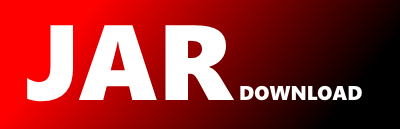
org.conqat.engine.sourcecode.coverage.TestUniformPathUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of teamscale-commons Show documentation
Show all versions of teamscale-commons Show documentation
Provides common DTOs for Teamscale
/*
* Copyright (c) CQSE GmbH
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.conqat.engine.sourcecode.coverage;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import org.conqat.engine.resource.util.UniformPathUtils;
import org.conqat.lib.commons.string.StringUtils;
import org.conqat.lib.commons.uniformpath.RelativeUniformPath;
import org.conqat.lib.commons.uniformpath.UniformPath;
import org.conqat.lib.commons.uniformpath.UniformPathCompatibilityUtil;
import com.google.common.base.Preconditions;
/**
* Utility methods for converting test execution paths to uniform paths and the
* other way around.
*/
public class TestUniformPathUtils {
/** Symbol that starts extending the test with parameters */
public static final String TEST_PARAMETERIZATION_START = "[";
/** Symbol that stops extending the test with parameters */
public static final String TEST_PARAMETERIZATION_END = "]";
/** Symbol that starts the test method's call signature */
public static final String TEST_SIGNATURE_START = "(";
/** Asserts that the given path is non-null and has a test execution prefix. */
public static void assertIsTestExecutionPath(String uniformPath) {
Preconditions.checkArgument(uniformPath != null);
Preconditions.checkArgument(
uniformPath.startsWith(UniformPath.EType.TEST_EXECUTION.getPrefix() + UniformPathUtils.SEPARATOR),
"Test execution path " + uniformPath + " was expected to start with -test-execution-!");
}
/**
* Asserts that the given path is non-null and has a test execution or execution
* unit prefix.
*/
public static void assertIsCoverageUnitPath(String uniformPath) {
Preconditions.checkArgument(uniformPath != null);
Preconditions.checkArgument(
uniformPath.startsWith(UniformPath.EType.TEST_EXECUTION.getPrefix() + UniformPathUtils.SEPARATOR)
|| uniformPath
.startsWith(UniformPath.EType.EXECUTION_UNIT.getPrefix() + UniformPathUtils.SEPARATOR),
"Coverage unit path " + uniformPath
+ " was expected to start with -test-execution- or -execution-unit-!");
}
/**
* Converts the given test execution path string (as specified in a test
* execution report) to a uniform path considering that the test name may
* contain parameters. Parameters are detected as a suffix in brackets as e.g.
* path/to/test[some/parameter]. The parameter is treated as a single segment
* together with the test name, i.e. test[some/parameter] and any slashes in
* there will be escaped.
*/
public static UniformPath escapeAndConvertToTestExecutionUniformPath(String testUniformPath) {
Preconditions.checkArgument(!testUniformPath.startsWith(UniformPath.EType.TEST_EXECUTION.getPrefix()));
List testPathSegments = getTestPathSegments(testUniformPath);
return UniformPath.of(UniformPath.EType.TEST_EXECUTION, testPathSegments);
}
/**
* Converts the given test id string to a uniform path considering that the test
* name may contain parameters. Parameters are detected as a suffix in brackets
* as e.g. path/to/test[some/parameter]. The parameter is treated as a single
* segment together with the test name, i.e. test[some/parameter] and any
* slashes in there will be escaped.
*/
public static UniformPath convertToExecutionUnitUniformPath(String executableUnitPath) {
Preconditions.checkArgument(!executableUnitPath.startsWith(UniformPath.EType.EXECUTION_UNIT.getPrefix()));
RelativeUniformPath relativeUniformPath = UniformPathCompatibilityUtil.convertRelative(executableUnitPath);
return UniformPath.of(UniformPath.EType.EXECUTION_UNIT).resolve(relativeUniformPath);
}
/**
* Returns a list of escaped test path segments. Splitting happens at the last
* slash that is not contained in a pair of brackets to account for JUnit-like
* parameterized tests.
*/
private static List getTestPathSegments(String testUniformPath) {
List segments = Arrays.asList(UniformPathUtils.splitPath(testUniformPath));
for (int i = 0; i < segments.size(); i++) {
if (segments.get(i).contains(TEST_PARAMETERIZATION_START) || segments.get(i).contains("(")
|| i == segments.size() - 1) {
String testName = StringUtils.concat(segments.subList(i, segments.size()), UniformPathUtils.SEPARATOR);
List testSegments = new ArrayList<>(segments.subList(0, i));
testSegments.add(UniformPath.escapeSegment(testName));
return testSegments;
}
}
return Collections.singletonList(UniformPath.escapeSegment(testUniformPath));
}
/**
* Strips away the -test-execution-/ or -execution-unit-/ prefix of a test
* execution uniform path and removes escaping so that the result matches the
* test name the test runner gave us.
*/
public static String convertCoverageUnitPathToTestName(String uniformPath) {
assertIsCoverageUnitPath(uniformPath);
String testIdWithoutPrefix = StringUtils.stripPrefix(uniformPath,
UniformPath.EType.TEST_EXECUTION.getPrefix() + UniformPathUtils.SEPARATOR);
testIdWithoutPrefix = StringUtils.stripPrefix(testIdWithoutPrefix,
UniformPath.EType.EXECUTION_UNIT.getPrefix() + UniformPathUtils.SEPARATOR);
return UniformPath.unescapeSegment(testIdWithoutPrefix);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy