueClass(containedInBackup = true)
public class ProbeCoverageInfo implements Serializable {
private static final long serialVersionUID = 1L;
/** The name of the JSON property name for {@link #probes}. */
private static final String PROBES_PROPERTY = "probes";
/** The name of the JSON property name for {@link #uploaded}. */
private static final String UPLOADED_PROPERTY = "uploaded";
@JsonProperty(PROBES_PROPERTY)
@ExportAsType("Array")
private final ArrayList probes;
/**
* Signals whether this is coverage info uploaded externally. See also class
* comment
*/
@JsonProperty(UPLOADED_PROPERTY)
private final boolean uploaded;
public ProbeCoverageInfo(boolean uploaded) {
this.probes = new ArrayList<>();
this.uploaded = uploaded;
}
@JsonCreator
public ProbeCoverageInfo(@JsonProperty(UPLOADED_PROPERTY) boolean uploaded,
@JsonProperty(PROBES_PROPERTY) Collection probes) {
this(uploaded);
this.probes.addAll(probes);
}
/** Constructor. */
public ProbeCoverageInfo(ProbeCoverageInfo coverageProbeInfo) {
this.probes = new ArrayList<>(coverageProbeInfo.probes);
this.uploaded = coverageProbeInfo.uploaded;
}
/** Adds the given probe */
public void addProbe(CoverageProbeBase probe) {
probes.add(probe);
}
public UnmodifiableList getProbes() {
return CollectionUtils.asUnmodifiable(probes);
}
/** Overwrites the probes of this coverage info. */
public void setProbes(List probes) {
this.probes.clear();
this.probes.addAll(probes);
}
/** @see #uploaded */
public boolean isUploaded() {
return uploaded;
}
/** Returns the number of coverable entities of this probe coverage */
public int getCoverableCount() {
int coverableCount = 0;
for (CoverageProbeBase probe : probes) {
coverableCount += probe.getCoverableCount();
}
return coverableCount;
}
/** Returns the number of covered entities of this probe coverage */
public int getCoveredCount() {
int coveredCount = 0;
for (CoverageProbeBase probe : probes) {
coveredCount += probe.getCoveredCount();
}
return coveredCount;
}
/**
* Merges the results from the other coverage into this one. This is done by
* comparing the content of {@link #probes} where the same type and line is used
* and merging those probes. Note that probe merging is non-trivial and hence
* error-prone, unless in very simple cases.
*
* This operation is "almost" symmetric, i.e. it does not really matter, what is
* merged into what, but the this
instance will be modified to
* store the result.
*
* @return whether the merge was safe, i.e. if this returns true
we
* are sure that the result of the merge was correct, otherwise this
* might be off.
*/
public boolean mergeWith(ProbeCoverageInfo otherCoverage) {
if (otherCoverage.probes.isEmpty()) {
return true;
}
if (probes.isEmpty()) {
probes.addAll(otherCoverage.probes);
return true;
}
return mergeProbes(otherCoverage.probes, probes);
}
/**
* Merges the given source probes into the target probes (i.e. the target
* collection will be modified).
*
* @return whether the merge was safe, i.e. if this returns true
we
* are sure that the result of the merge was correct, otherwise this
* might be off.
*/
private static boolean mergeProbes(Collection sourceProbes,
Collection targetProbes) {
Map> targetProbesByLine = targetProbes.stream()
.collect(Collectors.groupingBy(CoverageProbeBase::getLine));
boolean safe = true;
for (CoverageProbeBase sourceProbe : sourceProbes) {
List targetProbeCandidates = targetProbesByLine.get(sourceProbe.getLine());
Optional matchedProbe = findFirstMatchingProbe(sourceProbe, targetProbeCandidates);
if (matchedProbe.isPresent()) {
safe = matchedProbe.get().mergeWith(sourceProbe) && safe;
} else {
targetProbes.add(sourceProbe);
}
}
return safe;
}
private static Optional findFirstMatchingProbe(CoverageProbeBase sourceProbe,
List targetCandidates) {
if (targetCandidates == null) {
return Optional.empty();
}
return targetCandidates.stream().filter(targetProbe -> targetProbe.getClass() == sourceProbe.getClass())
.findFirst();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy