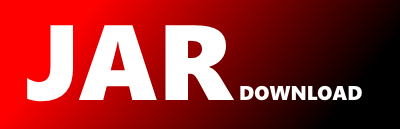
com.tectonica.jonix.onix3.Collection Maven / Gradle / Ivy
/*
* Copyright (C) 2012-2024 Zach Melamed
*
* Latest version available online at https://github.com/zach-m/jonix
* Contact me at [email protected]
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.tectonica.jonix.onix3;
import com.tectonica.jonix.common.JPU;
import com.tectonica.jonix.common.ListOfOnixComposite;
import com.tectonica.jonix.common.ListOfOnixDataCompositeWithKey;
import com.tectonica.jonix.common.ListOfOnixElement;
import com.tectonica.jonix.common.OnixComposite.OnixSuperComposite;
import com.tectonica.jonix.common.codelist.CollectionIdentifierTypes;
import com.tectonica.jonix.common.codelist.CollectionSequenceTypes;
import com.tectonica.jonix.common.codelist.RecordSourceTypes;
import com.tectonica.jonix.common.struct.JonixCollectionIdentifier;
import com.tectonica.jonix.common.struct.JonixCollectionSequence;
import java.io.Serializable;
import java.util.function.Consumer;
/*
* NOTE: THIS IS AN AUTO-GENERATED FILE, DO NOT EDIT MANUALLY
*/
/**
* Collection composite
*
* An optional group of data elements which carry attributes of a collection of which the product is part. The composite
* is repeatable, to provide details when the product belongs to multiple collections.
*
*
*
* Reference name
* <Collection>
*
*
* Short tag
* <collection>
*
*
* Cardinality
* 0…n
*
*
*
* This tag may be included in the following composites:
*
* - <{@link DescriptiveDetail}>
*
*
* Possible placements within ONIX message:
*
* - {@link Product} ⯈ {@link DescriptiveDetail} ⯈ {@link Collection}
*
*/
public class Collection implements OnixSuperComposite, Serializable {
private static final long serialVersionUID = 1L;
public static final String refname = "Collection";
public static final String shortname = "collection";
/////////////////////////////////////////////////////////////////////////////////
// ATTRIBUTES
/////////////////////////////////////////////////////////////////////////////////
/**
* (type: dt.DateOrDateTime)
*/
public String datestamp;
/**
* (type: dt.NonEmptyString)
*/
public String sourcename;
public RecordSourceTypes sourcetype;
/////////////////////////////////////////////////////////////////////////////////
// CONSTRUCTION
/////////////////////////////////////////////////////////////////////////////////
private boolean initialized;
private final boolean exists;
private final org.w3c.dom.Element element;
public static final Collection EMPTY = new Collection();
public Collection() {
exists = false;
element = null;
initialized = true; // so that no further processing will be done on this intentionally-empty object
}
public Collection(org.w3c.dom.Element element) {
exists = true;
initialized = false;
this.element = element;
datestamp = JPU.getAttribute(element, "datestamp");
sourcename = JPU.getAttribute(element, "sourcename");
sourcetype = RecordSourceTypes.byCode(JPU.getAttribute(element, "sourcetype"));
}
@Override
public void _initialize() {
if (initialized) {
return;
}
initialized = true;
JPU.forElementsOf(element, e -> {
final String name = e.getNodeName();
switch (name) {
case CollectionType.refname:
case CollectionType.shortname:
collectionType = new CollectionType(e);
break;
case Contributor.refname:
case Contributor.shortname:
contributors = JPU.addToList(contributors, new Contributor(e));
break;
case SourceName.refname:
case SourceName.shortname:
sourceName = new SourceName(e);
break;
case NoContributor.refname:
case NoContributor.shortname:
noContributor = new NoContributor(e);
break;
case CollectionIdentifier.refname:
case CollectionIdentifier.shortname:
collectionIdentifiers = JPU.addToList(collectionIdentifiers, new CollectionIdentifier(e));
break;
case CollectionSequence.refname:
case CollectionSequence.shortname:
collectionSequences = JPU.addToList(collectionSequences, new CollectionSequence(e));
break;
case TitleDetail.refname:
case TitleDetail.shortname:
titleDetails = JPU.addToList(titleDetails, new TitleDetail(e));
break;
case ContributorStatement.refname:
case ContributorStatement.shortname:
contributorStatements = JPU.addToList(contributorStatements, new ContributorStatement(e));
break;
default:
break;
}
});
}
/**
* @return whether this tag (<Collection> or <collection>) is explicitly provided in the ONIX XML
*/
@Override
public boolean exists() {
return exists;
}
public void ifExists(Consumer action) {
if (exists) {
action.accept(this);
}
}
@Override
public org.w3c.dom.Element getXmlElement() {
return element;
}
/////////////////////////////////////////////////////////////////////////////////
// MEMBERS
/////////////////////////////////////////////////////////////////////////////////
private CollectionType collectionType = CollectionType.EMPTY;
/**
*
* An ONIX code indicating the type of a collection: publisher collection, ascribed collection, or unspecified.
* Mandatory in each occurrence of the <Collection> composite, and non-repeating.
*
* Jonix-Comment: this field is required
*/
public CollectionType collectionType() {
_initialize();
return collectionType;
}
private ListOfOnixComposite contributors = JPU.emptyListOfOnixComposite(Contributor.class);
/**
*
* A group of data elements which together describe a personal or corporate contributor to a collection. Optional,
* and repeatable to describe multiple contributors. The <Contributor> composite is included here for
* use only by those ONIX communities whose national practice requires contributors to be identified at collection
* level. In many countries, including the UK, USA, Canada and Spain, the required practice is to identify all
* contributors at product level in Group P.7.
*
* Jonix-Comment: this list is required to contain at least one item
*/
public ListOfOnixComposite contributors() {
_initialize();
return contributors;
}
private SourceName sourceName = SourceName.EMPTY;
/**
*
* If the <CollectionType> code indicates an ascribed collection (ie a collection which has been
* identified and described by a supply chain organization other than the publisher), this element may be used to
* carry the name of the organization responsible. Optional and non-repeating.
*
* Jonix-Comment: this field is optional
*/
public SourceName sourceName() {
_initialize();
return sourceName;
}
private NoContributor noContributor = NoContributor.EMPTY;
/**
*
* An empty element that provides a positive indication that a collection has no stated authorship. Optional and
* non-repeating. Must only be sent in a record that has no <Contributor> data in Group P.5.
*
*
* The <NoContributor/> element is provided here for use only by those ONIX communities whose national
* practice requires contributors to be identified at collection level. It should not be sent in a context
* where collection contributors are normally identified in Group P.6.
*
* Jonix-Comment: this field is optional
*/
public NoContributor noContributor() {
_initialize();
return noContributor;
}
public boolean isNoContributor() {
return (noContributor().exists());
}
private ListOfOnixDataCompositeWithKey collectionIdentifiers =
JPU.emptyListOfOnixDataCompositeWithKey(CollectionIdentifier.class);
/**
*
* A repeatable group of data elements which together specify an identifier of a bibliographic collection. The
* composite is optional, and may only repeat if two or more identifiers of different types are sent for the same
* collection. It is not permissible to have two identifiers of the same type.
*
* Jonix-Comment: this list may be empty
*/
public ListOfOnixDataCompositeWithKey
collectionIdentifiers() {
_initialize();
return collectionIdentifiers;
}
private ListOfOnixDataCompositeWithKey collectionSequences =
JPU.emptyListOfOnixDataCompositeWithKey(CollectionSequence.class);
/**
*
* An optional and repeatable group of data elements which indicates some ordinal position of a product within a
* collection. Different ordinal positions may be specified using separate repeats of the composite – for example, a
* product may be published first while also being third in narrative order within a collection.
*
* Jonix-Comment: this list may be empty
*/
public ListOfOnixDataCompositeWithKey
collectionSequences() {
_initialize();
return collectionSequences;
}
private ListOfOnixComposite titleDetails = JPU.emptyListOfOnixComposite(TitleDetail.class);
/**
*
* A group of data elements which together give the text of a collection title and specify its type. Optional, but
* the composite is required unless the only collection title is carried in full, and word-for-word, as an integral
* part of the product title in P.6, in which case it should not be repeated in P.5. The composite is
* repeatable with different title types.
*
* Jonix-Comment: this list may be empty
*/
public ListOfOnixComposite titleDetails() {
_initialize();
return titleDetails;
}
private ListOfOnixElement contributorStatements = ListOfOnixElement.empty();
/**
*
* Free text showing how the collection authorship should be described in an online display, when a standard
* concatenation of individual collection contributor elements would not give a satisfactory presentation. Optional,
* and repeatable where parallel text is provided in multiple languages. The language attribute is optional
* for a single instance of <ContributorStatement>, but must be included in each instance if
* <ContributorStatement> is repeated. When the <ContributorStatement> element is sent, the recipient
* should use it to replace all name detail sent in the <Contributor> composites within <Collection>
* for display purposes only. It does not replace the <BiographicalNote> element (or any other
* element) for individual contributors. The individual name detail must also be sent in one or more
* <Contributor> composites for indexing and retrieval purposes.
*
*
* The <ContributorStatement> element is provided here for use only by those ONIX communities whose
* national practice requires contributors to be identified at collection level. It should not be sent in a
* context where collection contributors are normally identified in Group P.6.
*
* Jonix-Comment: this list may be empty
*/
public ListOfOnixElement contributorStatements() {
_initialize();
return contributorStatements;
}
}