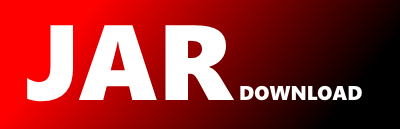
com.techempower.js.legacy.Visitors Maven / Gradle / Ivy
/*******************************************************************************
* Copyright (c) 2018, TechEmpower, Inc.
* All rights reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions are met:
* * Redistributions of source code must retain the above copyright
* notice, this list of conditions and the following disclaimer.
* * Redistributions in binary form must reproduce the above copyright
* notice, this list of conditions and the following disclaimer in the
* documentation and/or other materials provided with the distribution.
* * Neither the name TechEmpower, Inc. nor the names of its
* contributors may be used to endorse or promote products derived from
* this software without specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND
* ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED
* WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE
* DISCLAIMED. IN NO EVENT SHALL TECHEMPOWER, INC. BE LIABLE FOR ANY DIRECT,
* INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING,
* BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE,
* DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY
* OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING
* NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE,
* EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
*******************************************************************************/
package com.techempower.js.legacy;
import com.techempower.js.*;
/**
* Provides useful {@link Visitor} and {@link VisitorFactory} implementations
* for use with a {@link LegacyJavaScriptWriter}.
*
* As an example, imagine you want the application to render {@code User}
* objects in JSON like so:
*
*
* {
* "id": <the user's unique id>,
* "email": <the user's email address>
* }
*
*
* The object responsible for translating {@code User}s to that structure is
* a {@code VisitorFactory}. Here are a few different ways of creating
* that visitor factory:
*
*
* -
* Using a reflection-based approach. This works when all of the properties
* in the output exist as getter fields on the User class.
*
* VisitorFactory<User> userVisitors = Visitors.forClass(
* User.class,
* "id", "getId",
* "email", "getUserEmail");
*
*
* -
* Using the Visitors.map method. This is more flexible than the
* reflection-based approach because not every property must exist as a
* getter field on the User class. It also avoids having method names
* as strings in your code.
*
* VisitorFactory<User> userVisitors = new VisitorFactory<User>() {
* public Visitor visitor(User user)
* {
* return Visitors.map(
* "id", user.getId(),
* "email", user.getUserEmail());
* }
* };
*
*
* -
* Converting the users into one of the standard collection types--map,
* array, list, etc.--and using the predefined visitor factory for that
* collection type. (In this particular case, the Visitors.map approach
* would be less verbose and more efficient.)
*
* VisitorFactory<User> userVisitors = new VisitorFactory<User>() {
* public Visitor visitor(User user)
* {
* Map<String, Object> map = new HashMap<String, Object>();
* map.put("id", user.getId());
* map.put("email", user.getUserEmail());
* return Visitors.forMaps().visitor(map);
* }
* };
*
*
*
*/
public final class Visitors
{
private Visitors() {}
/**
* Produces visitors for arrays.
*/
public static VisitorFactory
© 2015 - 2025 Weber Informatics LLC | Privacy Policy