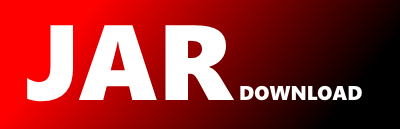
com.tectonica.jonix.onix3.NameAsSubject Maven / Gradle / Ivy
/*
* Copyright (C) 2012-2024 Zach Melamed
*
* Latest version available online at https://github.com/zach-m/jonix
* Contact me at [email protected]
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.tectonica.jonix.onix3;
import com.tectonica.jonix.common.JPU;
import com.tectonica.jonix.common.ListOfOnixComposite;
import com.tectonica.jonix.common.ListOfOnixDataComposite;
import com.tectonica.jonix.common.ListOfOnixDataCompositeWithKey;
import com.tectonica.jonix.common.OnixComposite.OnixSuperComposite;
import com.tectonica.jonix.common.codelist.NameIdentifierTypes;
import com.tectonica.jonix.common.codelist.PersonOrganizationDateRoles;
import com.tectonica.jonix.common.codelist.RecordSourceTypes;
import com.tectonica.jonix.common.struct.JonixNameIdentifier;
import com.tectonica.jonix.common.struct.JonixProfessionalAffiliation;
import com.tectonica.jonix.common.struct.JonixSubjectDate;
import java.io.Serializable;
import java.util.function.Consumer;
/*
* NOTE: THIS IS AN AUTO-GENERATED FILE, DO NOT EDIT MANUALLY
*/
/**
* Name as subject composite
*
* An optional group of data elements which together represent the name of a person or organization – real or fictional
* – that is part of the subject of a product. Repeatable in order to name multiple persons or organizations.
*
*
* Each instance of the composite must contain either:
*
*
* - one or more of the forms of representation of a person name, with or without an occurrence of the
* <NameIdentifier> composite; or
* - one or more of the forms of representation of a corporate name, with or without an occurrence of the
* <NameIdentifier> composite; or
* - an occurrence of the <NameIdentifier> composite without any accompanying name element(s).
*
*
*
* Reference name
* <NameAsSubject>
*
*
* Short tag
* <nameassubject>
*
*
* Cardinality
* 0…n
*
*
*
* This tag may be included in the following composites:
*
* - <{@link DescriptiveDetail}>
* - <{@link ContentItem}>
*
*
* Possible placements within ONIX message:
*
* - {@link Product} ⯈ {@link DescriptiveDetail} ⯈ {@link NameAsSubject}
* - {@link Product} ⯈ {@link ContentDetail} ⯈ {@link ContentItem} ⯈ {@link NameAsSubject}
*
*/
public class NameAsSubject implements OnixSuperComposite, Serializable {
private static final long serialVersionUID = 1L;
public static final String refname = "NameAsSubject";
public static final String shortname = "nameassubject";
/////////////////////////////////////////////////////////////////////////////////
// ATTRIBUTES
/////////////////////////////////////////////////////////////////////////////////
/**
* (type: dt.DateOrDateTime)
*/
public String datestamp;
/**
* (type: dt.NonEmptyString)
*/
public String sourcename;
public RecordSourceTypes sourcetype;
/////////////////////////////////////////////////////////////////////////////////
// CONSTRUCTION
/////////////////////////////////////////////////////////////////////////////////
private boolean initialized;
private final boolean exists;
private final org.w3c.dom.Element element;
public static final NameAsSubject EMPTY = new NameAsSubject();
public NameAsSubject() {
exists = false;
element = null;
initialized = true; // so that no further processing will be done on this intentionally-empty object
}
public NameAsSubject(org.w3c.dom.Element element) {
exists = true;
initialized = false;
this.element = element;
datestamp = JPU.getAttribute(element, "datestamp");
sourcename = JPU.getAttribute(element, "sourcename");
sourcetype = RecordSourceTypes.byCode(JPU.getAttribute(element, "sourcetype"));
}
@Override
public void _initialize() {
if (initialized) {
return;
}
initialized = true;
JPU.forElementsOf(element, e -> {
final String name = e.getNodeName();
switch (name) {
case PersonName.refname:
case PersonName.shortname:
personName = new PersonName(e);
break;
case KeyNames.refname:
case KeyNames.shortname:
keyNames = new KeyNames(e);
break;
case CorporateName.refname:
case CorporateName.shortname:
corporateName = new CorporateName(e);
break;
case NameIdentifier.refname:
case NameIdentifier.shortname:
nameIdentifiers = JPU.addToList(nameIdentifiers, new NameIdentifier(e));
break;
case NameType.refname:
case NameType.shortname:
nameType = new NameType(e);
break;
case PersonNameInverted.refname:
case PersonNameInverted.shortname:
personNameInverted = new PersonNameInverted(e);
break;
case TitlesBeforeNames.refname:
case TitlesBeforeNames.shortname:
titlesBeforeNames = new TitlesBeforeNames(e);
break;
case NamesBeforeKey.refname:
case NamesBeforeKey.shortname:
namesBeforeKey = new NamesBeforeKey(e);
break;
case PrefixToKey.refname:
case PrefixToKey.shortname:
prefixToKey = new PrefixToKey(e);
break;
case NamesAfterKey.refname:
case NamesAfterKey.shortname:
namesAfterKey = new NamesAfterKey(e);
break;
case SuffixToKey.refname:
case SuffixToKey.shortname:
suffixToKey = new SuffixToKey(e);
break;
case LettersAfterNames.refname:
case LettersAfterNames.shortname:
lettersAfterNames = new LettersAfterNames(e);
break;
case TitlesAfterNames.refname:
case TitlesAfterNames.shortname:
titlesAfterNames = new TitlesAfterNames(e);
break;
case Gender.refname:
case Gender.shortname:
gender = new Gender(e);
break;
case CorporateNameInverted.refname:
case CorporateNameInverted.shortname:
corporateNameInverted = new CorporateNameInverted(e);
break;
case AlternativeName.refname:
case AlternativeName.shortname:
alternativeNames = JPU.addToList(alternativeNames, new AlternativeName(e));
break;
case SubjectDate.refname:
case SubjectDate.shortname:
subjectDates = JPU.addToList(subjectDates, new SubjectDate(e));
break;
case ProfessionalAffiliation.refname:
case ProfessionalAffiliation.shortname:
professionalAffiliations = JPU.addToList(professionalAffiliations, new ProfessionalAffiliation(e));
break;
default:
break;
}
});
}
/**
* @return whether this tag (<NameAsSubject> or <nameassubject>) is explicitly provided in the ONIX XML
*/
@Override
public boolean exists() {
return exists;
}
public void ifExists(Consumer action) {
if (exists) {
action.accept(this);
}
}
@Override
public org.w3c.dom.Element getXmlElement() {
return element;
}
/////////////////////////////////////////////////////////////////////////////////
// MEMBERS
/////////////////////////////////////////////////////////////////////////////////
private PersonName personName = PersonName.EMPTY;
/**
*
* The name of a person who contributed to the creation of the product, unstructured, and presented in normal order.
* Optional and non-repeating: see Group P.7 introductory text for valid options.
*
* Jonix-Comment: this field is required
*/
public PersonName personName() {
_initialize();
return personName;
}
private KeyNames keyNames = KeyNames.EMPTY;
/**
*
* The fourth part of a structured name of a person who contributed to the creation of the product: key name(s),
* ie the name elements normally used to open an entry in an alphabetical list, eg ‘Smith’ or ‘Garcia
* Marquez’ or ‘Madonna’ or ‘Francis de Sales’ (in Saint Francis de Sales). Non-repeating. Required if name part
* elements P.7.11 to P.7.18 are used.
*
* Jonix-Comment: this field is required
*/
public KeyNames keyNames() {
_initialize();
return keyNames;
}
private CorporateName corporateName = CorporateName.EMPTY;
/**
*
* The name of a corporate body which contributed to the creation of the product, unstructured. Optional and
* non-repeating: see Group P.7 introductory text for valid options.
*
* Jonix-Comment: this field is required
*/
public CorporateName corporateName() {
_initialize();
return corporateName;
}
private ListOfOnixDataCompositeWithKey nameIdentifiers =
JPU.emptyListOfOnixDataCompositeWithKey(NameIdentifier.class);
/**
* Jonix-Comment: this list is required to contain at least one item
*/
public ListOfOnixDataCompositeWithKey nameIdentifiers() {
_initialize();
return nameIdentifiers;
}
private NameType nameType = NameType.EMPTY;
/**
*
* An ONIX code indicating the type of a primary name. Optional, and non-repeating. If omitted, the default is
* ‘unspecified’.
*
* Jonix-Comment: this field is optional
*/
public NameType nameType() {
_initialize();
return nameType;
}
private PersonNameInverted personNameInverted = PersonNameInverted.EMPTY;
/**
*
* The name of a person who contributed to the creation of the product, presented with the element used for
* alphabetical sorting placed first (‘inverted order’). Optional and non-repeating: see Group P.7 introductory
* text for valid options.
*
* Jonix-Comment: this field is optional
*/
public PersonNameInverted personNameInverted() {
_initialize();
return personNameInverted;
}
private TitlesBeforeNames titlesBeforeNames = TitlesBeforeNames.EMPTY;
/**
*
* The first part of a structured name of a person who contributed to the creation of the product: qualifications
* and/or titles preceding a person’s names, eg ‘Professor’ or ‘HRH Prince’ or ‘Saint’. Optional and
* non-repeating: see Group P.7 introductory text for valid options.
*
* Jonix-Comment: this field is optional
*/
public TitlesBeforeNames titlesBeforeNames() {
_initialize();
return titlesBeforeNames;
}
private NamesBeforeKey namesBeforeKey = NamesBeforeKey.EMPTY;
/**
*
* The second part of a structured name of a person who contributed to the creation of the product: name(s) and/or
* initial(s) preceding a person’s key name(s), eg James J. Optional and non-repeating.
*
* Jonix-Comment: this field is optional
*/
public NamesBeforeKey namesBeforeKey() {
_initialize();
return namesBeforeKey;
}
private PrefixToKey prefixToKey = PrefixToKey.EMPTY;
/**
*
* The third part of a structured name of a person who contributed to the creation of the product: a prefix which
* precedes the key name(s) but which is not to be treated as part of the key name, eg ‘van’ in Ludwig van
* Beethoven. This element may also be used for titles that appear after given names and before key names, eg
* ‘Lord’ in Alfred, Lord Tennyson. Optional and non-repeating.
*
* Jonix-Comment: this field is optional
*/
public PrefixToKey prefixToKey() {
_initialize();
return prefixToKey;
}
private NamesAfterKey namesAfterKey = NamesAfterKey.EMPTY;
/**
*
* The fifth part of a structured name of a person who contributed to the creation of the product: name suffix, or
* name(s) following a person’s key name(s), eg ‘Ibrahim’ (in Anwar Ibrahim). Optional and non-repeating.
*
* Jonix-Comment: this field is optional
*/
public NamesAfterKey namesAfterKey() {
_initialize();
return namesAfterKey;
}
private SuffixToKey suffixToKey = SuffixToKey.EMPTY;
/**
*
* The sixth part of a structured name of a person who contributed to the creation of the product: a suffix
* following a person’s key name(s), eg ‘Jr’ or ‘III’. Optional and non-repeating.
*
* Jonix-Comment: this field is optional
*/
public SuffixToKey suffixToKey() {
_initialize();
return suffixToKey;
}
private LettersAfterNames lettersAfterNames = LettersAfterNames.EMPTY;
/**
*
* The seventh part of a structured name of a person who contributed to the creation of the product: qualifications
* and honors following a person’s names, eg ‘CBE FRS’. Optional and non-repeating.
*
* Jonix-Comment: this field is optional
*/
public LettersAfterNames lettersAfterNames() {
_initialize();
return lettersAfterNames;
}
private TitlesAfterNames titlesAfterNames = TitlesAfterNames.EMPTY;
/**
*
* The eighth part of a structured name of a person who contributed to the creation of the product: titles following
* a person’s names, eg ‘Duke of Edinburgh’. Optional and non-repeating.
*
* Jonix-Comment: this field is optional
*/
public TitlesAfterNames titlesAfterNames() {
_initialize();
return titlesAfterNames;
}
private Gender gender = Gender.EMPTY;
/**
*
* An optional ONIX code specifying the gender of a personal contributor. Not repeatable. Note that this indicates
* the gender of the contributor’s public identity (which may be pseudonymous) based on designations used in ISO
* 5218, rather than the gender identity, biological sex or sexuality of a natural person.
*
* Jonix-Comment: this field is optional
*/
public Gender gender() {
_initialize();
return gender;
}
private CorporateNameInverted corporateNameInverted = CorporateNameInverted.EMPTY;
/**
*
* The name of a corporate body which contributed to the creation of the product, presented in inverted order, with
* the element used for alphabetical sorting placed first. Optional and non-repeating: see Group P.7
* introductory text for valid options.
*
* Jonix-Comment: this field is optional
*/
public CorporateNameInverted corporateNameInverted() {
_initialize();
return corporateNameInverted;
}
private ListOfOnixComposite alternativeNames = JPU.emptyListOfOnixComposite(AlternativeName.class);
/**
*
* A repeatable group of data elements which together represent an alternative name of a person or organization that
* is part of the subject of a product, and specify its type. The <AlternativeName> composite is optional.
*
* Jonix-Comment: this list may be empty
*/
public ListOfOnixComposite alternativeNames() {
_initialize();
return alternativeNames;
}
private ListOfOnixDataCompositeWithKey subjectDates =
JPU.emptyListOfOnixDataCompositeWithKey(SubjectDate.class);
/**
*
* A group of data elements which together specify a date associated with the person or organization identified in
* an occurrence of the <NameAsSubject> composite, eg birth or death. Optional, and repeatable to
* provide multiple dates with their various roles.
*
* Jonix-Comment: this list may be empty
*/
public ListOfOnixDataCompositeWithKey subjectDates() {
_initialize();
return subjectDates;
}
private ListOfOnixDataComposite professionalAffiliations =
JPU.emptyListOfOnixDataComposite(ProfessionalAffiliation.class);
/**
*
* An optional group of data elements which together identify a subject’s professional position and/or affiliation,
* repeatable to allow multiple positions and affiliations to be specified.
*
* Jonix-Comment: this list may be empty
*/
public ListOfOnixDataComposite professionalAffiliations() {
_initialize();
return professionalAffiliations;
}
}