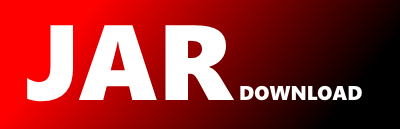
org.conqat.engine.commons.util.CommonUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of teamscale-commons Show documentation
Show all versions of teamscale-commons Show documentation
Provides common DTOs for Teamscale
/*
* Copyright (c) CQSE GmbH
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.conqat.engine.commons.util;
import java.nio.charset.Charset;
import java.text.ParseException;
import java.text.SimpleDateFormat;
import java.util.Arrays;
import java.util.Collection;
import java.util.Date;
import java.util.Objects;
import java.util.regex.Pattern;
import java.util.regex.PatternSyntaxException;
import org.conqat.engine.core.core.ConQATException;
/**
* Collection of utility methods.
*/
public class CommonUtils {
/** Default date format. */
public static final String DEFAULT_DATE_FORMAT_PATTERN = "yyyy-MM-dd";
/**
* Create {@link SimpleDateFormat} from pattern string. In contrast to the
* constructor of {@link SimpleDateFormat} this raises a {@link ConQATException}
* for invalid patterns.
*/
public static SimpleDateFormat createDateFormat(String pattern) throws ConQATException {
try {
return new SimpleDateFormat(pattern);
} catch (IllegalArgumentException e) {
throw new ConQATException("Illegal date pattern: " + pattern, e);
}
}
/**
* This method parses a date string using the specified format pattern. See
* {@link SimpleDateFormat} for pattern syntax.
*
* @throws ConQATException
* if an illegal format string was supplied or the date string does
* not match the given format.
*/
public static Date parseDate(String dateString, String formatString) throws ConQATException {
SimpleDateFormat format = createDateFormat(formatString);
try {
return format.parse(dateString);
} catch (ParseException e) {
throw new ConQATException("Illegal date format for '" + dateString + "'.", e);
}
}
/**
* Wraps {@link Pattern#compile(String)} to produce {@link ConQATException}s
* instead of {@link PatternSyntaxException}s.
*/
public static Pattern compilePattern(String regex) throws ConQATException {
try {
return Pattern.compile(regex);
} catch (PatternSyntaxException e) {
throw new ConQATException("Illegal regular expression: ", e);
}
}
/**
* Get an encoding for the specified name. This throws a {@link ConQATException}
* if the encoding is not supported.
*/
public static Charset obtainEncoding(String encodingName) throws ConQATException {
if (!Charset.isSupported(encodingName)) {
throw new ConQATException("Unsupported encoding: " + encodingName);
}
return Charset.forName(encodingName);
}
/** Check if obj is equal to one of the provided objects. */
@SafeVarargs
public static boolean isOneOf(T obj, T... others) {
Objects.requireNonNull(others);
return isOneOf(obj, Arrays.asList(others));
}
/** Check if obj is equal to one of the provided objects. */
public static boolean isOneOf(T obj, Collection others) {
Objects.requireNonNull(others);
for (Object otherObj : others) {
if (Objects.equals(obj, otherObj)) {
return true;
}
}
return false;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy