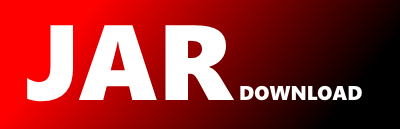
com.teamscale.config.toml.TestTeamscaleTomlV1Builder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of teamscale-config-test-fixtures Show documentation
Show all versions of teamscale-config-test-fixtures Show documentation
Support for the common .teamscale.toml config format
package com.teamscale.config.toml;
import java.net.URI;
import java.text.ParseException;
import com.teamscale.config.path.CodePath;
/**
* Builds {@link TeamscaleTomlV1} objects for test purposes.
*/
public class TestTeamscaleTomlV1Builder {
private String version;
private boolean root;
private URI serverUrl;
private String projectId;
private String projectBranch;
private CodePath projectPath;
/**
* @return a builder creating an object representing an {@code .teamscale.toml}
* configuration file.
*/
public static TestTeamscaleTomlV1Builder emptyTeamscaleToml() {
return new TestTeamscaleTomlV1Builder();
}
/**
* Ensures that the {@link TeamscaleTomlV1} is {@linkplain #build() built} with
* the given {@linkplain TeamscaleTomlV1#getVersion() version}.
*/
public TestTeamscaleTomlV1Builder withVersion(String version) {
this.version = version;
return this;
}
/**
* Ensures that the {@link TeamscaleTomlV1} is {@linkplain #build() built} with
* the given {@linkplain TeamscaleTomlV1#isRoot() root flag}.
*/
public TestTeamscaleTomlV1Builder withRootFlag(boolean root) {
this.root = root;
return this;
}
/**
* Ensures that the {@link TeamscaleTomlV1} is {@linkplain #build() built} with
* the given {@linkplain TeamscaleTomlV1.Server#getUrl() server URL}.
*/
public TestTeamscaleTomlV1Builder withServerUrl(URI serverUrl) {
this.serverUrl = serverUrl;
return this;
}
/**
* Ensures that the {@link TeamscaleTomlV1} is {@linkplain #build() built} with
* the given {@linkplain TeamscaleTomlV1.Server#getUrl() server URL}.
*/
public TestTeamscaleTomlV1Builder withServerUrl(String serverUrl) {
return withServerUrl(URI.create(serverUrl));
}
/**
* Ensures that the {@link TeamscaleTomlV1} is {@linkplain #build() built} with
* the given {@linkplain TeamscaleTomlV1.Project#getId() project ID}.
*/
public TestTeamscaleTomlV1Builder withProjectId(String projectId) {
this.projectId = projectId;
return this;
}
/**
* Ensures that the {@link TeamscaleTomlV1} is {@linkplain #build() built} with
* the given {@linkplain TeamscaleTomlV1.Project#getBranch() project branch}.
*/
public TestTeamscaleTomlV1Builder withProjectBranch(String projectBranch) {
this.projectBranch = projectBranch;
return this;
}
/**
* Ensures that the {@link TeamscaleTomlV1} is {@linkplain #build() built} with
* the given {@linkplain TeamscaleTomlV1.Project#getPath() project path}.
*/
public TestTeamscaleTomlV1Builder withProjectPath(CodePath projectPath) {
this.projectPath = projectPath;
return this;
}
/**
* Ensures that the {@link TeamscaleTomlV1} is {@linkplain #build() built} with
* the given {@linkplain TeamscaleTomlV1.Project#getPath() project path}.
*/
public TestTeamscaleTomlV1Builder withProjectPath(String projectPath) {
try {
return withProjectPath(CodePath.parse(projectPath));
} catch (ParseException e) {
throw new IllegalArgumentException(e);
}
}
/**
* Builds the {@link TeamscaleTomlV1} as configured.
*/
public TeamscaleTomlV1 build() {
TeamscaleTomlV1.Server server;
if (serverUrl != null) {
server = new TeamscaleTomlV1.Server(serverUrl);
} else {
server = null;
}
TeamscaleTomlV1.Project project = null;
if (projectId != null || projectBranch != null || projectPath != null) {
project = new TeamscaleTomlV1.Project(projectId, projectBranch, projectPath);
} else {
project = null;
}
return new TeamscaleTomlV1(version, root, server, project);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy